The Orders table looks a little different to what we sent to the client. In the following screenshot, note the fields marked in boxes. These are the fields we are not sending and will instead manipulate inside the action method:
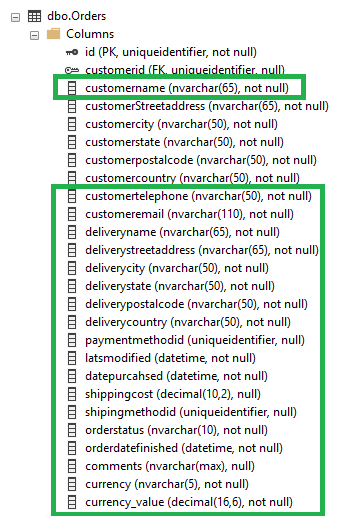
Fields such as name, email, and telephone number can be fetched from the Customers table. Customerid is sent from the client, using which we will fetch these details, as follows:
// POST: api/Orders
[HttpPost]
public async Task<IActionResult> PostOrders([FromBody] Orders orders)
{
if (!ModelState.IsValid)
{
return BadRequest(ModelState);
}
// Retrieve customer details and add to order.
if (orders.Customerid != null)
{
var customer = _context.Customers.SingleOrDefault
(x => x.Id == orders.Customerid);
if (customer != null)
{
orders.Deliveryname = orders.Customername = customer.Firstname;
orders.Customeremail = customer.Email;
orders.Customertelephone = customer.Telephone;
}
}
...
The following code snippet illustrates how a user can copy their billing address so that it is also their delivery address:
// Copy customer address to delivery address.
orders.Deliverycity = orders.Customercity;
orders.Deliverycountry = orders.Customercountry;
orders.Deliverystreetaddress = orders.CustomerStreetaddress;
orders.Deliverypostalcode = orders.Customerpostalcode;
orders.Deliverystate = orders.Customerstate;
Additional fields, including datapurchased, lastmodified, and orderdatefinished, will be set as DateTime.Now. Details such as currency and currency_value will be set as dollars ($) and zero (0). We will also set Guid.NewGuid as shipingmethodid and paymentmethodid.
These can be made inside the Orders constructor as follows:
public Orders()
{
Id = Guid.NewGuid();
OrderProductAttributes = new HashSet<OrderProductAttributes>();
OrdersProducts = new HashSet<OrdersProducts>();
Datepurchased = DateTime.Now;
Lastmodified = DateTime.Now;
Shipingmethodid = Guid.NewGuid();
Paymentmethodid = Guid.NewGuid();
Shippingcost = 0;
Orderdatefinished = DateTime.Now;
Currency = "$";
CurrencyValue = 0;
Orderstatus = "Placed";
}
Notice that the Orderstatus is Placed. This is something that can be updated by the site when an order is ready for shipping. Subsequent statuses might include Approved, Ready, Shipped, Delivered, and so on. If you design the admin screen, make sure you handle this field update along with latsmodified and orderdatefinished.