Writing code, however, is still required in order for the widgets to be fully functional in your application. Let's learn how to add items to our item view widgets using C++ code!
First, open up mainwindow.cpp and write the following code to the class constructor, right after ui->setupui(this):
ui->listWidget->addItem("My Test Item");
As simple as that, you have successfully added an item to the List Widget!
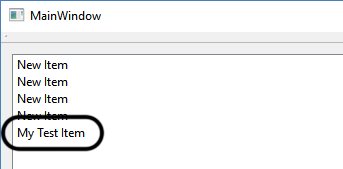
There is another way to add an item to the List Widget. But before that, we must add the following headers to mainwindow.h:
#ifndef MAINWINDOW_H #define MAINWINDOW_H #include <QMainWindow> #include <QDebug> #include <QListWidgetItem>
The QDebug header is for us to print out debug message, and the QListWidgetItem header is for us to declare List Widget Item objects. Next, open up mainwindow.cpp and add the following code:
QListWidgetItem* listItem = new QListWidgetItem; listItem->setText("My Second Item"); listItem->setData(100, 1000); ui->listWidget->addItem(listItem);
The preceding code does the same as the previous one-line code. Except, this time, I've added an extra data to the item. The setData() function takes in two input variables—the first variable is the data-role of item, which indicates how it should be treated by Qt. If you put a value that matches the Qt::ItemDataRole enumerator, the data will affect the display, decoration, tooltip, and so on, and that may change its appearance.
In my case, I just simply set a number that doesn't match any of the enumerators in Qt::ItemDataRole so that I can store it as a hidden data for later use. To retrieve the data, you can simply call data() and insert the number that matches the one you've just set:
qDebug() << listItem->data(100);
Build and run the project; you should be able to see that the new item is now being added to the List Widget:
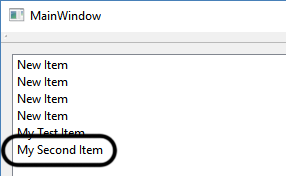
As mentioned earlier, hidden data can be attached to a list item for later use. For example, you could use the list widget to display a list of products ready to be purchased by the user. Each of these items can be attached with its product ID so that when the user selects the item and places it on the cart, your system can automatically identify which product has been added to the cart by identifying the product ID stored as the data role.
In the preceding example, I stored custom data, 1000, in my list item and set its data role as 100, which does not match any of the Qt::ItemDataRole enumerators. This way, the data won't be shown to the users, and thus it can only be retrieved through C++ code.