Inserting data into the cart table can be done by calling the POST action, which should look like the following code block in CartsController:
// POST: api/Carts
[HttpPost]
public async Task<IActionResult> PostCart([FromBody] Cart cart)
{
if (!ModelState.IsValid)
{
return BadRequest(ModelState);
}
_context.Cart.Add(cart);
try
{
await _context.SaveChangesAsync();
}
catch (DbUpdateException)
{
if (CartExists(cart.Id))
{
return new StatusCodeResult(StatusCodes.Status409Conflict);
}
else
{
throw;
}
}
return CreatedAtAction("GetCart", new { id = cart.Id }, cart);
}
The client-side function to call this action can be designed as follows:
function PostCart(customerId, productId, qty, finalPrice)
{
var cart =
{
Customerid: customerId,
Productid: productId,
Qty: qty,
Finalprice: finalPrice
};
$.ajax({
url: 'http://localhost:57571/api/Carts',
type: "POST",
contentType: "application/json",
dataType: "json",
data: JSON.stringify(cart),
success: function (result) {
console.log(result);
},
error: function (message) {
console.log(message.statusText);
}
});
}
Straightforward, isn't it? Now we can build a cart object and send it a POST action. You can try this out by calling the following method inside our AddToCart() function:
// Add one Cart record in Database: POST /api/Carts
PostCart('910D4C2F-B394-4578-8D9C-7CA3FD3266E2',
productId,
cartItem.find('td.qty').html(),
cartItem.find('td.price').html().replace('$', ''))
Here, the first parameter is Customerid, which we have hard-coded. Customerid can be held in session storage for any requests—although this is considered a risky practice.
Let's now run our app and click on Add To Cart for a particular product. Oops! The following error has appeared in the developer tool:
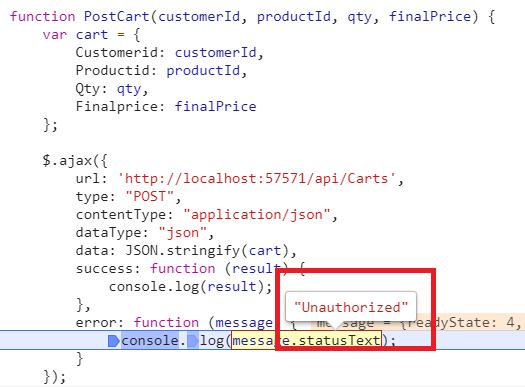
Why has this happened?
The reason for this error is actually pretty obvious. As an [Authorize] attribute has been applied to the controller, every call to CartsController now expects a token generated by a request to the OAuth2.0 Authorize server with Email Id and Password.
To move on with our implementation, we will call the token server from Postman and use that inside our app. Ideally, when you receive an Unauthorized error, you should open the login screen so that the user can log in. If Email Id and Password are validated, a token would be returned. This token can be used for further requests, such as Add To Cart.
To save time and space, we will generate a token using Postman directly by using taditdash@gmail.com as our email ID and 12345 as our password. The subsequent Ajax call with the token should look like the following:
$.ajax({
url: 'http://localhost:57571/api/Carts',
type: "POST",
contentType: "application/json",
dataType: "json",
data: JSON.stringify(cart),
headers: { "Authorization": "Bearer eyJhbGciOiJSUzI1NiIs...
[Long String Removed]" },
success: function (result)
{
var cartItem = $('#tblCart').find('tr[data-product-id=' +
productId + ']');
cartItem.attr('data-cart-id', result.id);
},
});
Note that we have removed the token string in the preceding snippet for brevity. With the previous code, one cart record will be created and data returned from the API will give us all the detail about that record. You can store the cart's Id on the HTML row (as shown inside the preceding code block) for further processing while updating or deleting the cart record.
The following screenshot of the Elements tab in the Chrome Developer Tool illustrates the cart record's ID stored as a data-cart-id attribute:
