Continuing from the previous section, you will be configuring the Xamarin project for Android Auto:
- Open Visual Studio 2017.
- Go to the project through File | Open | Project / Solution, go to the MyPodCast directory, and choose the MyPodCast.sln file.
- Go to Tools | Android | Android SDK Manager.
- In the Platforms tab, go to Android 5.0 Lollipop and select Android SDK Platform 21.
- In Tools | Extras | Android Auto API Simulators and Android Auto Desktop Head Unit (DHU) emulator are selected.
- Click Apply Changes.
The following screenshot shows the Android SDK manager for installing DHU:

Installing DHU
- Download and unzip (https://bit.ly/2JF8jMq) the file to the MyPodCast\Resources directory. The downloaded file contains all the images and icons you will need to build the MyPodCast application.
- Go back to the Visual Studio MyPodCast project.
- Click on the MyPodCast project.
- Go to Project | Show All Files.
- Ctrl + click on each of the folders that you unzipped from step 7 to select them.
- Right-click on the selected folders and choose Include in Project.
The following screenshot shows the unzipped folders:
- Expand the Resources/drawable, right-click on icon.png, and select Include in Project.
- Right-click on MyPodCast project | Properties.
- Click the Application tab.
- From the Compiling using Android version (Target Framework) drop-down, choose Android 5.0 (Lollipop).
- Click on the Android Manifest tab.
- Enter the package name com.henry.mypodcast. This uniquely identifies the application.
- For the Application icon, choose @drawable/icon.
- For Minimum Android version and Target Android version, choose Android 5.0 (API Level 21 - Lollipop).
- In Required permissions, choose INTERNET and WAKE_LOCK. INTERNET permission allows your application to use the phone's internet connection and it is needed for streaming media. WAKE_LOCK permission stops the phone from going to sleep and is particularly required when you are streaming media.
- Ctrl + S to save the changes.
- Expand Properties and open AndroidManifest.xml.
- Add <meta-data android:name="com.google.android.gms.car.application" android:resource="@xml/automotive_app_desc" /> at the side of the application node. This will allow your application access to Android Auto features.
The following XML shows the completed AndroidManifest.xml:
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
android:versionCode="1"
android:versionName="1.0"
package="com.henry.mypodcast"
android:installLocation="auto">
<uses-sdk android:minSdkVersion="21" android:targetSdkVersion="21" />
<uses-permission android:name="android.permission.INTERNET" />
<uses-permission android:name="android.permission.WAKE_LOCK" />
<application android:icon="@drawable/icon" android:label="@string/app_name">
<meta-data android:name="com.google.android.gms.car.application"
android:resource="@xml/automotive_app_desc" />
</application>
</manifest>
- Right-click on the Resources folder | Add | New Folder called xml.
- Right-click on xml folder | Add New Item.
- Select XML File, name it automotive_app_desc.xml, and click Add.
The following screenshot shows adding automotive_app_desc.xml:

Adding an automotive_app_desc.xml
- The automotive_app_desc.xml file is referenced in AndroidManifest.xml and it will contain the Android Auto feature MyPodCast will be using. Add <automotiveApp> <uses name="media"/> </automotiveApp> to automotive_app_desc.xml.
The following XML adds an Android Auto media feature that can be used by MyPodCast:
<?xml version="1.0" encoding="utf-8"?>
<automotiveApp>
<uses name="media"/>
</automotiveApp>
- Add Helpers, MediaService, and UI folders by right-clicking on MyPodCast project| Add | New Folder. You will be adding C# programming files under these folders in the following sections.
The following screenshot shows adding a new folder:

Adding a new folder
- Right-click on MyPodCast | Manage NuGet Packages.
- Add Newton.Json and SkiaSharp.Views. Newton.Json is used for working with JSON format and SkiaSharp.Views is needed for working manipulating the downloaded images like the media covers shown on the Android Auto.
The following screenshot shows added NuGet packages:
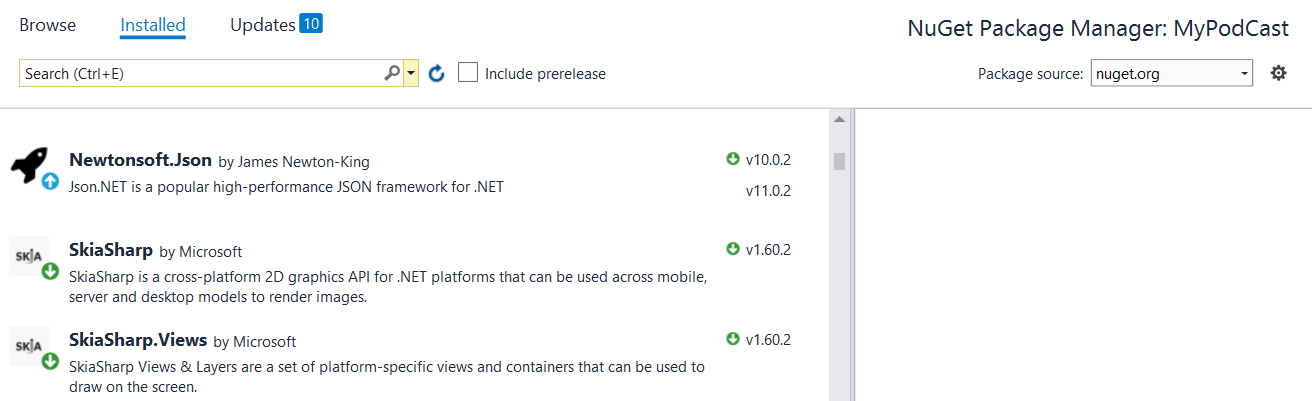
NuGet packages
You have now successfully prepared the MyPodCast Xamarin project for Android Auto. In the following section, you will be writing a program to serve media content to Android Auto.