- Import the necessary packages:
import sys import cv2 import numpy as np
- Load the input image:
in_file = sys.argv[1] image = cv2.imread(in_file)
- Convert the RGB image into grayscale:
image_gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY) cv2.imshow('Input grayscale image', image_gray)
- Regulate the histogram of the grayscale image:
image_gray_histoeq = cv2.equalizeHist(image_gray) cv2.imshow('Histogram equalized - grayscale image', image_gray_histoeq)
- Regulate the histogram of the RGB image:
image_yuv = cv2.cvtColor(image, cv2.COLOR_BGR2YUV) image_yuv[:,:,0] = cv2.equalizeHist(image_yuv[:,:,0]) image_histoeq = cv2.cvtColor(image_yuv, cv2.COLOR_YUV2BGR)
- Display the output image:
cv2.imshow('Input image', image) cv2.imshow('Histogram equalized - color image', image_histoeq) cv2.waitKey()
- The command used to execute the histogram.py Python program file, along with the input image (finger.jpg), is shown here:

- The input image used to execute the histogram.py file is shown here:

- The histogram equalized grayscale image obtained after executing the histogram.py file is shown here:

- The histogram equalized color image obtained after executing the histogram.py file is shown here:
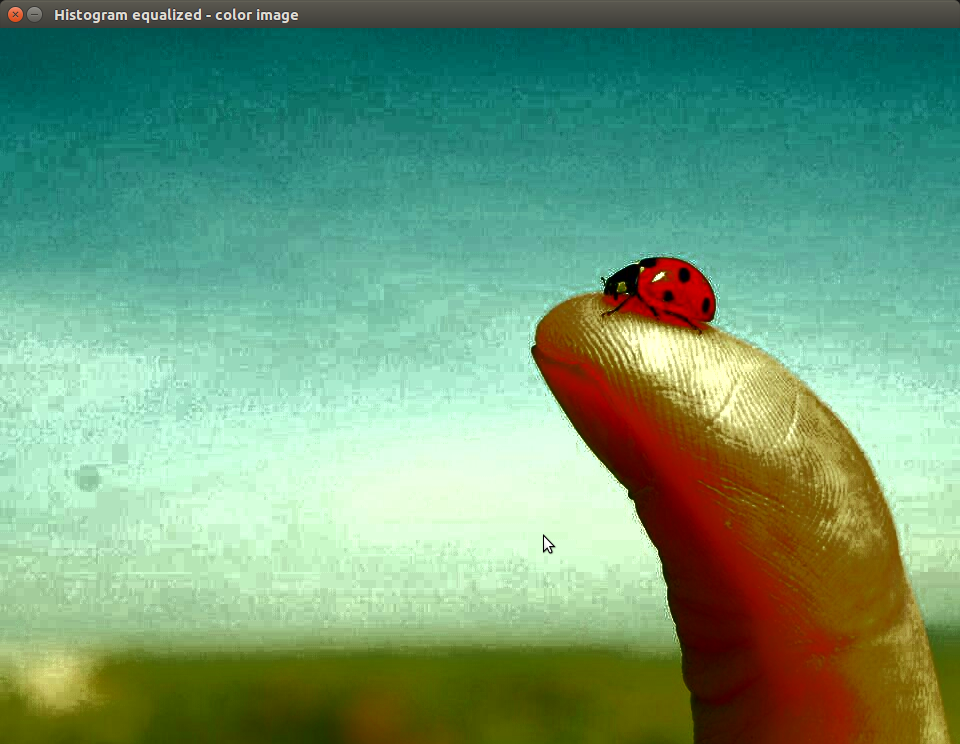