To write the C++ code for video players, we perform the following steps:
- For mainwindow.h, there aren't many changes to it. All we need to do is to include QVideoWidget in the header:
#include <QMainWindow> #include <QDebug> #include <QFileDialog> #include <QMediaPlayer> #include <QMediaMetaData> #include <QTime> #include <QVideoWidget>
- Then, open mainwindow.cpp. We must define a QVideoWidget object and set it as the video output target, before adding it to the layout of the QFrame object we just added in the previous step:
MainWindow::MainWindow(QWidget *parent) : QMainWindow(parent), ui(new Ui::MainWindow) { ui->setupUi(this); player = new QMediaPlayer(this); QVideoWidget* videoWidget = new QVideoWidget(this); player->setVideoOutput(videoWidget); ui->movieLayout->addWidget(videoWidget); player->setVolume(ui->volume->value()); connect(player, &QMediaPlayer::stateChanged, this, &MainWindow::stateChanged); connect(player, &QMediaPlayer::positionChanged, this, &MainWindow::positionChanged); }
- In the slot function thatgets called when the Open File action has been triggered, we simply change the file-selection dialog to accept only MP4 and MOV formats. You can add in other video formats too if you wish:
QString fileName = QFileDialog::getOpenFileName(this, "Select Movie File", qApp->applicationDirPath(), "MP4 (*.mp4);;MOV (*.mov)");
That's it. The rest of the code is literally the same as the audio player example. The main difference with this example is that we defined the video output widget, and Qt will handle the rest for us.
If we build and run the project now, we should be getting a really slick video player, like what you see here:
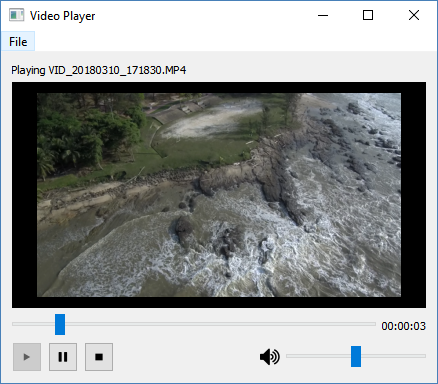
On a windows system, there was a case where the video player would throw an error. This problem is similar to the one reported here: https://stackoverflow.com/questions/32436138/video-play-returns-directshowplayerservicedoseturlsource-unresolved-error-cod
To resolve this error, simply download and install the K-Lite_Codec_Pack which you can find here: https://www.codecguide.com/download_k-lite_codec_pack_basic.htm. After this, the video should play like a charm!
To resolve this error, simply download and install the K-Lite_Codec_Pack which you can find here: https://www.codecguide.com/download_k-lite_codec_pack_basic.htm. After this, the video should play like a charm!