We have remained faithful to the print command so far, as we have been limited in what we require from the output. If we want to print out, say, the username, UID, and default shell, we need to start formatting the output just a little. In this case, we can organize the output in well-shaped columns. Without formatting, the command we use will look similar to the following example, where we use commas to separate the field that we want to print:
$ awk ' BEGIN { FS=":" } { print $1,$3,$7 } ' /etc/passwd
We use the BEGIN block here, as we can make use of it to print column headers later.
To understand the problem a little better, take a look at the following screenshot, which illustrates uneven column widths:

The issue that we have in the output is that the columns do not align, as the username is an inconsistent length. To improve on this, we can use the printf function where we can specify the column width. The syntax for the awk statements will be similar to the following example:
$ awk ' BEGIN { FS=":" } > { printf "%10s %4d %17s\n",$1,$3,$7 } ' /etc/passwd
The printf formatting is included within double quotes. We also need to include the newline with theĀ \n. The printf function does not add a newline automatically, whereas the print function does. We print the three fields; the first accepts string values and is set to 10 characters wide. The middle field accepts up to 4 numbers and we finish with the default shell field where we allow up to 17 string characters.
The following screenshot shows how the output can be improved:
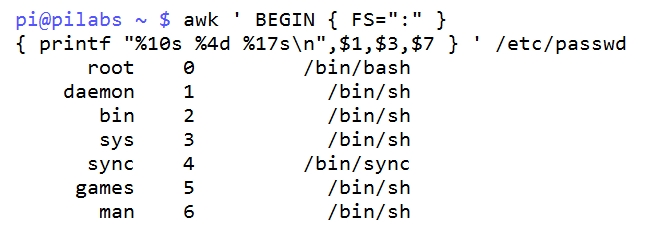
We can further enhance this by adding header information. Although the code starts to look untidy at this stage, we will later see how we can resolve this with AWK control files. The following example shows the header information being added to the Begin block. The semicolon is used to separate the two statements in the BEGIN block:
$ awk 'BEGIN {FS=":" ;printf "%10s %4s %17s\n","Name","UID","Shell" } > { printf "%10s %4d %17s\n",$1,$3,$7 } ' /etc/passwd
In the following screenshot, we can see how this improves the output even further:
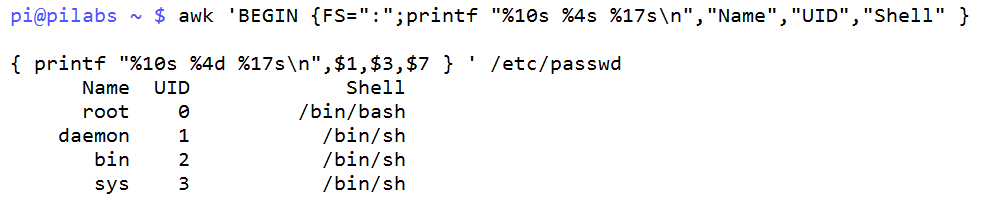
In the previous chapter, we saw how we can augment the output with the use of colors in the shell. We may also use color from within AWK by adding our own functions. In the next code example, you will see that AWK allows us to define our own functions to facilitate more complex operations and isolate the code. We will now modify the previous code to include green output in the header:
$ awk 'function green(s) { > printf "\033[1;32m" s "\033[0m\n" > } > BEGIN {FS=":";
green(" Name: UID: Shell:") } > { printf "%10s %4d %17s\n",$1,$3,$7 } ' /etc/passwd
Creating the function within awk allows color to be added where we require, in this case, green text. It is easy to create functions to define other colors. The code and output are included in the following screenshot:
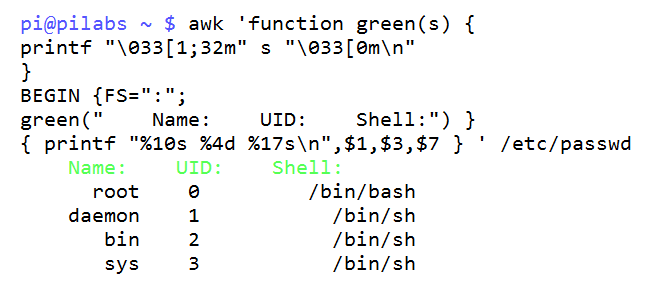