Our handle_cmd() method does nothing, for now, so let's update it. First of all, we'll need a method to send a response to a client:
impl Client { #[async] fn send(mut self, answer: Answer) -> Result<Self> { self.writer = await!(self.writer.send(answer))?; Ok(self) } }
This simply calls the send() method of the writer. Since it consumes it, we reassign the result to the attribute.
Now, we'll handle the USER FTP command:
#[async] fn handle_cmd(mut self, cmd: Command) -> Result<Self> { println!("Received command: {:?}", cmd); match cmd { Command::User(content) => { if content.is_empty() { self = await!
(self.send(Answer::new(ResultCode::InvalidParameterOrArgument, "Invalid
username")))?; } else { self = await!(self.send(Answer::new(ResultCode::UserLoggedIn,
&format!("Welcome {}!", content))))?; } } Command::Unknown(s) => self = await!(self.send(Answer::new(ResultCode::UnknownCommand, &format!("\"{}\": Not implemented", s))))? , _ => self = await!(self.send(Answer::new(ResultCode::CommandNotImplemented,
"Not implemented")))?, } Ok(self) }
Here, we pattern match to know which command was sent by the client. If it is not User, we send a response to say that the command is not implemented. If it is User, we check the content and if it is good, we send the welcome message. This is very similar to what we did in the previous chapter.
If we run the server again, we'll see the following:
>
Figure 9.2
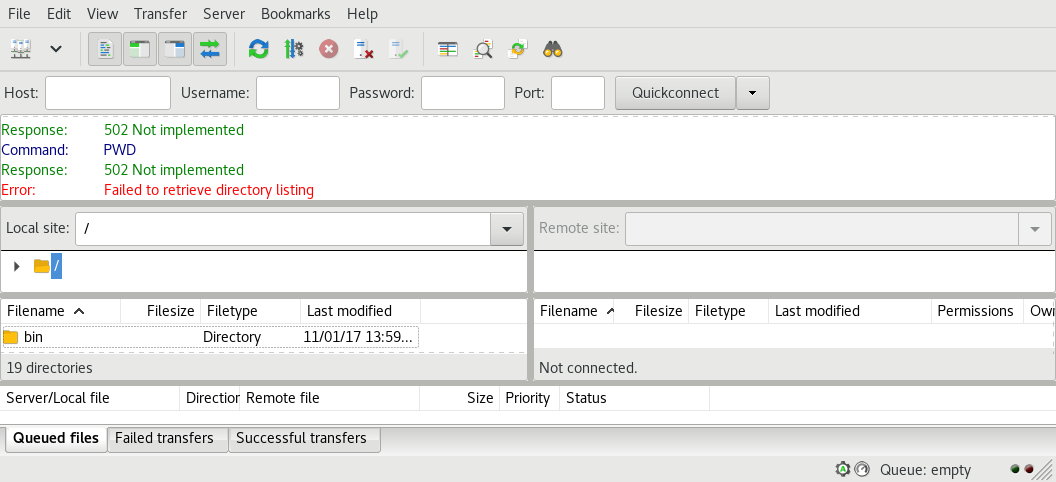