- Add the following using statements to the top of your Program.cs file:
using System.IO;
using System.IO.Compression;
- Create a method called ZipIt() and add the code to it to ZIP the Documents directory. The code is pretty straightforward to understand. I want to, however, highlight the use of the CreateFromDirectory() method. Notice that we have set the compression level to CompressionLevel.Optimal and set the includeBaseDirectory parameter to false:
private static void ZipIt(string path)
{
string sourceDirectory = $"{path}Documents";
if (Directory.Exists(sourceDirectory))
{
string archiveName = $"{path}DocumentsArchive.zip";
ZipFile.CreateFromDirectory(sourceDirectory, archiveName,
CompressionLevel.Optimal, false);
}
}
- Run your console application and look at the temp folder again. You will see the following ZIP file created:
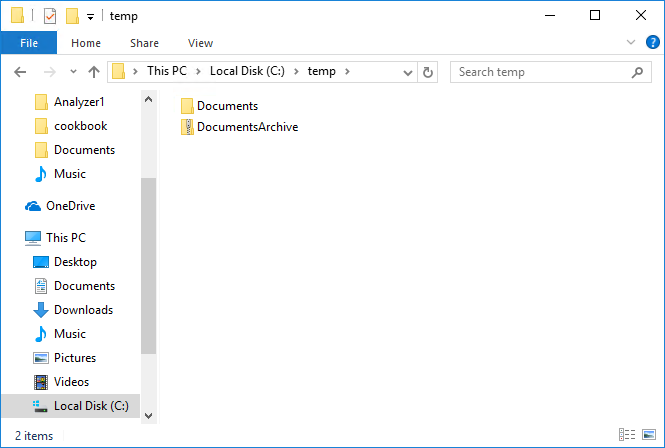
- Viewing the contents of the ZIP file will display the files contained in the Documents folder that we saw earlier:
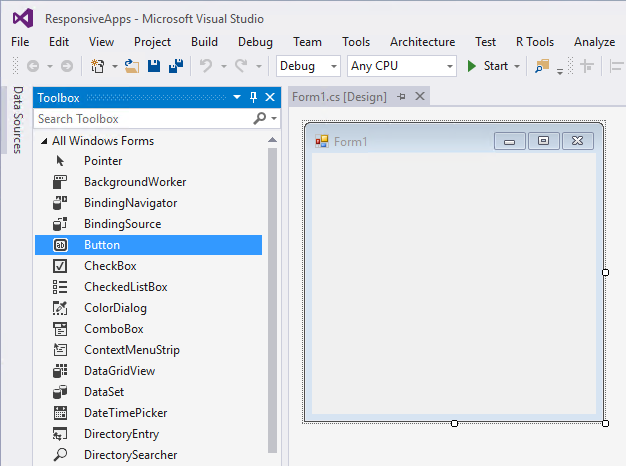
- Viewing the properties of the ZIP file, you will see that it has been compressed to 36 KB:

- Extracting a ZIP file is just as easy to do. Create a method called UnZipIt() and pass the path to the temp folder to it. Then, specify the directory to unzip the files to and set the variable called destinationDirectory. Call the ExtractToDirectory() method and pass it the archiveName and destinationDirectory variables as parameters:
private static void UnZipIt(string path)
{
string destinationDirectory = $"{path}DocumentsUnzipped";
if (Directory.Exists(path))
{
string archiveName = $"{path}DocumentsArchive.zip";
ZipFile.ExtractToDirectory(archiveName, destinationDirectory);
}
}
- Run your console application and view the output folder:

- Viewing the extracted files in the DocumentsUnzipped folder, you will see the original files we started off with:
