When a user logs in to the system, a token is generated based on the payload (that is, the user information and secret key). The generated token is stored locally. For all future requests, this token is added to the request and the application will validate the token before responding to the request:
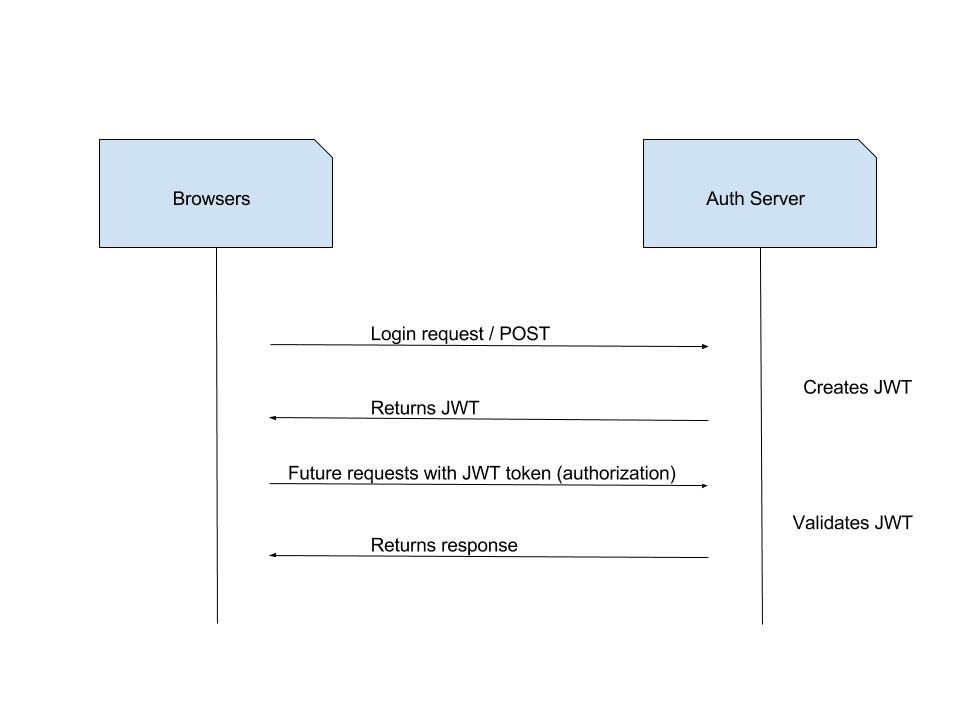
The token will be in this format:
Authorization: Bearer <token>
In JHipster, we use JJWT (Java-based JSON Web Tokens) from Okta. This is a simplified builder pattern-based library used to generate and sign the token as a producer, and parse and validate the token as a consumer.
Creating the token:
public class TokenProvider {
...
public String createToken(Authentication authentication, Boolean rememberMe) {
...
return Jwts.builder()
.setSubject(authentication.getName())
.claim(AUTHORITIES_KEY, authorities)
.signWith(SignatureAlgorithm.HS512, secretKey)
.setExpiration(validity)
.compact();
}
}
Validating the token:
public boolean validateToken(String authToken) {
try {
Jwts.parser().setSigningKey(secretKey).parseClaimsJws(authToken);
return true;
} catch (SignatureException e) {
log.info("Invalid JWT signature.");
log.trace("Invalid JWT signature trace: {}", e);
} catch (MalformedJwtException e) {
log.info("Invalid JWT token.");
log.trace("Invalid JWT token trace: {}", e);
} catch (ExpiredJwtException e) {
log.info("Expired JWT token.");
log.trace("Expired JWT token trace: {}", e);
} catch (UnsupportedJwtException e) {
log.info("Unsupported JWT token.");
log.trace("Unsupported JWT token trace: {}", e);
} catch (IllegalArgumentException e) {
log.info("JWT token compact of handler are invalid.");
log.trace("JWT token compact of handler are invalid trace: {}", e);
}
return false;
}
So far, we have created a gateway application, which will serve as a single point of entry for our application and services. Now, we will generate a microservice application using JHipster.
Microservice applications are the services. We will construct two sample services in this book and discuss the features that JHipster offers, one with the SQL database (MySQL) and the other with the NoSQL database (MongoDB). These services are individual and loosely coupled.
With JHipster, you can build microservice applications serving as REST endpoints.