In the following steps, you will learn how to run instrumentation tests:
- Let's create a simple app, which will just have the Hello World! text and a button:
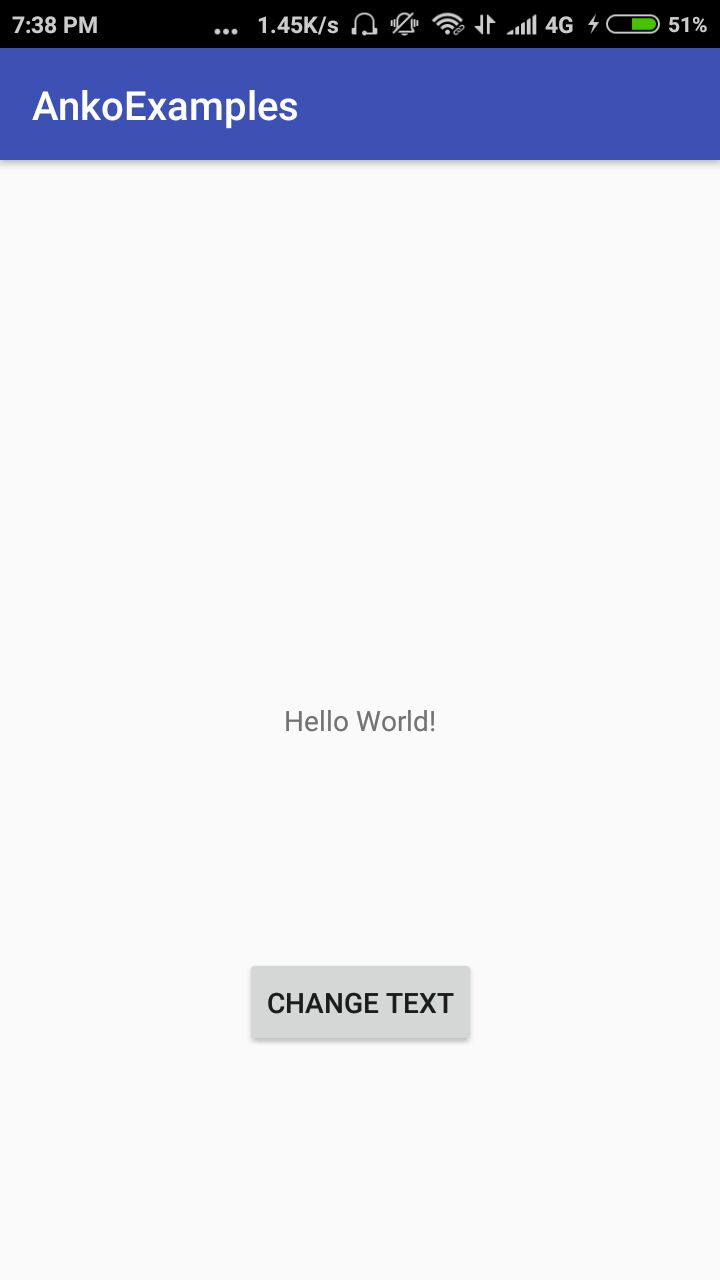
- On clicking the button, the text will change to Goodbye World!:
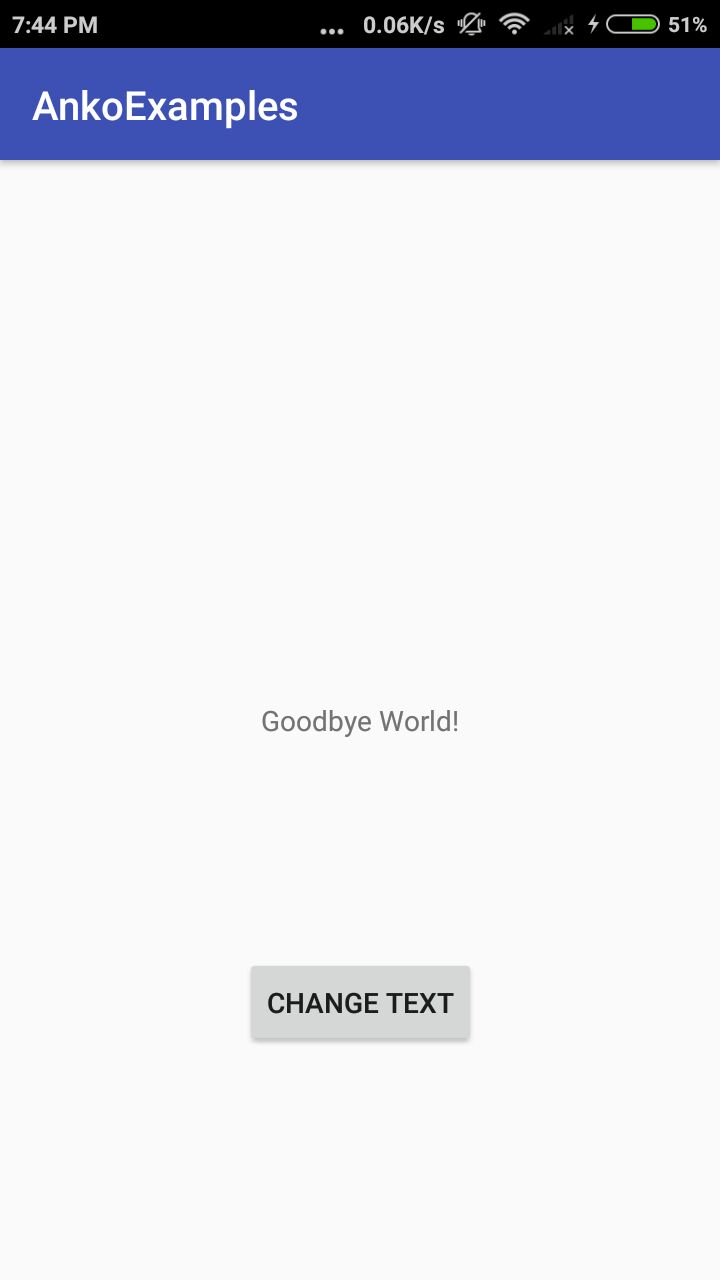
- Now, let's write a test to verify this behavior.
In this recipe, we will learn how to run the espresso test; in the next recipe, we will learn how to write an espresso test, so just bear with me until I explain how to write an espresso test in the later recipe because it is complicated and needs an entire recipe to do justice to it.
Here's an espresso test:
class MainActivityTest {
@Rule
@JvmField var activityRule: ActivityTestRule<MainActivity> = ActivityTestRule(MainActivity::class.java)
@Test
fun testButtonBehaviour() {
onView(withText("Hello World!"))
.check(matches(isDisplayed()))
onView(withId(button)).perform(click())
onView(withText("Goodbye World!"))
.check(matches(isDisplayed()))
}
}
In the first line of the testButtonBehaviour method, we are checking whether Hello World! is appearing on screen. Then, we are performing a click operation on the button and finally checking whether Goodbye World! is appearing on the screen.
- To run the preceding test, just right-click on the test class and select Run MainActivityTest:

- Once you select that option, you'll be shown a dialog box that will ask which device you want to run the test on. You can either choose a real device or an emulator.
- After that, you can see your tests running on your device (you will see the steps written in the code being performed on the device).