Spring was initially started as an alternative to more heavy approaches to enterprise applications such as the J2EE standard. It made it possible to cleanly separate the framework from the code by allowing the configuration of POJOs (Plain Old Java Objects) rather than forcing classes to extend a certain class or implement an interface.
Spring grew and evolved over time and is the most popular Java framework for building applications today.
Core Spring
Core Spring includes Spring’s Dependency Injection (DI) framework and configuration. The DI design pattern is a way to externalize the details of a dependency by allowing them to be injected. This, coupled with the use of interfaces, allows you to decouple code and makes software more manageable and extensible. DI is a subset of Inversion of Control (IoC) , where the flow of an application is reversed or inverted.
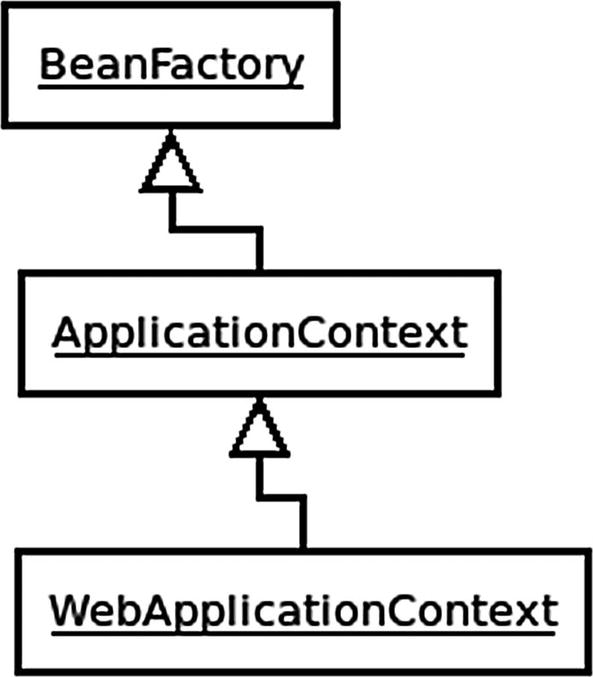
Simplified class diagram for WebApplicationContext
An ApplicationContext provides Bean factory methods for accessing application components (inherited from the ListableBeanFactory interface), the ability to load file resources in a generic fashion, the ability to publish events to registered listeners (inherited from the ApplicationEventPublisher interface), the ability to resolve messages supporting internationalization (inherited from the MessageSource interface), and possible inheritance from a parent ApplicationContext. ApplicationContext has many different subclasses, one of which is WebApplicationContext, which, as the same suggests, is useful for web applications.
Beans from POJOs can be configured in one of three ways: XML, methods annotated with @Bean in a configuration Java class annotated with @Configuration, or when using component scanning, you can add an annotation such as @Component or @Service on the POJO class itself. The most recommended way is using one or more Java configuration classes for infrastructure and component scanning for business classes.
Spring Modules
Aspect-oriented programming (AOP) – Enables implementing cross-cutting concerns through runtime code interweaving.
Spring Security – Authentication and authorization, configurable security that supports a range of standards, protocols, tools, and practices.
Spring Data – Templates and tools for working with relational database management systems on the Java platform using Java Database Connectivity (JDBC), Object-Relational Mapping (ORM) tools, Reactive Relational Database Connectivity (R2DBC), and NoSQL databases.
Core – Inversion of Control container, configuration of application components, and life-cycle management of Beans.
Messaging – Registration of message listener objects for transparent message-consumption and sending to/from message queues via multiple transport layers including Java Message Service (JMS), AMQP, Kafka, and many others.
Spring MVC (Model-View-Controller) – An HTTP and servlet-based framework providing hooks for extension and customization for web applications and RESTful (Representational State Transfer) web services.
Transaction management – Unifies several transaction management APIs and coordinates transactions supporting JTA and JXA.
Testing – Supports classes for writing both unit and integration tests such as Spring MVC Test which supports testing the controllers of Spring MVC applications.
Spring Boot – Convention over configuration framework for simplifying application development. It includes auto-configuration and has “starter” dependencies that include many open source dependencies and the compatible versions of each dependency.
Spring WebFlux – A reactive web framework using the reactive stream specification that can run on Netty, Tomcat, or Jetty (using Servlet 3.0 asynchronous threading).

Spring modules