As you remember, other than MainActivity, we also have some more activities. In our application, we created activities to create/edit Notes and TODOs. Our plan is to connect them to the button click events, and then, when the user clicks on the button, the proper screen will open. We will start by defining anĀ enum that represents an operation that we will perform in an opened activity. When we open it, we can view, create, or update Note or Todo. Create a new package called model and enum with the name MODE. Make sure you have the following enum values:
enum class MODE(val mode: Int) { CREATE(0), EDIT(1), VIEW(2); companion object { val EXTRAS_KEY = "MODE" fun getByValue(value: Int): MODE { values().forEach { item -> if (item.mode == value) { return item } } return VIEW } } }
We added a few additions here. In enum's companion object, we defined the extras key definition. Soon, you will need it, and you will understand its purpose. We also created a method that will give us an enum based on its value.
As you probably remember, both activities for working with Notes and Todos share the same class. Open ItemActivity and extend it as follows:
abstract class ItemActivity : BaseActivity() { protected var mode = MODE.VIEW override fun getActivityTitle() = R.string.app_name override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) val modeToSet = intent.getIntExtra(MODE.EXTRAS_KEY,
MODE.VIEW.mode) mode = MODE.getByValue(modeToSet) Log.v(tag, "Mode [ $mode ]") } }
We introduced a field mode of the type we just defined that will tell us if we are viewing, creating, or editing a Note or Todo item. Then, we overrode the onCreate() method. This is important! When we click on the button and open the activity, we will pass some values to it. This code snippet retrieves the value we passed. To achieve that, we access the Intent instance (in the next section, we will explain intents) and the integer field called MODE (value of MODE.EXTRAS_KEY). The method that gives us this value is called getIntExtra(). There is a version of the method for every type. If there is no value, MODE.VIEW.mode is returned. Finally, we set mode to a value we obtained by getting the MODE instance from the integer value.
The last piece of the puzzle is triggering an activity opening. Open ItemsFragment and extend it as follows:
class ItemsFragment : BaseFragment() { ... override fun onCreateView( inflater: LayoutInflater?, container: ViewGroup?, savedInstanceState: Bundle? ): View? { val view = inflater?.inflate(getLayout(), container, false) val btn = view?.findViewById<FloatingActionButton>
(R.id.new_item) btn?.setOnClickListener { val items = arrayOf( getString(R.string.todos), getString(R.string.notes) ) val builder =
AlertDialog.Builder(this@ItemsFragment.context) .setTitle(R.string.choose_a_type) .setItems( items, { _, which -> when (which) { 0 -> { openCreateTodo() } 1 -> { openCreateNote() } else -> Log.e(logTag, "Unknown option selected
[ $which ]") } } ) builder.show() } return view } private fun openCreateNote() { val intent = Intent(context, NoteActivity::class.java) intent.putExtra(MODE.EXTRAS_KEY, MODE.CREATE.mode) startActivity(intent) } private fun openCreateTodo() { val intent = Intent(context, TodoActivity::class.java) intent.putExtra(MODE.EXTRAS_KEY, MODE.CREATE.mode) startActivity(intent) } }
We accessed the FloatingActionButton instance and assigned a click listener. On clicking, we will create a dialog with two options. Each of these options will trigger a proper method for activity opening. The implementation of both methods is very similar. As an example, we will focus on openCreateNote().
We will create a new Intent instance. In Android, Intent represents our intention to do something. To start an activity, we must pass the context and the class of the activity we want to start. We must also assign some values to it. Those values will be passed to an activity instance. In our case, we are passing the integer value for the MODE.CREATE. startActivity() method that will execute the intent and a screen will appear.
Run the application, click on the rounded button at the bottom-right corner of your screen, and choose an option from the dialog, as shown in the following screenshot:

This will take you to this screen:
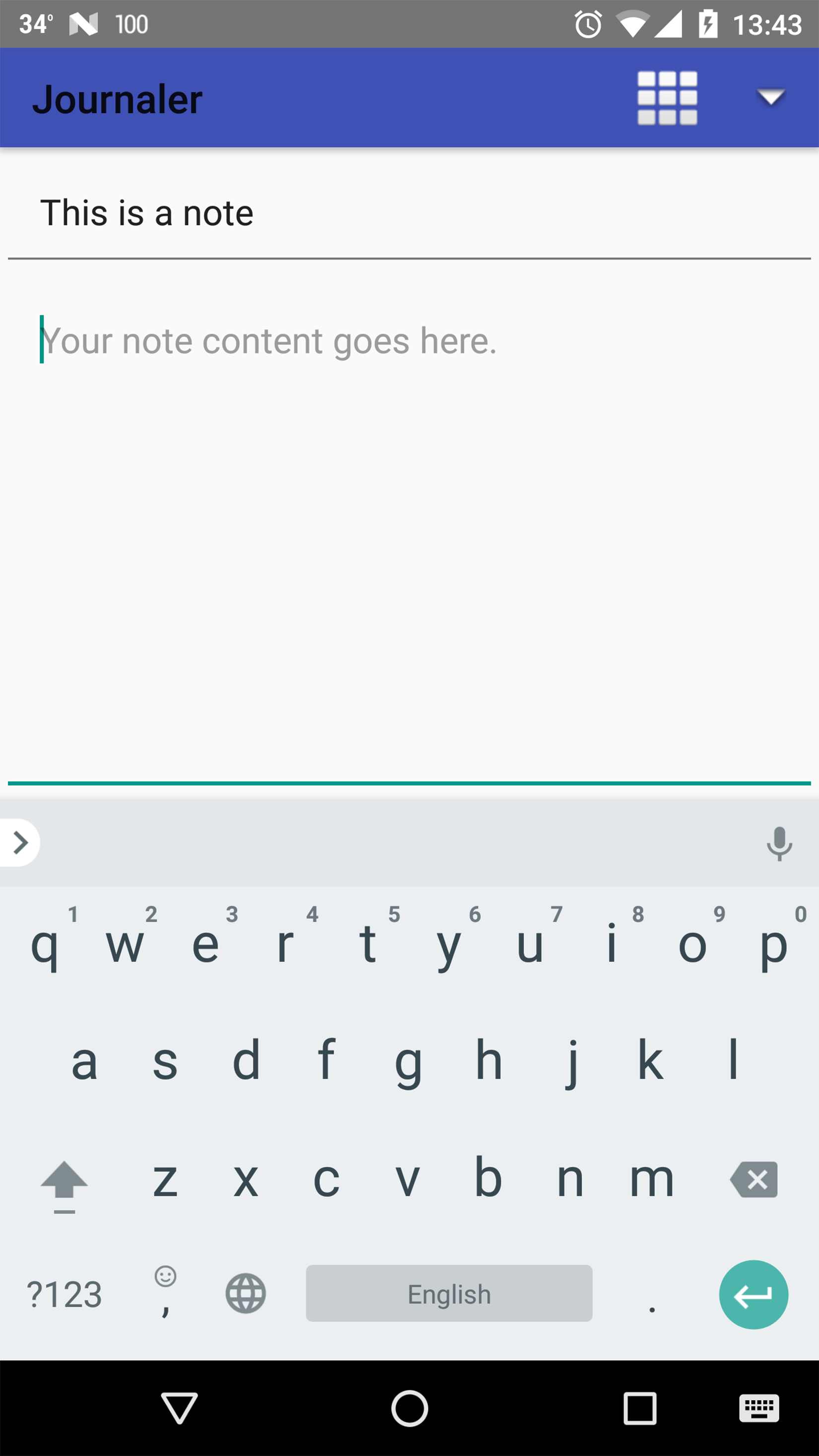
This will take you further to add your own data with date and time:
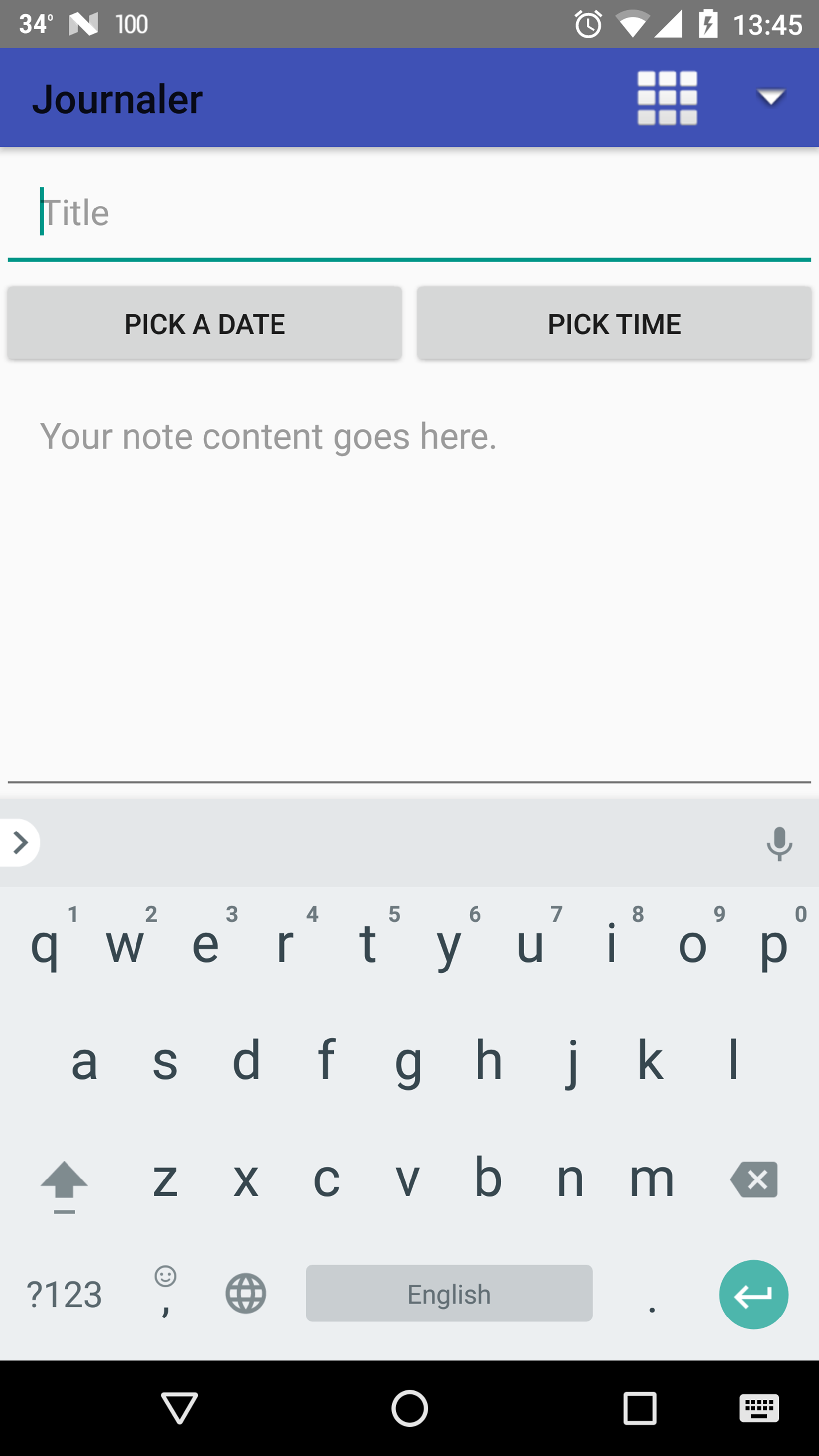