Requests are handled by the Use, Run, Map, and MapWhen extension methods. These methods configure the request delegates.
To understand this in detail, let's create a dummy project using ASP.NET Core. Go through the following steps:
- Open Visual Studio.
- Go to File | New | Project, or click Ctrl + Shift + N. Refer to the following screenshot:
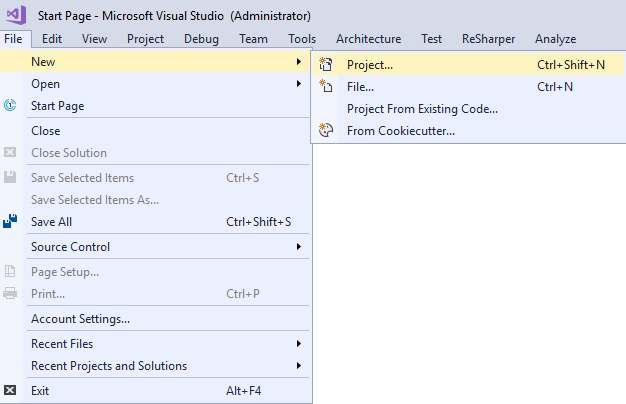
- From the New Project screen, select ASP.NET Core Web Application.
- Name your new project (say Chap05_01), select a location, and click OK, as shown in the following screenshot:
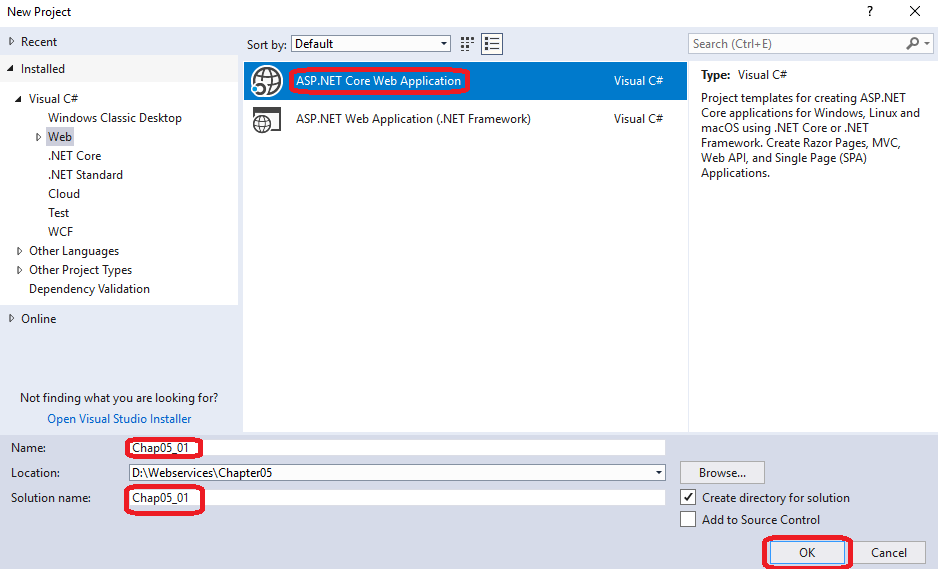
- From the new ASP.NET Core Web Application template screen, choose the API template. Make sure you select .NET Core and ASP.NET Core 2.0.
- Click OK, as shown in the following screenshot:
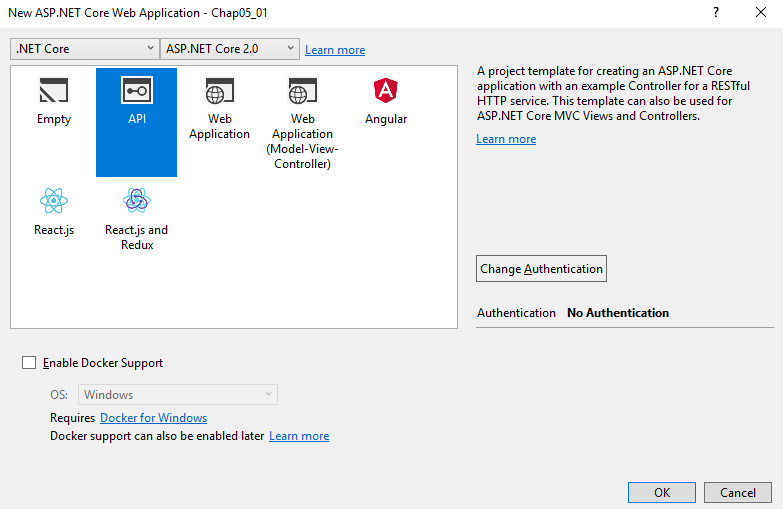
- Open Solution Explorer. You will see the file/folder structure, as shown in the following screenshot:
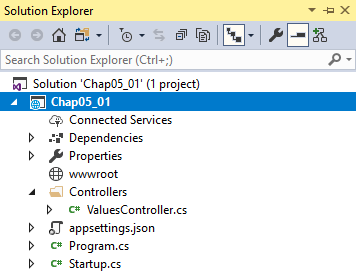
From the dummy project that we have just created, open the Startup.cs file and look at the Configure method, which contains the following code:
// This method gets called by the runtime. Use this method to configure the HTTP request pipeline.
public void Configure(IApplicationBuilder app, IHostingEnvironment env)
{
if (env.IsDevelopment())
{
app.UseDeveloperExceptionPage();
}
app.UseMvc();
}
The preceding code is self-explanatory: it tells the system to add Mvc to the request pipelines by initiating the app.UseMvc() extension method of Microsoft.AspNetCore.Builder.IApplicationBuilder.
It also instructs the system to use a particular exception page if the environment is in development. The preceding method configures the application.
In the next section, we will discuss four important IApplicationBuilder methods in detail.