These steps cover writing and running your application:
- From your Terminal or console application, create a new directory called ~/projects/go-programming-cookbook/chapter9/tools, and navigate to this directory.
- Run the following command:
$ go mod init github.com/PacktPublishing/Go-Programming-Cookbook-Second-Edition/chapter9/tools
You should see a file called go.mod that contains the following:
module github.com/PacktPublishing/Go-Programming-Cookbook-Second-Edition/chapter9/tools
- Create a file called funcs.go with the following content:
package tools
import (
"fmt"
)
func example() error {
fmt.Println("in example")
return nil
}
var example2 = func() int {
fmt.Println("in example2")
return 10
}
- Create a file called structs.go with the following content:
package tools
import (
"errors"
"fmt"
)
type c struct {
Branch bool
}
func (c *c) example3() error {
fmt.Println("in example3")
if c.Branch {
fmt.Println("branching code!")
return errors.New("bad branch")
}
return nil
}
- Create a file called funcs_test.go with the following content:
package tools
import (
"testing"
. "github.com/smartystreets/goconvey/convey"
)
func Test_example(t *testing.T) {
tests := []struct {
name string
}{
{"base-case"},
}
for _, tt := range tests {
Convey(tt.name, t, func() {
res := example()
So(res, ShouldBeNil)
})
}
}
func Test_example2(t *testing.T) {
tests := []struct {
name string
}{
{"base-case"},
}
for _, tt := range tests {
Convey(tt.name, t, func() {
res := example2()
So(res, ShouldBeGreaterThanOrEqualTo, 1)
})
}
}
- Create a file called structs_test.go with the following content:
package tools
import (
"testing"
. "github.com/smartystreets/goconvey/convey"
)
func Test_c_example3(t *testing.T) {
type fields struct {
Branch bool
}
tests := []struct {
name string
fields fields
wantErr bool
}{
{"no branch", fields{false}, false},
{"branch", fields{true}, true},
}
for _, tt := range tests {
Convey(tt.name, t, func() {
c := &c{
Branch: tt.fields.Branch,
}
So((c.example3() != nil), ShouldEqual, tt.wantErr)
})
}
}
- Run the gocov test | gocov report command, and you will see the following output:
$ gocov test | gocov report
ok github.com/PacktPublishing/Go-Programming-Cookbook-Second-
Edition/chapter9/tools 0.006s
coverage: 100.0% of statements
github.com/PacktPublishing/Go-Programming-Cookbook-Second-
Edition/chapter9/tools/struct.go
c.example3 100.00% (5/5)
github.com/PacktPublishing/Go-Programming-Cookbook-Second-
Edition/chapter9/tools/funcs.go example
100.00% (2/2)
github.com/PacktPublishing/Go-Programming-Cookbook-Second-
Edition/chapter9/tools/funcs.go @12:16
100.00% (2/2)
github.com/PacktPublishing/Go-Programming-Cookbook-Second-
Edition/chapter9/tools ----------
100.00% (9/9)
Total Coverage: 100.00% (9/9)
- Run the goconvey command, and it will open a browser that should look like this:
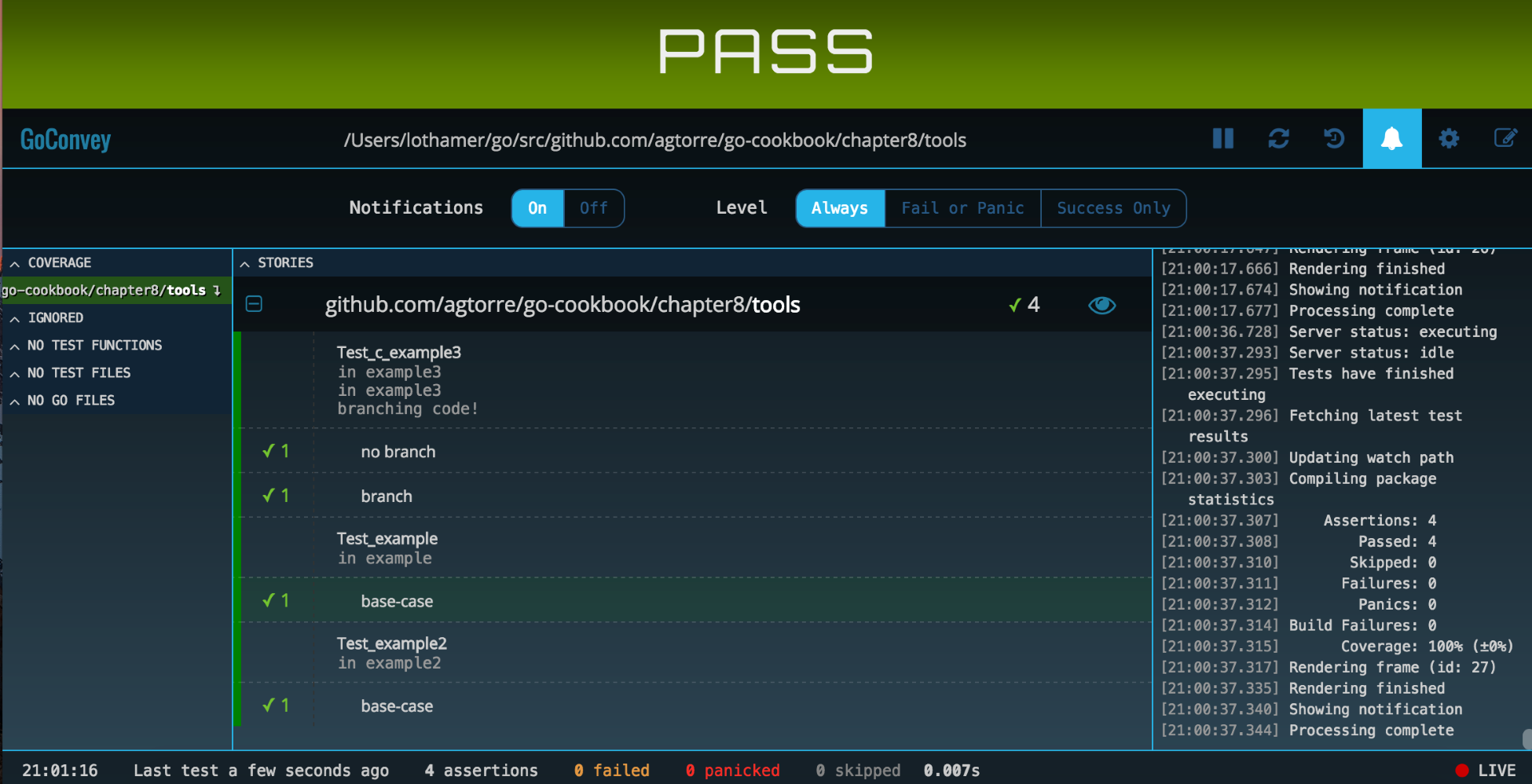
- Ensure that all the tests pass.
- The go.mod file may be updated and the go.sum file should now be present in the top-level recipe directory.