Anchor characters are used to match the beginning or the end of a line. There are two anchor characters: the caret (^) and the dollar sign ($).
The caret character is used to match the beginning of a line:
$ echo "Welcome to shell scripting" | awk '/^Welcome/{print $0}'
$ echo "SHELL scripting" | awk '/^Welcome/{print $0}'
$ echo "Welcome to shell scripting" | sed -n '/^Welcome/p'

So, the caret character is used to check whether the specified text is at the beginning of the line.
If you want to search for the caret as a character, you should escape it with a backslash if you use AWK.
However, if you use sed, you don't need to escape it:
$ echo "Welcome ^ is a test" | awk '/\^/{print $0}'
$ echo "Welcome ^ to shell scripting" | sed -n '/^/p'

To match the end of the text, you can use the dollar sign character ($):
$ echo "Welcome to shell scripting" | awk '/scripting$/{print $0}'
$ echo "Welcome to shell scripting" | sed -n '/scripting$/p'

You can use both characters (^) and ($) in the same pattern to specify text.
You can use these characters to do something useful, such as search for empty lines and trim them:
$ awk '!/^$/{print $0}' myfile
The exclamation mark (!) is called the negation character, which negates what's after it.
The pattern searches for ^$ where the caret (^) refers to the beginning of a line and the dollar sign ($) refers to the end of a line, which means search for lines that have nothing between the beginning and the end which means empty lines. Then we negate that with the exclamation mark (!) to get the other lines that are not empty.
Let's apply it to the following file:
Lorem Ipsum is simply dummy text .
Lorem Ipsum has been the industry's standard dummy.
It has survived not only five centuries
It is a long established fact that a reader will be distracted.
Now, let's see the magic:
$ awk '!/^$/{print $0}' myfile
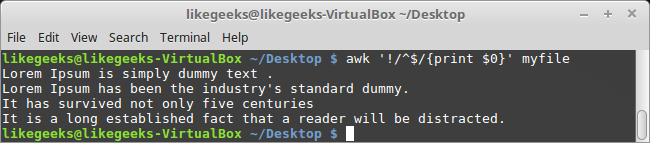
The lines are printed without the empty lines.