In the previous example, we have successfully drawn some simple shapes and text onto the Graphics View widget. However, these graphics items are not interactive and thus don't suit our purpose. What we want is an interactive organization chart where the user can move the items around using mouse. It is actually really easy to make these items movable under Qt; let's see how we can do that by continuing our previous project.
First, make sure you don't change the default interactive property of our Graphics View widget, which is set to enabled (checkbox is checked):
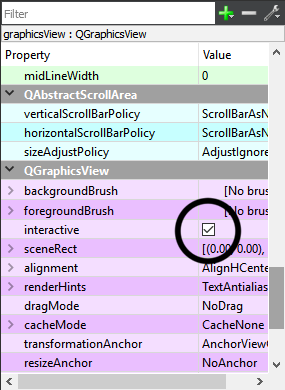
After that, add the following code below each of the graphics items we just created in the previous Hello World example:
QGraphicsRectItem* rectangle = scene->addRect(80, 0, 80, 80, pen, greenBrush); rectangle->setFlag(QGraphicsItem::ItemIsMovable); rectangle->setFlag(QGraphicsItem::ItemIsSelectable); QGraphicsEllipseItem* ellipse = scene->addEllipse(0, -80, 200, 60, pen, blueBrush); ellipse->setFlag(QGraphicsItem::ItemIsMovable); ellipse->setFlag(QGraphicsItem::ItemIsSelectable); QGraphicsTextItem* text = scene->addText("Hello World!", QFont("Times", 25)); text->setFlag(QGraphicsItem::ItemIsMovable); text->setFlag(QGraphicsItem::ItemIsSelectable);
Build and run the program again, and this time you should be able to select and move the items around the Graphics View. Do note that ItemIsMovable and ItemIsSelectable both give you a different behavior—the former flag will make the item movable by mouse, and the latter makes the item selectable, which typically gives it a visual indication using dotted outline when selected. Each of the flags works independently and will not affect the other.
We can test out the effect of ItemIsSelectable flag by using the signal and slot mechanism in Qt. Let's go back to our code and add the following line:
ui->setupUi(this); scene = new QGraphicsScene(this); ui->graphicsView->setScene(scene);
connect(scene, &QGraphicsScene::selectionChanged, this, &MainWindow::selectionChanged);
The selectionChanged() signal will be triggered whenever you selected an item on the Graphics View widget and the selectionChanged() slot function under our MainWindow class will then be called (which we need to write). Let's open up mainwindow.h and add in another header for displaying debug messages:
#include <QDebug>
Then, we declare the slot function, like this:
private: Ui::MainWindow *ui; public slots: void selectionChanged();
After that open mainwindow.cpp and define the slot function, like this:
void MainWindow::selectionChanged() { qDebug() << "Item selected"; }
Now try and run the program again; you should see a line of debug messages that say Item selection which appears whenever a graphics item has been clicked. It's really simple, isn't it?
As for the ItemIsMovable flag, we won't be able to test it using the signal and slot method. This is because all classes inherited from QGraphicsItem class are not inherited from the QObject class, and therefore the signal and slot mechanism doesn't work on these classes. This is intentionally done by Qt developers to make it lightweight, which improves the performance, especially when rendering thousands of items on the screen.
Even though signal and slot is not an option for this, we can still use the event system, which requires an override to the itemChange() virtual function, which I will demonstrate in the next section.