After that, add in the following code to the HTML file we just created:
<!DOCTYPE html><html> <head> <title>Page Title</title> </head> <body> <p>Hello World!</p> </body> </html>
These are the basic HTML tags which show you nothing other than a line of words that says Hello World!. You can try and load it using your web browser:

After that, let's go back to our Qt project and go to File | New File or Project and create a Qt Resource File:
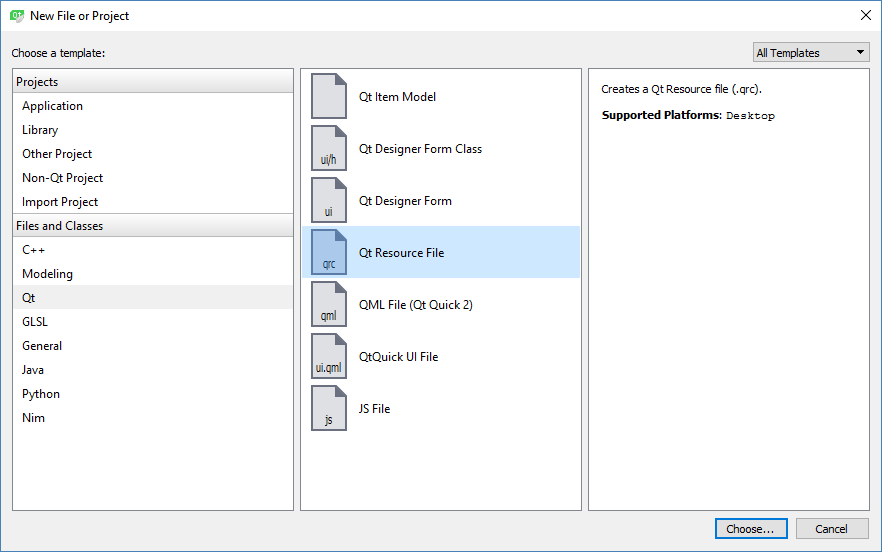
Then, open up the Qt resource file we just created and add in an /html prefix followed by adding the HTML file to the resource file, like so:

Right-click on text.html while the resource file is still opened, then select Copy Resource Path to Clipboard. Right after that, change the URL of your web view to:
webview->load(QUrl("qrc:///html/test.html"));
You can use the link you just copied from the resource file, but make sure you add the URL scheme qrc:// at the front of the link. Build and run your project now and you should be able to see the result instantly:

Next, we need to set up a function in JavaScript that will be called by C++ in just a moment. We'll just create a simple function that pops up a simple message box and changes the Hello World! text to something else when called:
<!DOCTYPE html> <html> <head> <title>Page Title</title> <script> function hello() { document.getElementById("myText").innerHTML =
"Something happened!"; alert("Good day sir, how are you?"); } </script> </head> <body> <p id="myText">Hello World!</p> </body> </html>
Note that I have added an ID to the Hello World! text so that we are able to find it and change its text. Once you're done, let's go to our Qt project again.
Let's proceed to add a push button to our program UI, and when the button is pressed, we want our Qt program to call the hello() function we just created in JavaScript. It's actually very easy to do that in Qt; you simply call the runJavaScript() function from the QWebEnginePage class, like so:
void MainWindow::on_pushButton_clicked() { webview->page()->runJavaScript("hello();"); }
The result is pretty astounding, as you can see from the following screenshot:
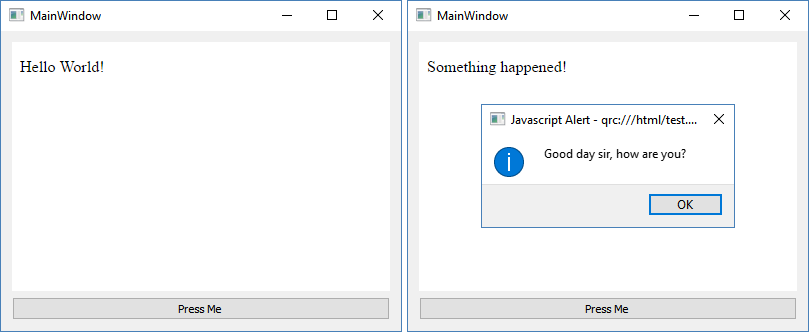
You can do a lot more than just change the text or call a message box. For example, you can start or stop an animation in an HTML canvas, show or hide an HTML element, trigger an Ajax event to retrieve information from a PHP script, and so on and so forth... endless possibilities!