Use optionals when a value cannot be set. Think of optionals as containers that can take either a value or nil. Optionals gives us the ability to check whether the value is nil or not. To create an optional value, you will have to provide it with a data type followed by a question mark (?). Before we do that, let's create a string that is not an optional. Add the following to Playgrounds:
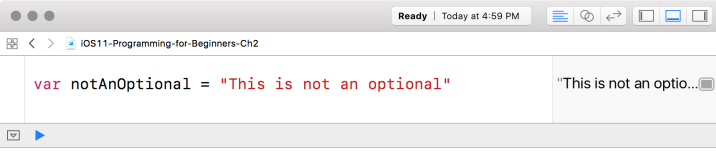
Now, let's add an optional to Playgrounds:

In this example, we created a string optional, and, if you notice in the Results Panel, it is nil. But for our notAnOptional, we see "This is not an optional". Now, on the next line, let's set optional equal to "This is an optional":

In our Results Panel, we see "This is an optional". Now let's print both notAnOptional and optional, as you will see a difference between the two:

Note that our notAnOptional variable looks fine, but optional has an optional ("") wrapped around the String. The ("") means that, in order for us to access the value, we must unwrap the optional. One way we could do this is by force-unwrapping the optional using an (!). Let's update our print statement and change it to the following:

We force-unwrapped our optional, but this method is not recommended. If you force-unwrap an optional and there is no value, your app will crash, so avoid this. We should use what is called optional binding, which is the safe way to access the value using an if...let statement. Remove the (!) from the print statement and instead write the following optional binding:
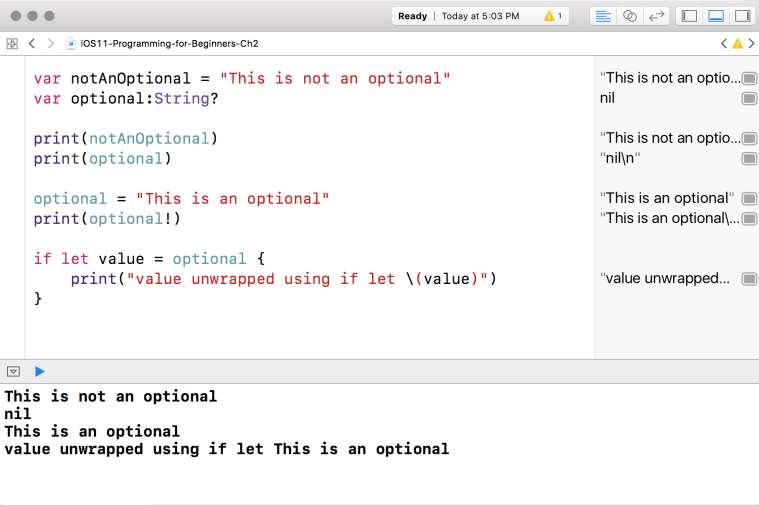
This if...let statement is saying that if the optional is not nil, set it to value, but, if this optional is nil, ignore it and do nothing. Now, we do not have to worry about anything regarding setting our value and causing our app to crash.