With our context in place, and taking care of the communication between our application and the database, let's go ahead and add a repository for facilitating interaction between our data models and our database. Please refer to the code for our repository, as discussed in step 10 of the Creating the project section.
Marking our result from GetAll as IObservable adds the reactive functionality we are looking for. Also, pay special attention to the return statement.
With this observable model, it becomes possible for us to handle streams of asynchronous events with the same ease we are used to when handling other, simpler collections:
return Observable.Return(GetProducts());
We are now ready to expose the functionality through our controllers. Right-click on the folder controller, click on Add New Item, and then select ASP.NET Core, Web API Controller class. Name it ProductController:
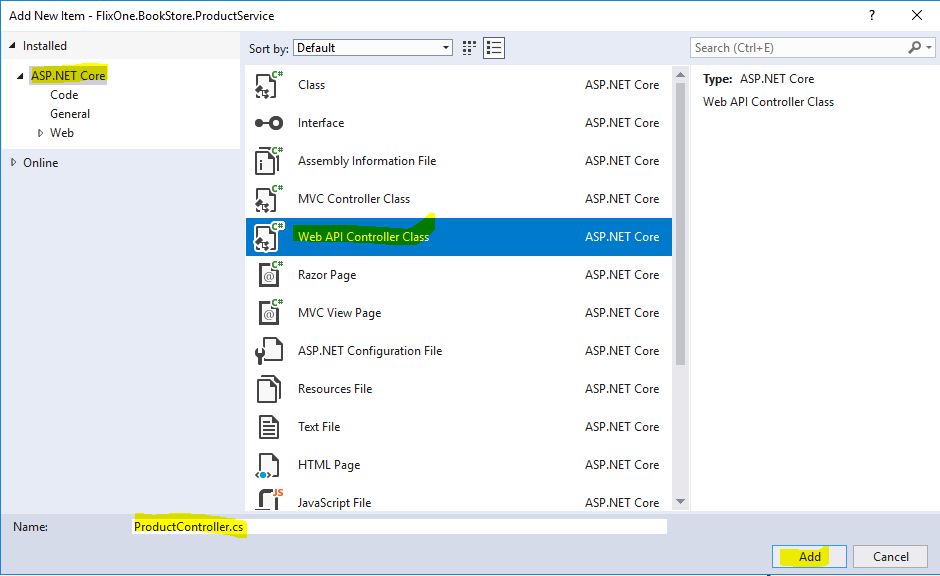
Here is what our controller will look like:
namespace FlixOne.BookStore.ProductService.Controllers
{
[Route("api/[controller]")]
public class ProductController : Controller
{
private readonly IProductRepository _productRepository;
public ProductController() => _productRepository =
new ProductRepository(new ProductContext());
public ProductController(IProductRepository
productRepository) => _productRepository =
productRepository;
[HttpGet]
public async Task<IEnumerable<Product>> Get() =>
await _productRepository.GetAll().SelectMany(p => p).ToArray();
}
}
The final structure looks similar to the following screenshot of the Solution explorer:

To create the database, you can refer to the EF Core migrations section in Chapter 2, Implementing Microservices, or simply call the Get API of our newly deployed service. When the service finds out that the database doesn't exist, the entity framework core code-first approach, in this case, will ensure that the database is created.
We can now go ahead and deploy this service to our client. With our reactive microservice deployed, we now need a client to call it.