In order to create the previously mentioned service, follow these steps in Azure portal:
- Click the Create a resource button found on the upper left-hand corner of the Azure portal, and then select Compute | Function App.
- Create the Function App, as per the settings in the following screenshot (some settings might vary as per your account and existing resources):
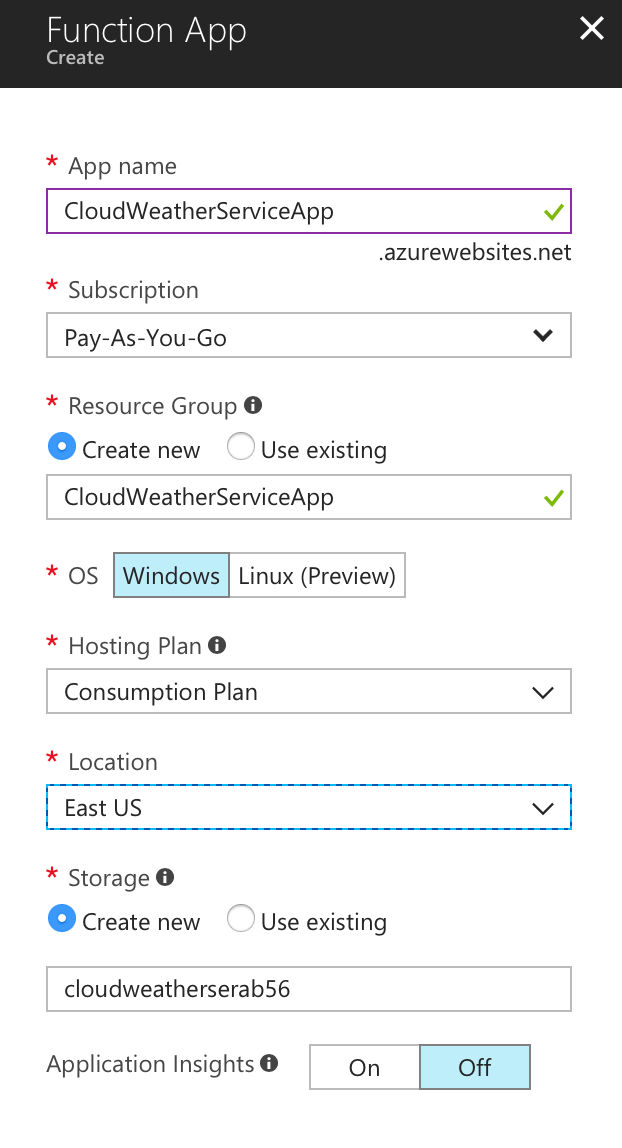
- Once the Functions App is created, it's time to create our core business login in the form of an Azure function. So, for that, expand your new function app, then click the + button next to Functions, and create a new HTTP trigger function as follows:
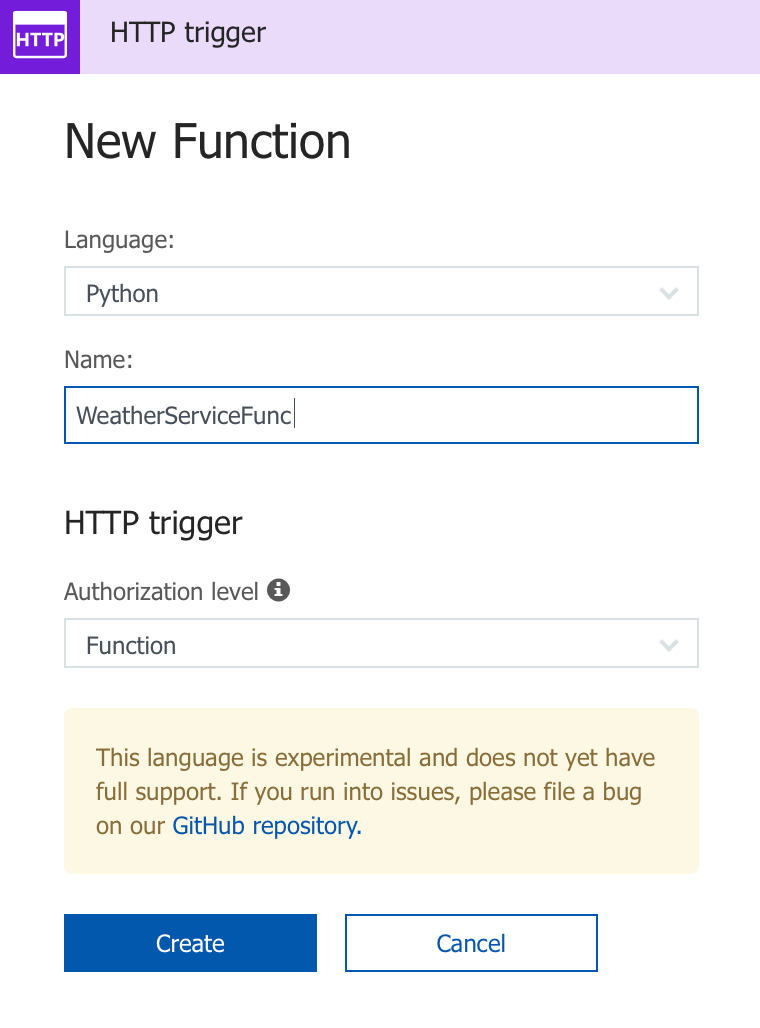
- Once the function is created, edit the inline code and use the following Python-based logic:
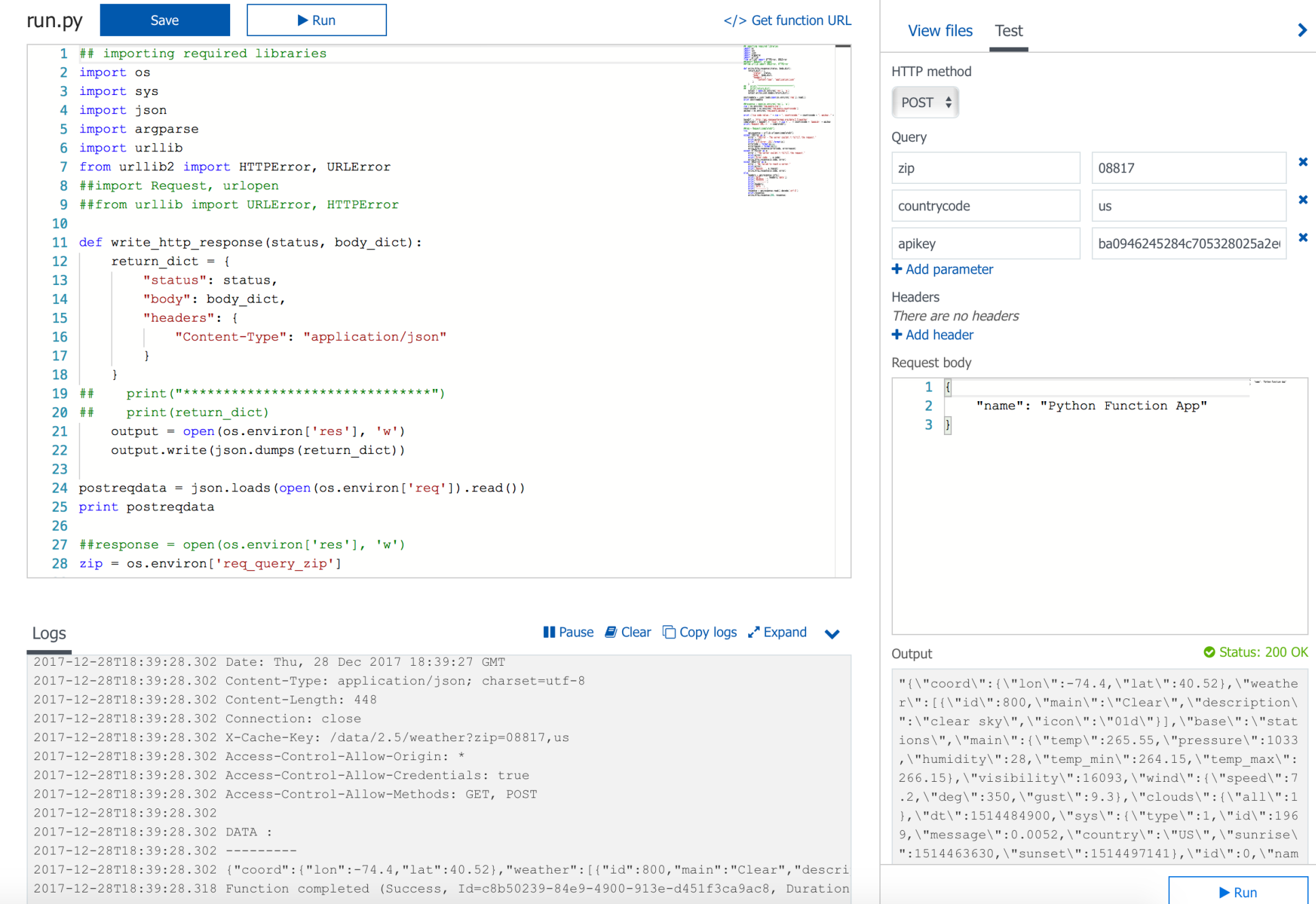
The Python code for the function
## importing required libraries
import os
import sys
import json
import argparse
import urllib
from urllib2 import HTTPError, URLError
## response construction and return
def write_http_response(status, body_dict):
return_dict = {
"status": status,
"body": body_dict,
"headers": {
"Content-Type": "application/json"
}
}
output = open(os.environ['res'], 'w')
output.write(json.dumps(return_dict))
## extract input parameter values
zip = os.environ['req_query_zip']
countrycode = os.environ['req_query_countrycode']
apikey = os.environ['req_query_apikey']
print ("zip code value::" + zip + ", countrycode:" + countrycode + ",
apikey::" + apikey)
## construct full URL to invoke OpenWeatherMap service with proper inputs
baseUrl = 'http://api.openweathermap.org/data/2.5/weather'
completeUrl = baseUrl + '?zip=' + zip + ',' + countrycode + '&appid=' + apikey
print('Request URL--> ' + completeUrl)
## Invoke OpenWeatherMap API and parse response with proper exception handling
try:
apiresponse = urllib.urlopen(completeUrl)
except IOError as e:
error = "IOError - The server couldn't fulfill the request."
print(error)
print("I/O error: {0}".format(e))
errorcode = format(e[1])
errorreason = format(e[2])
write_http_response(errorcode, errorreason)
except HTTPError as e:
error = "The server couldn't fulfill the request."
print(error)
print('Error code: ', e.code)
write_http_response(e.code, error)
except URLError as e:
error = "We failed to reach a server."
print(error)
print('Reason: ', e.reason)
write_http_response(e.code, error)
else:
headers = apiresponse.info()
print('DATE :', headers['date'])
print('HEADERS :')
print('---------')
print(headers)
print('DATA :')
print('---------')
response = apiresponse.read().decode('utf-8')
print(response)
write_http_response(200, response)
- Save the function, add sample query parameters (as shown in the preceding screenshot), and test your function. To look at all the function execution logs and results, you can go to the Monitor option under your function name and see the details as follows:
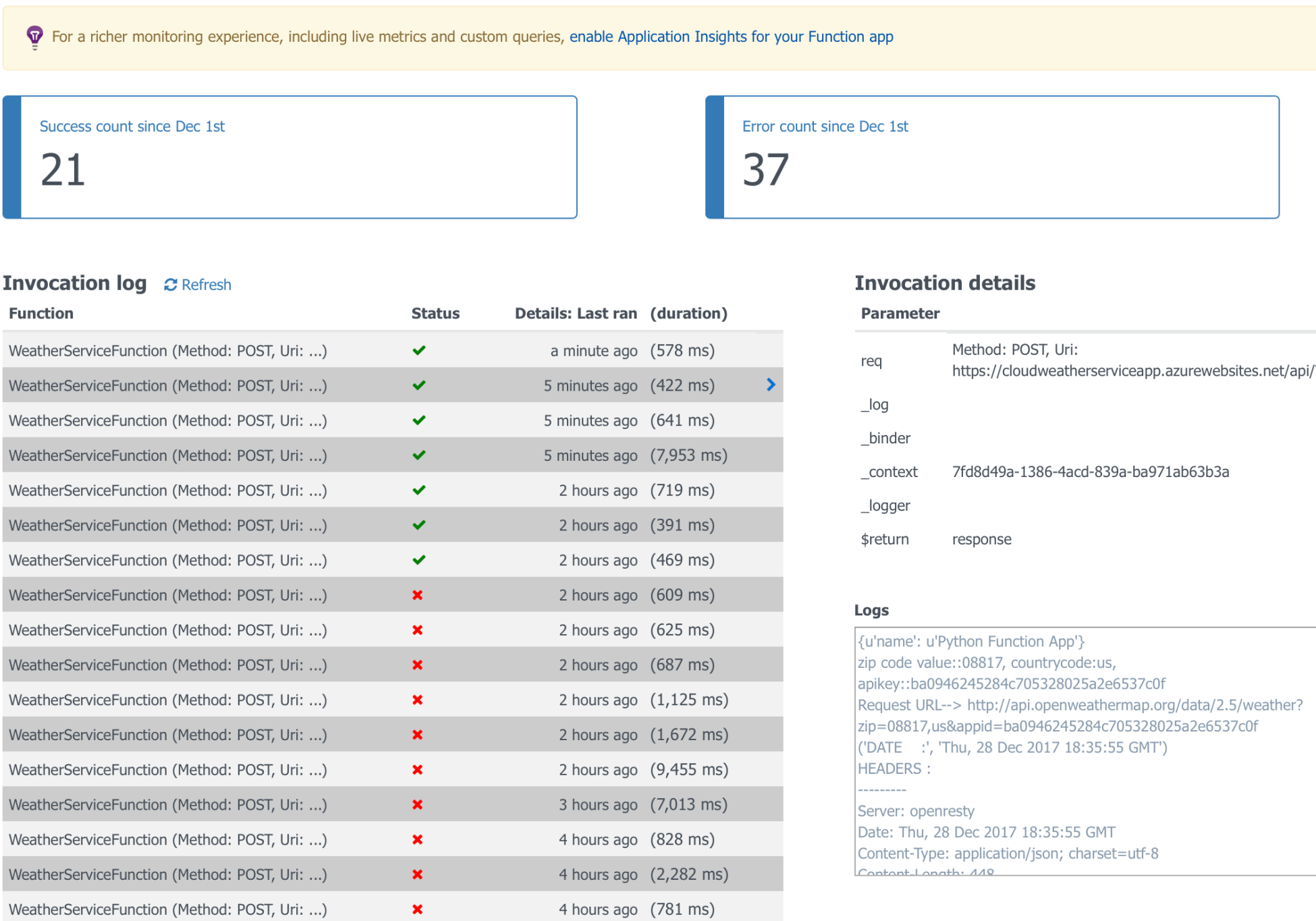
- Once you have unit tested the Azure Function from the portal, go to the Integrate option under your function and ensure that the HTTP GET method is enabled, apart from the other settings, as follows:
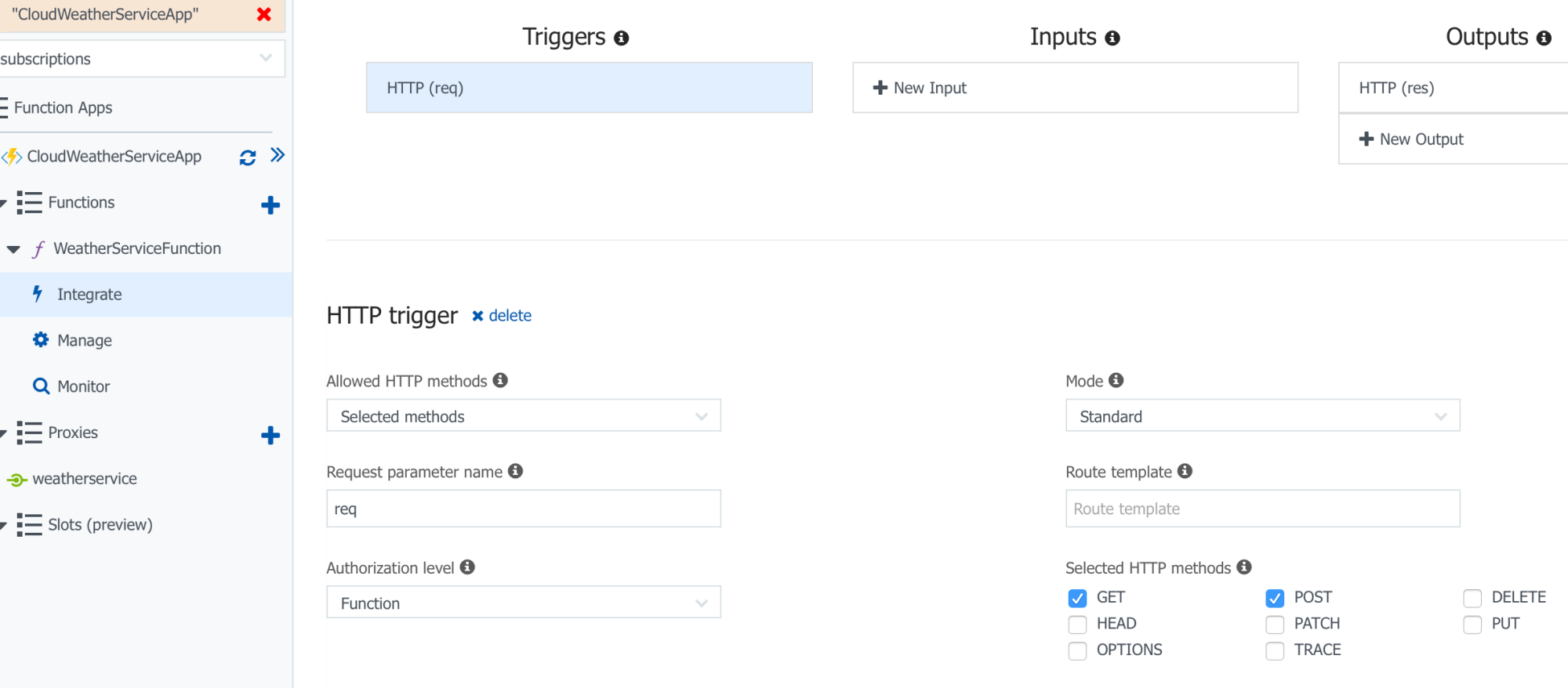
- Now, it's time to create an external facing API interface using proxies. To do that, you must first retrieve your Azure function URL from the top-right corner of your function definition, as follows:

Retrieving Azure function
- To expose this Azure function using an API, create a new proxy by pressing the + sign against the Proxies option. Ensure that it has the settings, like in the following screenshot. In the backend URL field, put the URL of your Azure function that you copied in the previous screenshot:

With this, your serverless microservice is now ready and you can test it using a web browser or CLI, which is detailed in the following subsection.