Although we have already removed most of the image background, forehead, and hair when we did the geometrical transformation, we can apply an elliptical mask to remove some of the corner regions, such as the neck, which might be in shadow from the face, particularly if the face is not looking perfectly straight toward the camera. To create the mask, we will draw a black-filled ellipse onto a white image. One ellipse to perform this has a horizontal radius of 0.5 (that is, it covers the face width perfectly), a vertical radius of 0.8 (as faces are usually taller than they are wide), and centered at the coordinates 0.5, 0.4, as shown in the following screenshot, where the elliptical mask has removed some unwanted corners from the face:
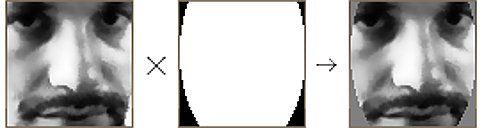
We can apply the mask when calling the cv::setTo() function, which would normally set a whole image to a certain pixel value, but as we will give a mask image, it will only set some parts to the given pixel value. We will fill the image in with gray so that it should have less contrast to the rest of the face, as follows:
// Draw a black-filled ellipse in the middle of the image.
// First we initialize the mask image to white (255).
Mat mask = Mat(warped.size(), CV_8UC1, Scalar(255));
double dw = DESIRED_FACE_WIDTH;
double dh = DESIRED_FACE_HEIGHT;
Point faceCenter = Point( cvRound(dw * 0.5),
cvRound(dh * 0.4) );
Size size = Size( cvRound(dw * 0.5), cvRound(dh * 0.8) );
ellipse(mask, faceCenter, size, 0, 0, 360, Scalar(0),
CV_FILLED);
// Apply the elliptical mask on the face, to remove corners.
// Sets corners to gray, without touching the inner face.
filtered.setTo(Scalar(128), mask);
The following enlarged screenshot shows a sample result from all the face preprocessing stages. Notice it is much more consistent for face recognition at different brightness, face rotations, angles from camera, backgrounds, positions of lights, and so on. This preprocessed face will be used as input to the face recognition stages, both when collecting faces for training and when trying to recognize input faces:
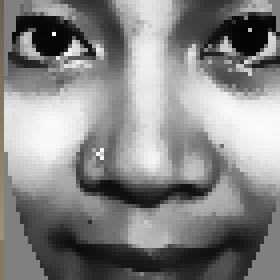