Let's try turning on/off appliances at home using the Flask framework. In previous chapters, we made use of PowerSwitch Tail II to control a table lamp using the Raspberry Pi Zero. Let's try to control the same using the Flask framework. Connect PowerSwitch Tail, as shown in the following figure:
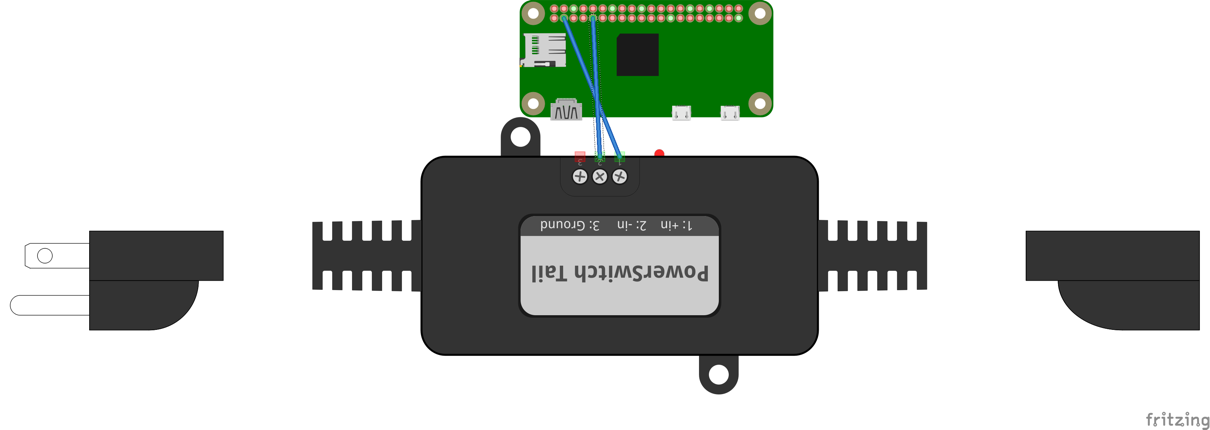
According to the Flask framework documentation, it is possible to route a URL to a specific function. For example, it is possible to bind /lamp/<control> using route() to the control() function:
@app.route("/lamp/<control>")
def control(control):
if control == "on":
lights.on()
elif control == "off":
lights.off()
return "Table lamp is now %s" % control
In the preceding code snippet, <control> is a variable that can be passed on as an argument to the binding function. This enables us to turn the lamp on/off. For example, <IP address>:5000/lamp/on turns on the lamp, and vice versa. Putting it all together, we have this:
#!/usr/bin/python3
from flask import Flask
from gpiozero import OutputDevice
app = Flask(__name__)
lights = OutputDevice(2)
@app.route("/lamp/<control>")
def control(control):
if control == "on":
lights.on()
elif control == "off":
lights.off()
return "Table lamp is now %s" % control
if __name__ == "__main__":
app.run('0.0.0.0')
The preceding example is available for download along with this chapter as appliance_control.py. Launch the Flask-based web server and open a web server on another computer. In order to turn on the lamp, enter <IP Address of the Raspberry Pi Zero>:5000/lamp/on as URL:
This should turn on the lamp:

Thus, we have built a simple framework that enables controlling appliances within the network. It is possible to include buttons to an HTML page and route them to a specific URL to perform a specific function. There are several other frameworks in Python that enable developing web applications. We have merely introduced you to different applications that are possible with Python. We recommend that you check out this book's website for more examples, such as controlling Halloween decorations and other holiday decorations using the Flask framework.