As with any normal view, our custom view will be subject to the layout manager's margins and to the view's padding. We should not worry that much about the margin values, as the layout manager will directly process them and will transparently modify the size available for our custom view. The values we've to take into consideration are the padding values. As we can see in the following image, the margin is the space the layout manager is adding before and after our custom view and the padding is the internal space between the view boundaries and the content itself:
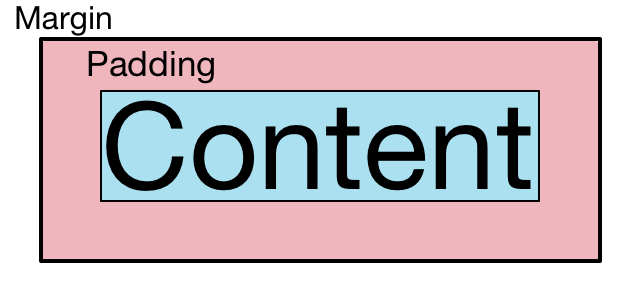
Our view has to manage this padding appropriately. To do so, we can directly use the different getPadding methods from canvas such as getPaddingTop(), getPaddingBottom(), getPaddingStart(), and so on. Using the padding values, we should adjust the rendering area accordingly on our onDraw() method:
protected void onDraw(Canvas canvas) {
int startPadding = getPaddingStart();
int topPadding = getPaddingTop();
int width = canvas.getWidth() - startPadding - getPaddingEnd();
int height = canvas.getHeight() - topPadding - getPaddingBottom();
}
In this code, we're storing the left and topmost points of our Canvas which are the start and top padding values respectively. We have to be careful with this sentence as the start padding might not be the left padding. If we check the documentation we'll find there are both getPaddingStart(), getPaddingEnd(), getPaddingLeft(), and getPaddingRight(). The start padding, for example, can be the right padding if our device is configured in Right-To-Left (RTL) mode. We have to be careful with these details if we'd like to support both LTR and RTL devices. In this specific example, we'll build it with RTL support by detecting the layout direction using the getLayoutDirection() method available on view. But first, let's focus on a very simple implementation.