In the last chapter, we engaged in a comprehensive examination of cryptocurrency transactions. Transactions are the fundamental unit of computation in cryptocurrency networks. Without transactions, there is no blockchain. A block consists of several transactions as well as related data. For any block that contains transactions, we want to ascertain two properties: firstly, that these transactions have not been tampered with and, secondly, whether a particular transaction is present in the block.
You will recall from Chapter 8 that the block attribute prevblockhash is used to test whether the previous block has been tampered with. This hash is computed over the block header of the previous block. The transactions in the previous block are not in this block header. However, the block header contains the merkle root of these transactions. This root can be used to verify the integrity of the transactions in the block. If any of the transactions have been altered, the merkle root will change, and consequently, the hash of the previous block will not match, invalidating the transaction.
In this chapter, we will look at how merkle trees are used to verify the integrity of block transactions. After this, we will implement code for Helium merkle trees.
Tree Data Structures
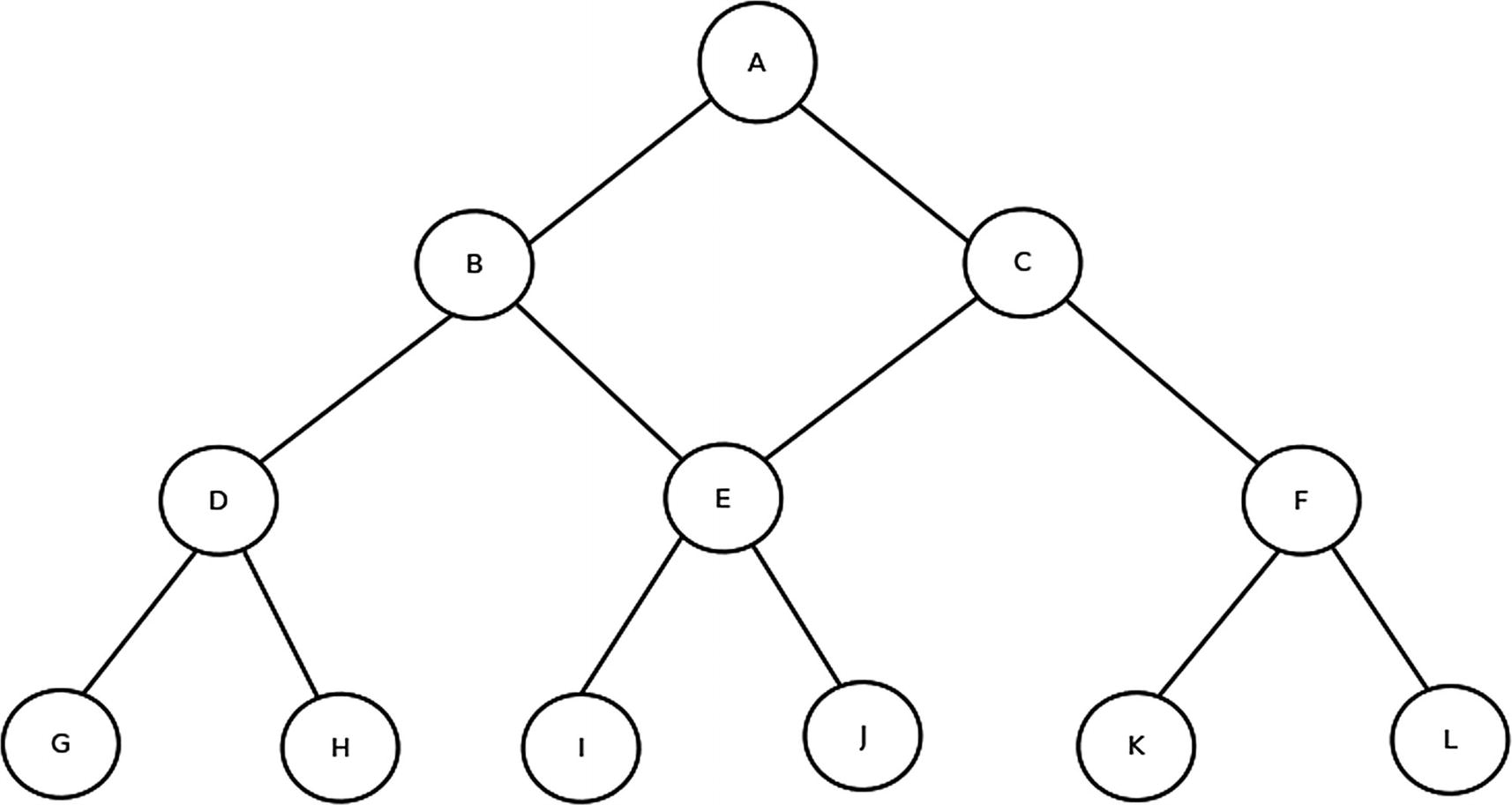
A Tree Data Structure
In this diagram, each node is represented by a circle. There are 12 nodes, labeled from A to L. These nodes are arranged in four layers. Each node, except for node A, is connected to a node above it with a line. The node above it is called a parent node. For example, D is a parent node of H, and C is a parent of E as well as F. Node A does not have a parent; it is called the root node. Aside from the nodes G to L, which are at the bottom of this tree structure, each node is connected to two nodes below it. These two nodes are called the children (or left and right children) of the node above (which is a parent node). For example, node E has two children, the I and J nodes. E is the parent of these nodes. The children nodes of a parent node are called siblings of each other. The nodes at the bottom of the tree do not have any children and are called leaf nodes.
This diagram is an example of a binary tree; all of the nodes except for the leaf nodes have two children. Furthermore, this is a balanced tree since all of the leaf nodes are at the same level. It is not necessary that each parent node have two children or that the tree be balanced. We can construct trees where nodes have a varying number of children and the tree is not balanced. However, balanced binary trees have very nice properties; in particular, we can do very fast insertions and deletions of nodes in the tree. Additionally, searching for a node in these trees is very fast.
Each node in a tree will typically contain pointers to its children, its parent node as well as data specific to the domain in which the tree is being used. The pointers in these nodes permit bidirectional movement through the tree.
Merkle Trees
In typical cryptocurrency implementations, the data portion of a block contains a collection of transactions. For example, a Bitcoin block may contain 2000–4000 transactions. In Helium, the transactions in a block are represented as a list. Each list element is a transaction, and each transaction has a dictionary structure. We will see shortly that we can represent this transaction list as a tree structure.
A merkle tree is a balanced binary tree where the nodes are related through cryptographic hashes. The root node of the merkle tree uniquely identifies the tree.
Suppose that we have a list of eight transactions in a block. We will construct the merkle tree of this list as follows.

Leaf Nodes of the Merkle Tree
TnhH is the SHA256 hash of the nth transaction. Since a merkle tree is a balanced binary tree, it must contain an even number of leaf nodes. Therefore, if we have an odd number of transactions in the block, we will simply add the last transaction twice to the transaction list.
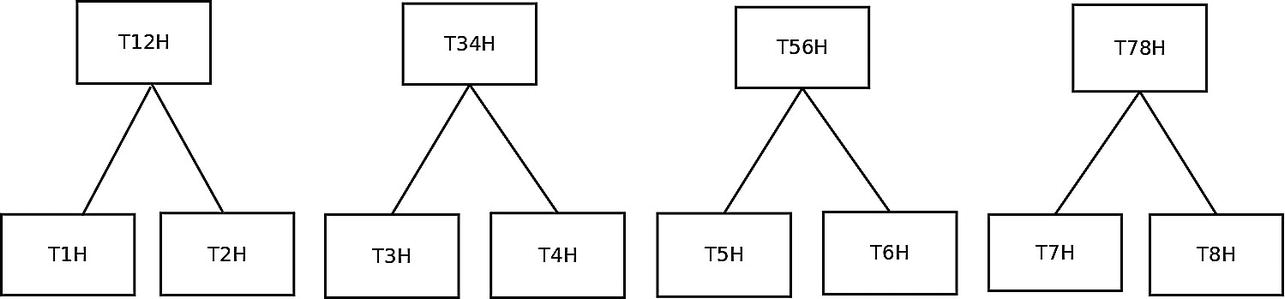
First Two Layers of the Merkle Tree
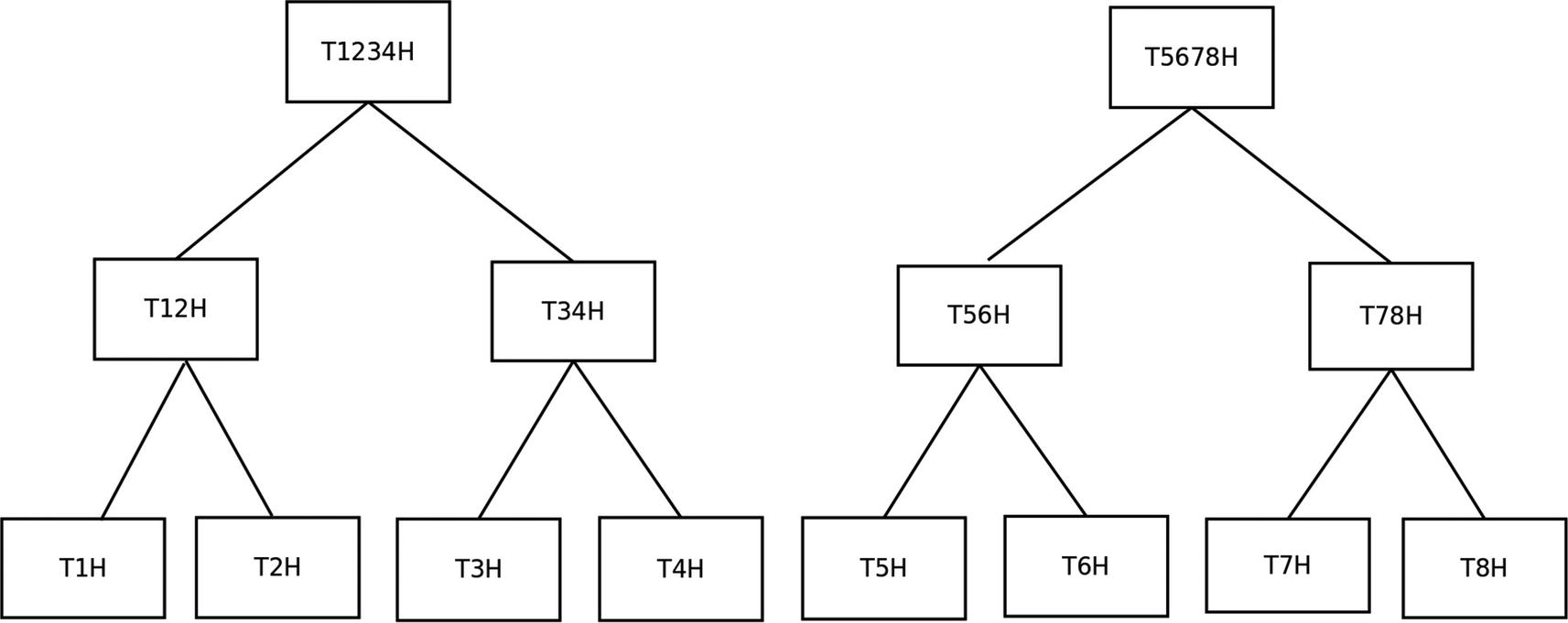
First Three Layers of the Merkle Tree
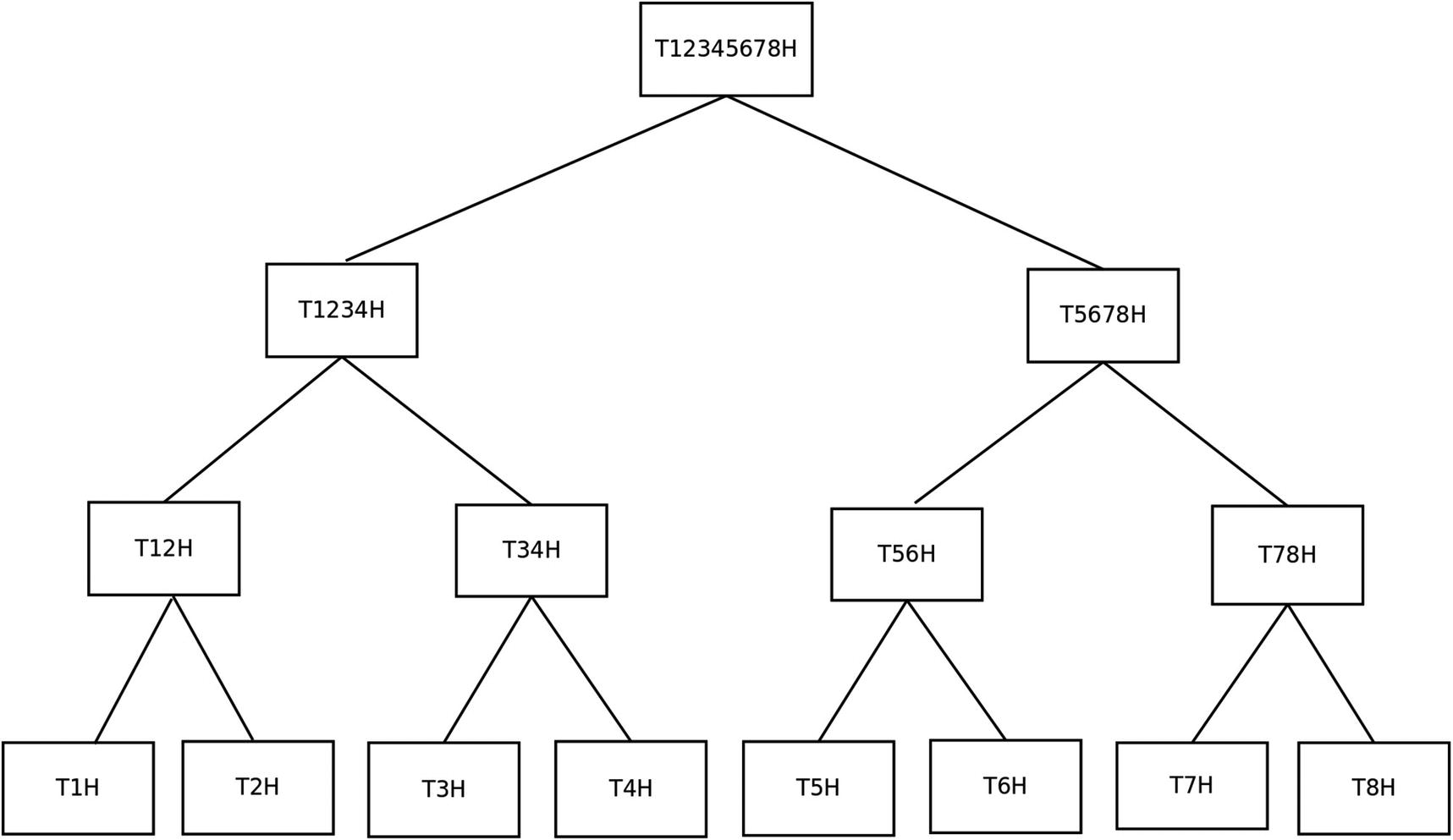
The Merkle Tree for the Eight Block Transactions
The hash value T12345678H is called the merkle root. It is a cryptographic hash of the transactions in the block. If any of the transactions are tampered with or if their order in the transaction list of the block is changed, the merkle root will be inconsistent with its previous value. Since the merkle root is in the block header, the block header will become inconsistent.
You may ask why we need to construct a merkle tree. The short answer is that a balanced binary tree such as a merkle tree is a remarkably efficient search structure. Consider a hypothetical list of one million block transactions. This list can be represented as a merkle tree with a depth of 20 layers. Furthermore, we can search for a particular transaction in this list in O(log n) time where n is the size of the number of transactions.
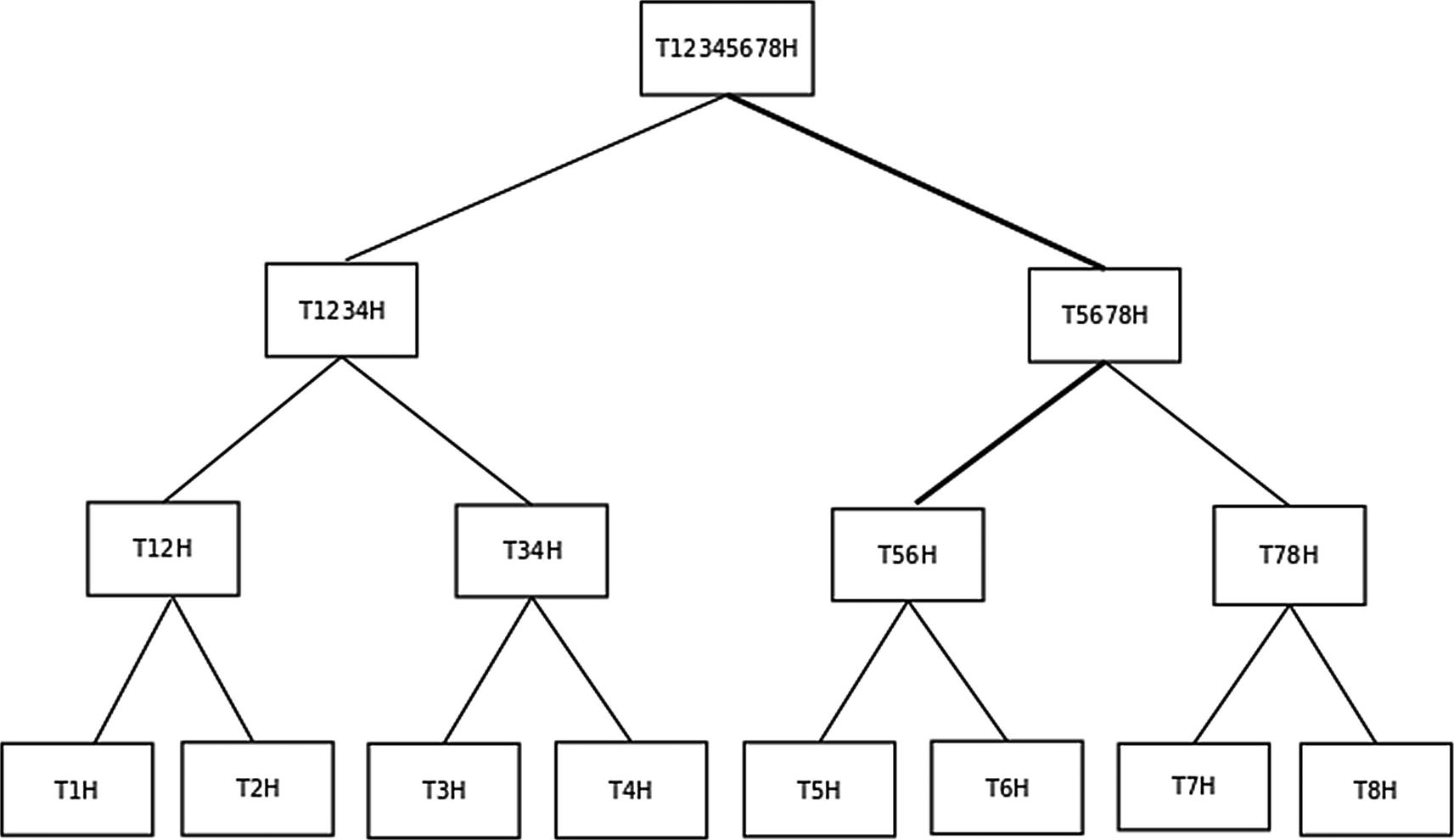
The Path to a Block Transaction
Deriving the Merkle Root in Helium
In Chapter 8, we wrote some of the Python code for the Helium blockchain. The function merkle_root in this module was stubbed out and returned a mocked value. We will now rectify this deficiency and code the function to compute and return the merkle root of a list of transactions in a block.
There are two functions: merkle_root and make_leaf_nodes. merkle_root receives a list of block transactions. merkle_root is called by the validate_block and blockheader_hash functions.
merkle_root is a recursive function; it receives a boolean flag as its second parameter. This flag is True if merkle_root is called from the aforementioned functions and False if this function has been called by itself. The very first thing that merkle_root does is to call the function make_leaf_nodes, whose task is to construct the leaf nodes of the merkle tree.
make_leaf_nodes performs some validation on the transaction list that it receives. If this list is empty, it returns False. An empty transaction list will have no leaf nodes. It then does some type checking to verify that it has received a list and not some other data structure. It also verifies that each list element is a dictionary type. If things are amiss, it returns a False value. Otherwise, make_leaf_nodes constructs the leaf nodes of the merkle tree for this transaction list and returns these values.
merkle_root then processes the leaf nodes to construct the merkle tree by calling itself recursively with the flag argument set to False.
As I previously indicated, Appendix 10 of this book contains the final source code for all of the Helium modules.
Pytests for the Merkle Code
We will now write some pytest unit tests to validate the merkle code. Add the following pytest code to the bottom of your test_blockchain module.
Conclusion
Merkle trees are important in blockchain applications for two principal reasons. Firstly, they permit us to efficiently test whether a list of transactions has been tampered with. Secondly, we can use these trees to verify whether a particular transaction is in a transaction list. In this chapter, we have examined merkle trees and written code to make a merkle tree from a list of transactions in a block.
In the next chapter, we will write transaction code for Helium and unit test this code.