Now that the basics are done, let's go ahead and build something meaningful using this system. Isn't it difficult to set your air-conditioner to the perfect temperature? No matter what you do, you end up feeling not in the most comfortable spot. This happens due to physiological changes in the body temperature over the course of the day.
When you wake up, your body temperature is relatively low. It is as much as 1° F, which is lower than the normal body temperature. As the day progresses, the body temperature rises until the time you hit the bed. Once you sleep, again your body temperature starts to dip reaching its lowest point around 4:00-6:00 am in the morning. That's the reason why what might feel warm while you go to bed, can be pretty cold when you wake up. Modern air-conditioners have something called a sleep mode. What this does is, it simply increases the temperature through the night. So that you do not feel cold at any point. But then again, how well it works is also a question.
So, now that we know the robotics very well, we will go ahead and make a system of our own that will take care of everything.
In this part, we will connect both the air-conditioner and your fan together so that they can both work in tandem and make you sleep well. Now, before jumping straight into it, I would like you to see the ratings that are mentioned on the relay. As you can see, the relay can handle only 250V and 5 ampere. Now, if you go through the brochure of your air-conditioner, you will easily understand why I am showing all of this to you. The power consumption of the air-conditioner will be much higher than what your relays can handle. So, if you try to run your air conditioner using the normal relays, then you will surely end up blowing the relay. There might be a chance that your appliance will be of a lower current rating than your relay. But with any device that has motors in it just keep in mind that the initial power consumption of that device is much higher than the nominal power consumption. Hence, if your air-conditioner needs 10 ampere nominal, then the starting load may be as much as 15 ampere. You must be thinking, it's not a problem, why don't we just purchase a relay that has a higher rating. Well, correct! That's exactly what we will be doing. But the naming of electronics can be tricky at times. The devices that deal with a higher-power higher-voltage electro-mechanical switching is generally called contractor instead of relay. Technically, they have the same working principal; However, there are construction differences, which at this point would not be our concern. So we will be using a contractor for the air conditioner switching and a dimmer for the fan speed control. Now that this has been cleared up, let's go ahead and attach the hardware as shown in the following diagram:

import RPi.GPIO as GPIO
import time
import Adafruit_DHT
GPIO.setmode(GPIO.BCM)
FAN = 18
AC = 17
pwm= GPIO.PWM(18,50)
GPIO.setup(FAN,GPIO.OUT)
GPIO.setup(AC, GPIO.OUT)
while True:
humidity, temperature = Adafruit_DHT.read_retry(sensor, pin)
if temperature =>20 && temperature <=30:
Duty = 50 + ((temperature-25)*10)
pwm.start(Duty)
if temperature <22 :
GPIO.output(AC, GPIO.LOW)
if temperature >= 24
GPIO.output(AC, GPIO.HIGH)}
The logic used here is pretty basic. Let's see what it is doing:
humidity, temperature = Adafruit_DHT.read_retry(sensor, pin)
if temperature =>20 && temperature <=30:
Duty = 50 + ((temperature-25)*10)
pwm.start(Duty)
Here we are taking the value of humidity and temperature. So far so good, but can we take it a step further and make it even more intelligent? The previous logic must have helped you sleep better, but can we make it just perfect for you?
There are multiple indicators in our body that give us an idea of what the state of the body is. For example, if you are tired, you will probably not be walking very fast or talking very loud. Instead, you would be doing the opposite! Similarly, there are multiple factors that indicate how our sleep cycle is going.
Some of these factors are: body temperature, respiration rate, REM sleep, and body movements. Measuring the exact body temperature or respiration rate and REM sleep is something of a challenge. But when we talk about body movements, I think we have already perfected it. So based on the body movements, we will be sensing how well we are sleeping and what kind of temperature adjustment is needed.
If you notice, whenever someone is sleeping and starts feeling cold, the body will go to a fetal position and will move much less. This happens automatically. However, when a person is comfortable, there are some inevitable movements such as changing sides and movement of arms or legs. This does not happen when a person is feeling cold. So with these movements we can figure out whether a person is feeling cold or not. Now that we have understood the physiological changes of the body, let's try to build a program around it and see what we can achieve.
To do this, firstly, we need to connect the circuit as follows:
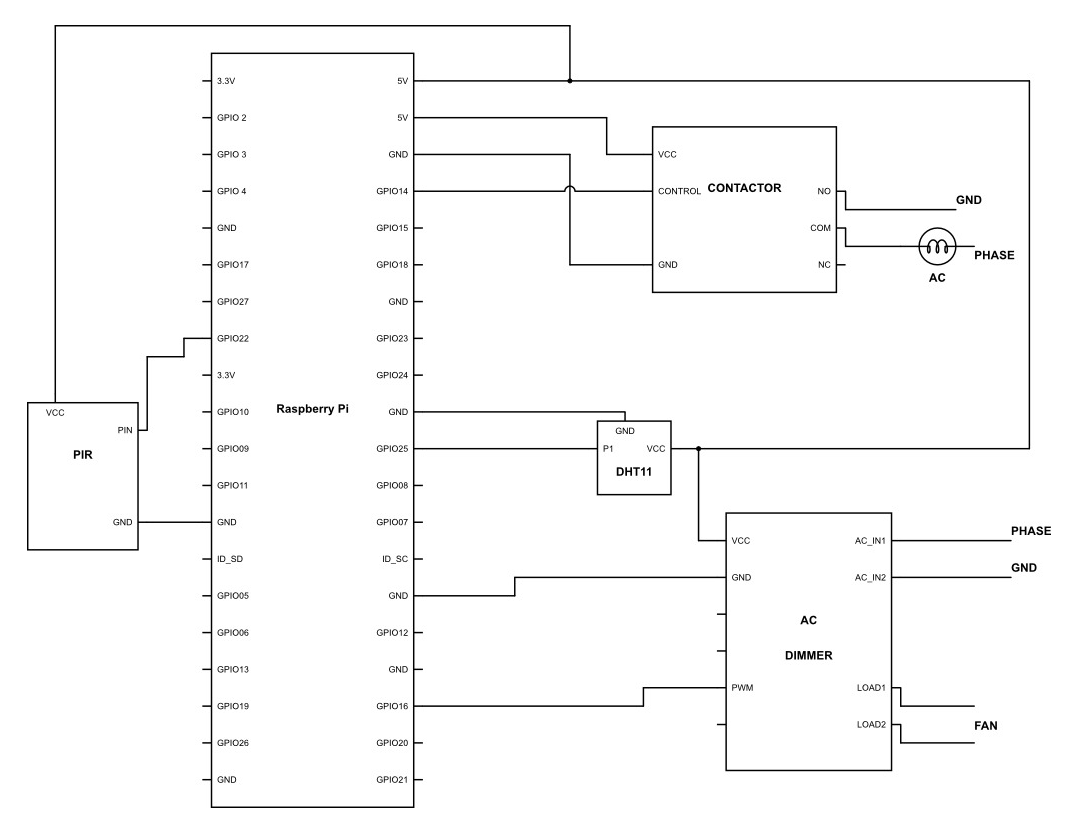
Once this is done, go ahead and write the following code:
import RPi.GPIO as GPIO
import time
import Adafruit_DHT
GPIO.setmode(GPIO.BCM)
FAN = 18
AC = 17
PIR = 22
PIN = 11
Sensor = 4
pwm= GPIO.PWM(18,50)
GPIO.setup(FAN,GPIO.OUT)
GPIO.setup(AC, GPIO.OUT)
while True:
humidity, temperature = Adafruit_DHT.read_retry(sensor, pin)
H = datetime.datetime.now().strftime('%H')
M = datetime.datetime.now().strftime('%M')
if H <= 6 && H <= 22:
if M <=58 :
M = datetime.datetime.now().strftime('%M')
humidity, temperature = Adafruit_DHT.read_retry(sensor, pin)
if GPIO.input(PIR) == 0 :
Movement = Movement + 1
time.sleep(10)
if temperature < 28:
if Movement > 5 :
Duty = Duty + 10
pwm.start(Duty)
Movement = 0
if M = 59 :
if Movement = 0 :
Duty = Duty -10
pwm.start(Duty)
Movement = 0
if temperature <22 :
GPIO.output(AC, GPIO.LOW)
if temperature >= 24 && H <= 6 && H >= 22:
GPIO.output(AC, GPIO.HIGH)
if temperature > 27
pwm.start(100)
for H > 7 && H < 20
GPIO.output(AC, GPIO.LOW)
if H = 20
GPIO.output(AC,GPIO.HIGH)
}
Let's have a look at what is going on under the hood:
if H <= 6 && H <= 22:
if M <=58 :
M = datetime.datetime.now().strftime('%M')
humidity, temperature = Adafruit_DHT.read_retry(sensor, pin)
The first thing you will see is that we have a condition: if H,= 6 && H<= 22:. This condition will only be true if the time frame is between 10 o'clock in the morning and 6 o'clock in the night. That is because this is the time when we generally sleep. Hence, the logic under this head will only work if it's time to sleep.
The second condition is if M <= 58, which will be true only when the time is between 0 and 58 minutes. So when the time is M = 59, then this condition will not work. We will see the reason for having this logic.
Thereafter, we are calculating the time and storing the value in a variable called M. We are also calculating the humidity and temperature values and storing it in variables called temperature and humidity:
if GPIO.input(PIR) == 0 :
Movement = Movement + 1
time.sleep(10)
Now, in this line, we are implementing a condition which will be true if the reading from the PIR is high. That is, there is some motion that will be detected. Whenever this happens, the Movement variable will be incremented by 1. Finally, we are using the time.sleep(10) function to wait for 10 seconds. This is done as the PIR might be high for a momentary period. In that case, the condition will be true over and over again which in turn will increment the value of Movement multiple times.
Our purpose of incrementing the value of Movement is to count the number of times the person has moved. Hence, incrementing it multiples times in one single time will defy the objective.
if temperature < 28:
if Movement > 5 :
Duty = Duty + 10
pwm.start(Duty)
Movement = 0
Now we have another condition, which says if temperature < 28. Not much explanation is needed for when the condition will be true. So whenever the condition is true and if the counted number of Movement is more than 5, the value of Duty will be incremented by 10. Therefore, we are sending the PWM to the AC dimmer, which in turn will increase the speed of the fan. Finally, we are resetting the value of Movement to 0.
So essentially, we are just counting the number of movements. This movement is counted only if the temperature is less than 28° C. If the movement is more than 5, then we will increase the speed of the fan by 10%.
if M = 59 :
if Movement = 0 :
Duty = Duty -10
pwm.start(Duty)
Movement = 0
In the previous section, the logic will only work when the time is between 0 and 58, that is, the time in which the counting will happen. When the value of M is 59, then the condition if Movement = 0 will be checked, and if true, then the value of Duty will be decremented by 10. This in turn will reduce the speed of the fan by 10%. Also, once this condition is executed, the value of Movement will be reset to 0. So then a new cycle can start for the next hour.
Now what it basically means is that counting will happen on an hourly basis. If the Movement is more than 5 then immediately the value of the Duty would be increased. However, if that is not the case, then the program will wait until the minute approaches the value of 59 and whenever that happens, it will check whether there is any movement, in which case, the fan speed will be decreased.
if temperature <22 :
GPIO.output(AC, GPIO.LOW)
if temperature >= 24 && H <= 6 && H >= 22:
GPIO.output(AC, GPIO.HIGH)
if temperature > 27
pwm.start(100)
All of this code is very straightforward. If the temperature is less than 22, then the AC will be switched off. Furthermore, if the temperature is equal to or more than 24, and time is between 10:00 p.m. and 6:00 a.m., then the AC will be turned on. Finally, if the temperature is more than 27, then the fan will be switch on to 100% speed.
for H > 7 && H < 20
GPIO.output(AC, GPIO.LOW)
if H = 20
GPIO.output(AC,GPIO.HIGH)
Finally, we are making sure by using the condition for H > 7 && H <20 that during this time the AC is always switched off. Also, if H = 20, then the AC should be turned on so that the room is cooled before you are ready to sleep.