- Start by adding a new Windows forms project to your solution.
- Call the project winformSecure and click on the OK button.
- In the Toolbox, search for the TextBox control and add it to your form.
- Lastly, add a button control to your form. You can resize this form, however you like to look more like a login form:

- With the textbox control selected on the Windows forms, open up the Properties panel and click on the Events button (it looks like a lightning bolt). In the Key group, double-click on the KeyPress event to create the handler in the code behind:
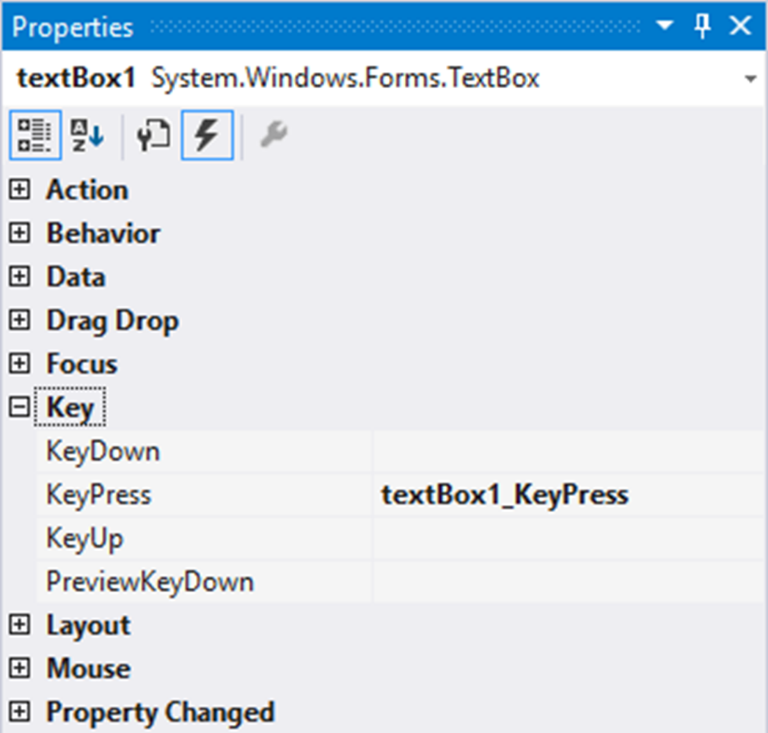
The code that is created for you is the KeyPress event handler for the textbox control. This will fire whenever a user presses a key on the keyboard:
private void textBox1_KeyPress(object sender, KeyPressEventArgs e)
{
}
- Back in the Properties panel, expand the Behavior group and change the value of UseSystemPasswordChar to True:
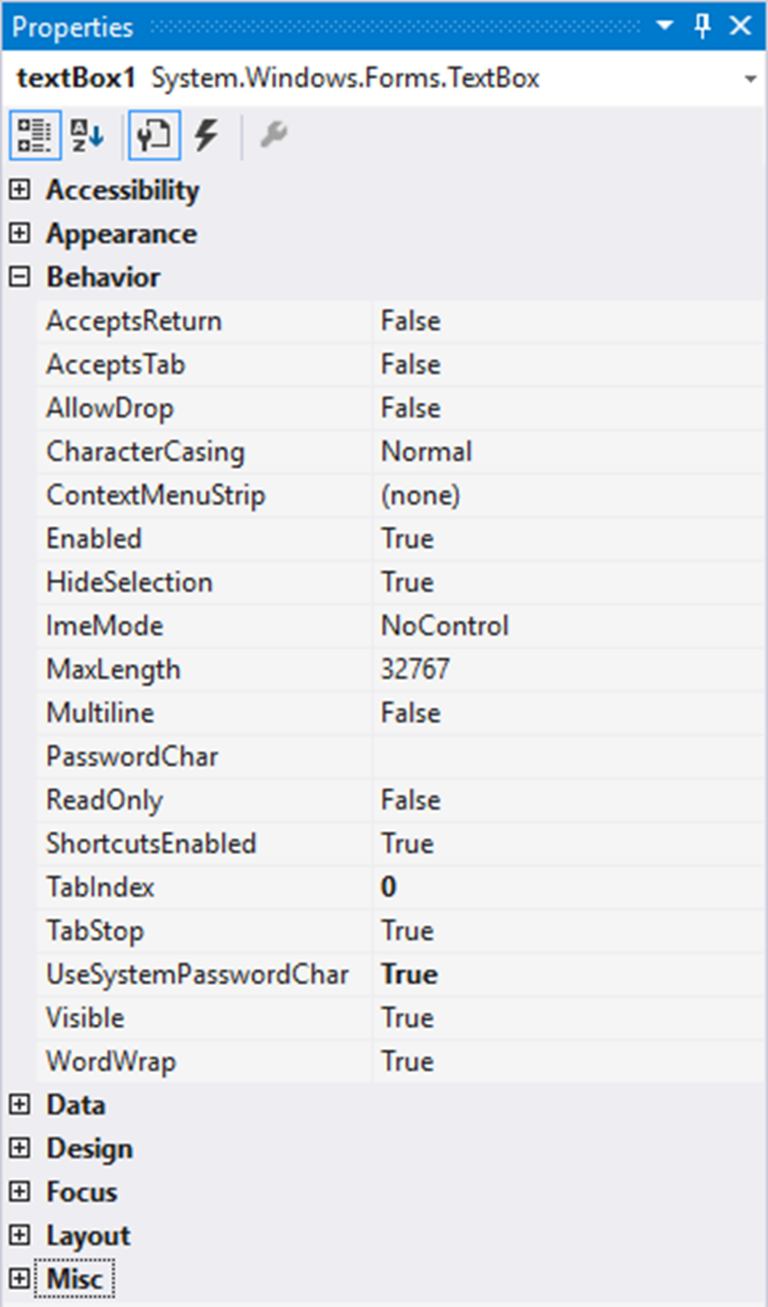
- In the code behind, add the followingusing statement:
using System.Runtime.InteropServices;
- Add the SecureString variable as a global variable to your Windows forms:
SecureString secure = new SecureString();
- Then, in the KeyPress event, append the KeyChar value to the SecureString variable every time the user presses a key. You might want to add code to ignore certain key presses, but this is beyond the scope of this recipe:
private void textBox1_KeyPress(object sender, KeyPressEventArgs e)
{
secure.AppendChar(e.KeyChar);
}
- Then, in the Login button's event handler, add the following code to read the value from the SecureString object. Here, we are working with unmanaged memory and unmanaged code:
private void btnLogin_Click(object sender, EventArgs e)
{
IntPtr unmanagedPtr = IntPtr.Zero;
try
{
if (secure == null)
throw new ArgumentNullException("Password not defined");
unmanagedPtr = Marshal.SecureStringToGlobalAllocUnicode(
secure);
MessageBox.Show($"SecureString password to validate is
{Marshal.PtrToStringUni(unmanagedPtr)}");
}
catch(Exception ex)
{
MessageBox.Show(ex.Message);
}
finally
{
Marshal.ZeroFreeGlobalAllocUnicode(unmanagedPtr);
secure.Dispose();
}
}
- Run your Windows forms application and type in a password:
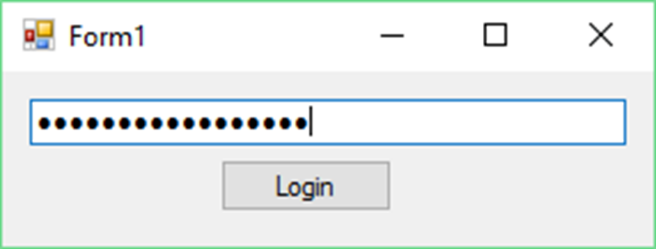
- Then click on the Login button. You will then see the password you typed in displayed in the message box:
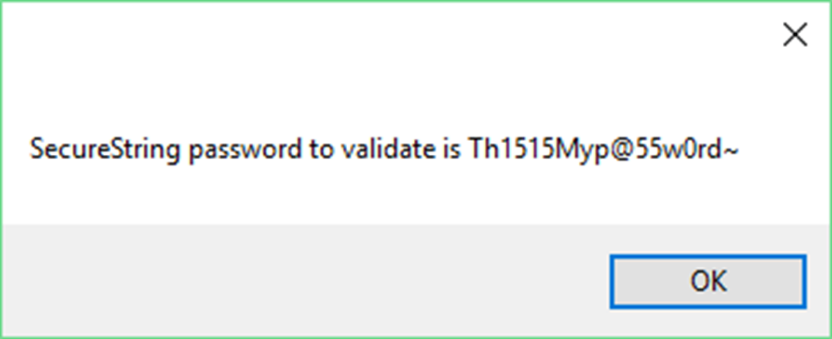