Lists in Python are one of the most versatile collection object types available. The other workhorses are dictionaries and tuples, but they are really more like variations of lists.
Python lists do the work of most of the data collection structures found in other languages, and since they are built in, you don't have to worry about manually creating them. Lists can be used for any type of object, from numbers and strings to other lists. They are accessed just like strings (since strings are just specialized lists), so they are simple to use. Lists are variable in length; that is, they grow and shrink automatically as they're used, and they can be changed in place; that is, a new list isn't created every time, unlike strings. In reality, Python lists are C arrays inside the Python interpreter and act just like an array of pointers.
The following screenshot shows the creation of a list and a few examples of how to use it:
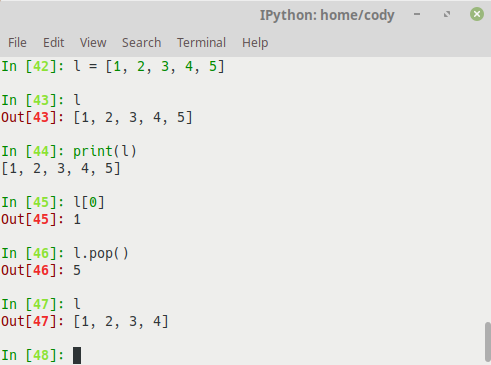
After the list is created in line 42, lines 43 and 44 show different ways of getting the values in a list; line 43 returns the list object while line 44 actually prints the items that are in the list. The difference is subtle, but will be more noticeable with more complicated code.
Line 45 returns the first item in the list, while line 46 pops out the last item. Returning an item doesn't modify the list, but popping an item does, as shown in line 47, where the list is visibly shorter.
The biggest thing to remember is that lists are series of objects written inside square brackets, separated by commas. Dictionaries and tuples will look similar except they have different types of brackets.