To pass information between our activities, we will use the Android Bundle. Bundle can contain multiple values of different types. We will illustrate the use of Bundle by extending our code. Open ItemsFragemnt and update it as follows:
private fun openCreateNote() { val intent = Intent(context, NoteActivity::class.java) val data = Bundle() data.putInt(MODE.EXTRAS_KEY, MODE.CREATE.mode) intent.putExtras(data) startActivityForResult(intent, NOTE_REQUEST) }
private fun openCreateTodo() { val date = Date(System.currentTimeMillis()) val dateFormat = SimpleDateFormat("MMM dd YYYY", Locale.ENGLISH) val timeFormat = SimpleDateFormat("MM:HH", Locale.ENGLISH) val intent = Intent(context, TodoActivity::class.java) val data = Bundle() data.putInt(MODE.EXTRAS_KEY, MODE.CREATE.mode) data.putString(TodoActivity.EXTRA_DATE, dateFormat.format(date)) data.putString(TodoActivity.EXTRA_TIME,
timeFormat.format(date)) intent.putExtras(data) startActivityForResult(intent, TODO_REQUEST) } override fun onActivityResult(requestCode: Int, resultCode: Int,
data: Intent?) { super.onActivityResult(requestCode, resultCode, data) when (requestCode) { TODO_REQUEST -> { if (resultCode == Activity.RESULT_OK) { Log.i(logTag, "We created new TODO.") } else { Log.w(logTag, "We didn't created new TODO.") } } NOTE_REQUEST -> { if (resultCode == Activity.RESULT_OK) { Log.i(logTag, "We created new note.") } else { Log.w(logTag, "We didn't created new note.") } } } }
Here, we introduced some important changes. First of all, we started our Note and Todo activities as sub activities. This means that our MainActivity class depends on the result of the work of those activities. When starting the sub activity instead of the startActivity() method, we used startActivityForResult(). Parameters we passed are the intent and request number. To get a result of the execution, we overrode the onActivityResult() method. As you can see, we checked which activity finished and if that execution produced a successful result.
We also changed the way we pass the information. We created the Bundle instance and assigned multiple values, like in case of the Todo activity. We added mode, date, and time. Bundle is assigned to intent using the putExtras() method. To use these extras, we updated our activities too. Open ItemsActivity and apply changes, like this:
abstract class ItemActivity : BaseActivity() { protected var mode = MODE.VIEW protected var success = Activity.RESULT_CANCELED override fun getActivityTitle() = R.string.app_name override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) val data = intent.extras data?.let{ val modeToSet = data.getInt(MODE.EXTRAS_KEY, MODE.VIEW.mode) mode = MODE.getByValue(modeToSet) } Log.v(tag, "Mode [ $mode ]") } override fun onDestroy() { super.onDestroy() setResult(success) } }
Here, we introduced the field holding result of activity work. We also updated the way we handle the passed information. As you can see, if there are any extras available, we will obtain an integer value for the mode. And, finally, the onDestroy() method sets the result of the work that will be available for parent activity.
Open TodoActivity and apply the following changes:
class TodoActivity : ItemActivity() { companion object { val EXTRA_DATE = "EXTRA_DATE" val EXTRA_TIME = "EXTRA_TIME" } override val tag = "Todo activity" override fun getLayout() = R.layout.activity_todo override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) val data = intent.extras data?.let { val date = data.getString(EXTRA_DATE, "") val time = data.getString(EXTRA_TIME, "") pick_date.text = date pick_time.text = time } } }
We have obtained values for date and time extras and set them to date/time picker buttons. Run your application and open the Todo activity. Your Todo screen should look like this:
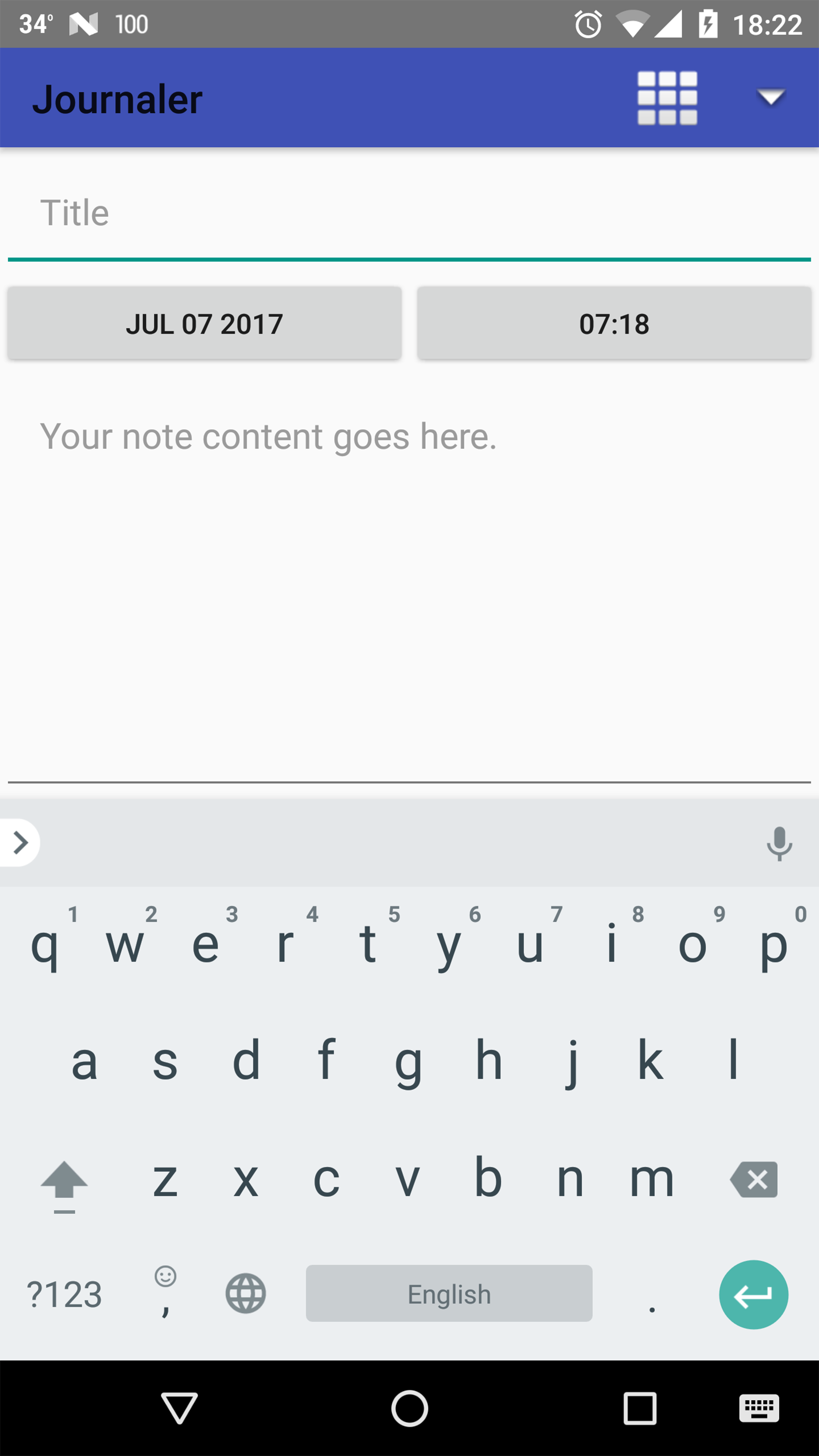
When you leave the Todo activity and return back to the main screen, observe your Logcat. There will be a log with the following content:
W/Items fragment--we didn't create a new TODO.
Since we have not created any Todo items yet, we passed a proper result. We canceled the creation process by going back to the main screen. In the later chapters, and the ones following, we will create Notes and Todos with success.