To get started, create a new file (File | New | File...) and select a Cocoa Touch file. Name the file ContactTableViewCell and make sure it subclasses UITableViewCell, as shown in the following screenshot:
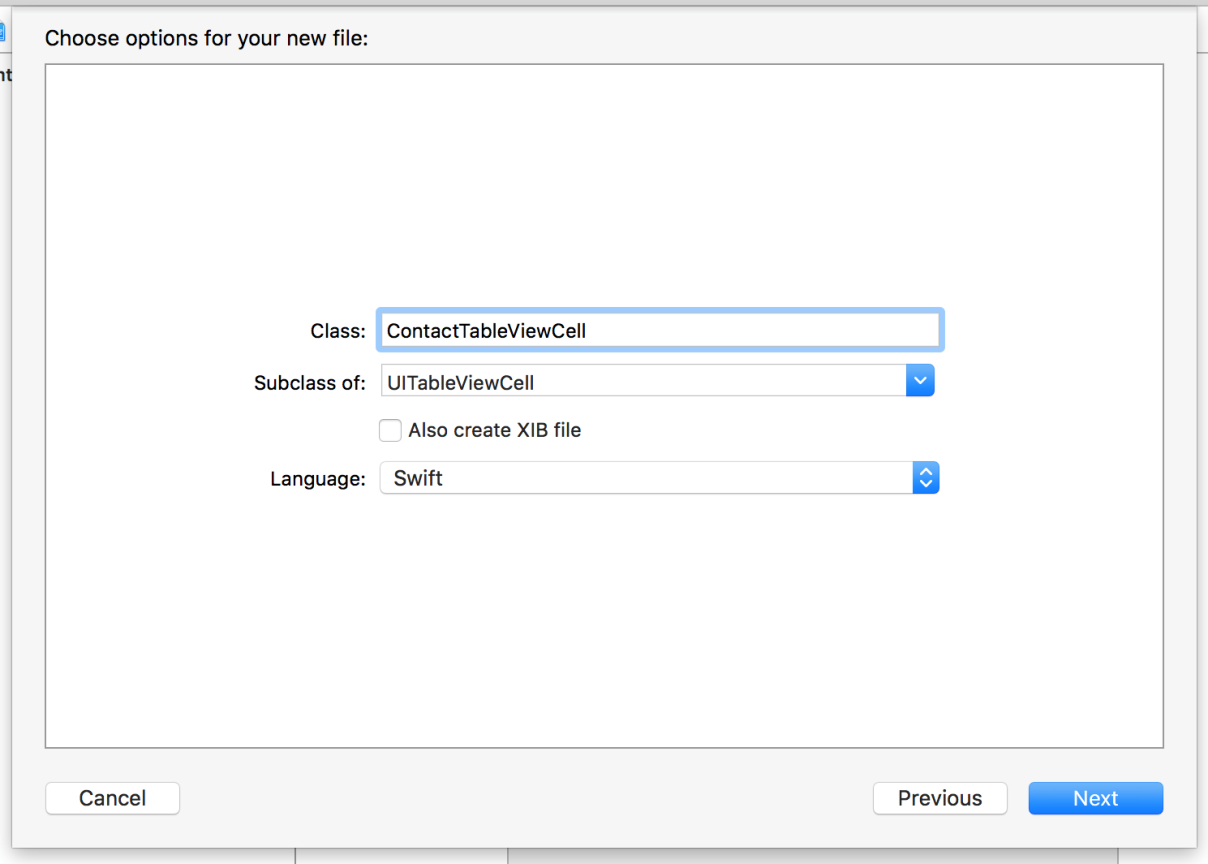
When you open the newly created file, you'll see two methods already added to the template for ContactTableViewCell.swift. These methods are awakeFromNib and setSelected(_:animated:). The awakeFromNib method is called the very first time this cell is created; you can use this method to do some initial setup that's required to be executed only once for your cell.
The other method is used to customize your cell when a user taps on it. You could, for instance, change the background color or text color or even perform an animation. For now, you can delete both of these methods and replace the contents of this class with the following code:
@IBOutlet var nameLabel: UILabel!
@IBOutlet var contactImage: UIImageView!
The preceding code should be the entire body of the ContactTableViewCell class. It creates two @IBOutlets that will allow you to connect your prototype cell with them so that you can use them in your code to display the contact's name and image later.
In the Main.storyboard file, you should select your cell, and in the Identity Inspector on the right, set its class to ContactTableViewCell (as shown in the following screenshot). This will make sure that the Interface Builder knows which class it should use for this cell, and it will make the @IBOutlets visible to Interface Builder.
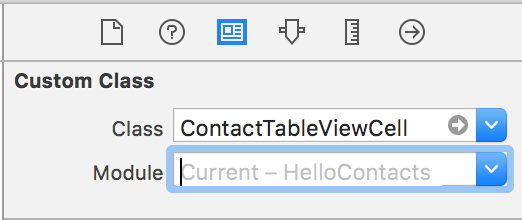
Now that the prototype cell has the correct class, select the label that will hold the contact's name in your prototype cell and click on Connections Inspector. Then, drag a new referencing outlet from the Connections Inspector to your cell and select nameLabel to connect the UILabel in the prototype cell to the @IBOutlet that was added to the UITableViewCell subclass earlier (refer to the following screenshot). Perform the same steps for the UIImageView and select the contactImage option instead of nameLabel.
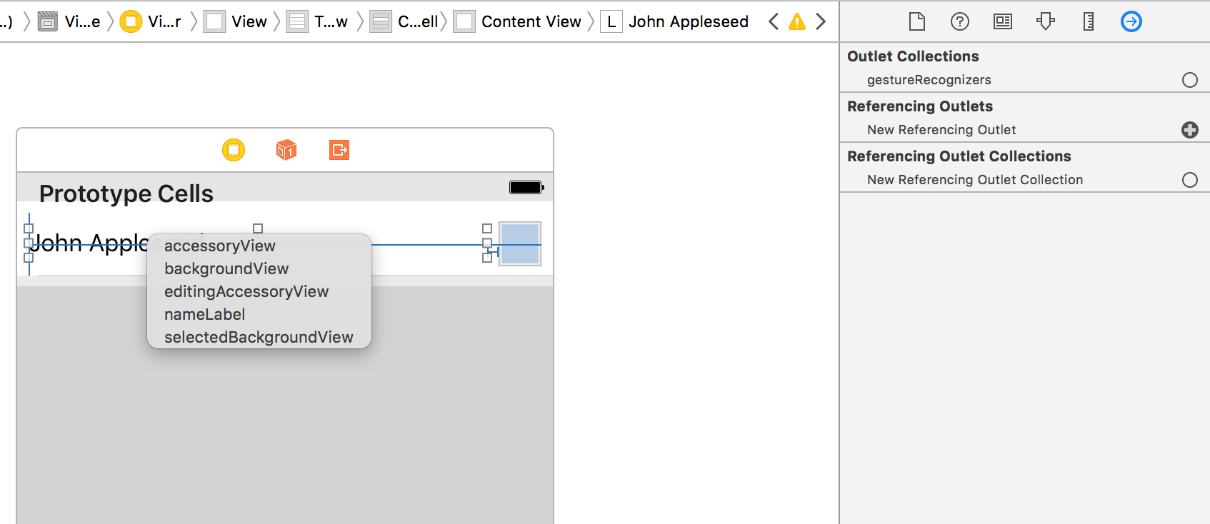
The last thing you will need to do is to provide a reuse identifier for the cell. The reuse identifier is the identifier that is used to inform the UITableView about which cell it should create or reuse when needed. Cell reuse is an optimization feature that will be covered more in depth later in this chapter.
To set the reuse identifier you must open the Attributes Inspector after selecting your cell. In the Attributes Inspector, you will find an input field for the Identifier. Set this input field to ContactTableViewCell.
Now that the custom UITableViewCell is all set up, you need to make sure that the UITableView is aware of the fact that our ViewController class will provide it with the required data and cells by setting the delegate and data source properties.