This chapter focuses on the building blocks that are necessary to become a great Swift programmer. This chapter covers how to use the playground user interface, how to write your first Swift program, and how to use the Xcode Integrated Development Environment (IDE).
Note
We will introduce you to using playgrounds, which will enable you to program right away without worrying about all of the complexities of Xcode projects. We use this approach to help you learn the concepts quickly, without discouragement, and to give you a great foundation to build upon.
Touring Xcode
Design or modify an algorithm, observing the results every step of the way
Create new tests, verifying that they work before promoting them into your test suite
First, you’ll need to learn a little more about the Xcode user interface. When you open an Xcode iOS project, you are presented with a screen that looks like Figure 2-1.

Xcode Integrated Developer Environment with a Swift project
Exploring the Workspace Window
The workspace window, shown in Figure 2-2, enables you to open and close files, set your application preferences, develop and edit an app, and view the text output and error console.

Xcode’s workspace window
The workspace window has four main areas: Editor, Navigator, Debug, and Utility.
When you select a project file, its contents appear in the Editor area, where Xcode opens the file in the appropriate editor.
Clicking this button shows or hides the Navigator area. This is where you view and maneuver through files and other facets of your project.
Clicking this button shows or hides the Debug area. This is where you control program execution and debug code.
Clicking this button shows or hides the Utility area. You use the Utility area for several purposes, most commonly to view and modify attributes of a file.
Navigating Your Workspace
You can access files, symbols, unit tests, diagnostics, and other features of your project from the Navigator area. In the navigator selector bar, you choose the navigator suited to your task. The content area of each navigator gives you access to relevant portions of your project, and each navigator’s filter bar allows you to restrict the content that is displayed.
Project navigator: Add, delete, group, and otherwise manage files in your project; or choose a file to view or edit its contents in the editor area.
Source Control navigator: View the detailed history of changes you have made to your project files when using a Version Control System (VCS) like Git.
Symbol navigator: Browse the class hierarchy in your project.
Find navigator: Use search options and filters to quickly find text within your project.
Issue navigator: View issues such as diagnostics, warnings, and errors found when opening, analyzing, and building your project.
Test navigator: Create, manage, run, and review unit tests.
Debug navigator: Examine the running threads and associated stack information at a specified point of time during program execution.
Breakpoint navigator: Fine-tune breakpoints by specifying characteristics such as triggering conditions and see all your project’s breakpoints in one place
Report navigator: View the history of your builds.
Editing Your Project Files
Source editor: Write and edit Swift source code.
Interface builder: Graphically create and edit user interface files
(see Figure 2-3).
Project editor: View and edit how your apps should be built, such as by specifying build options, target architectures, and app entitlements.
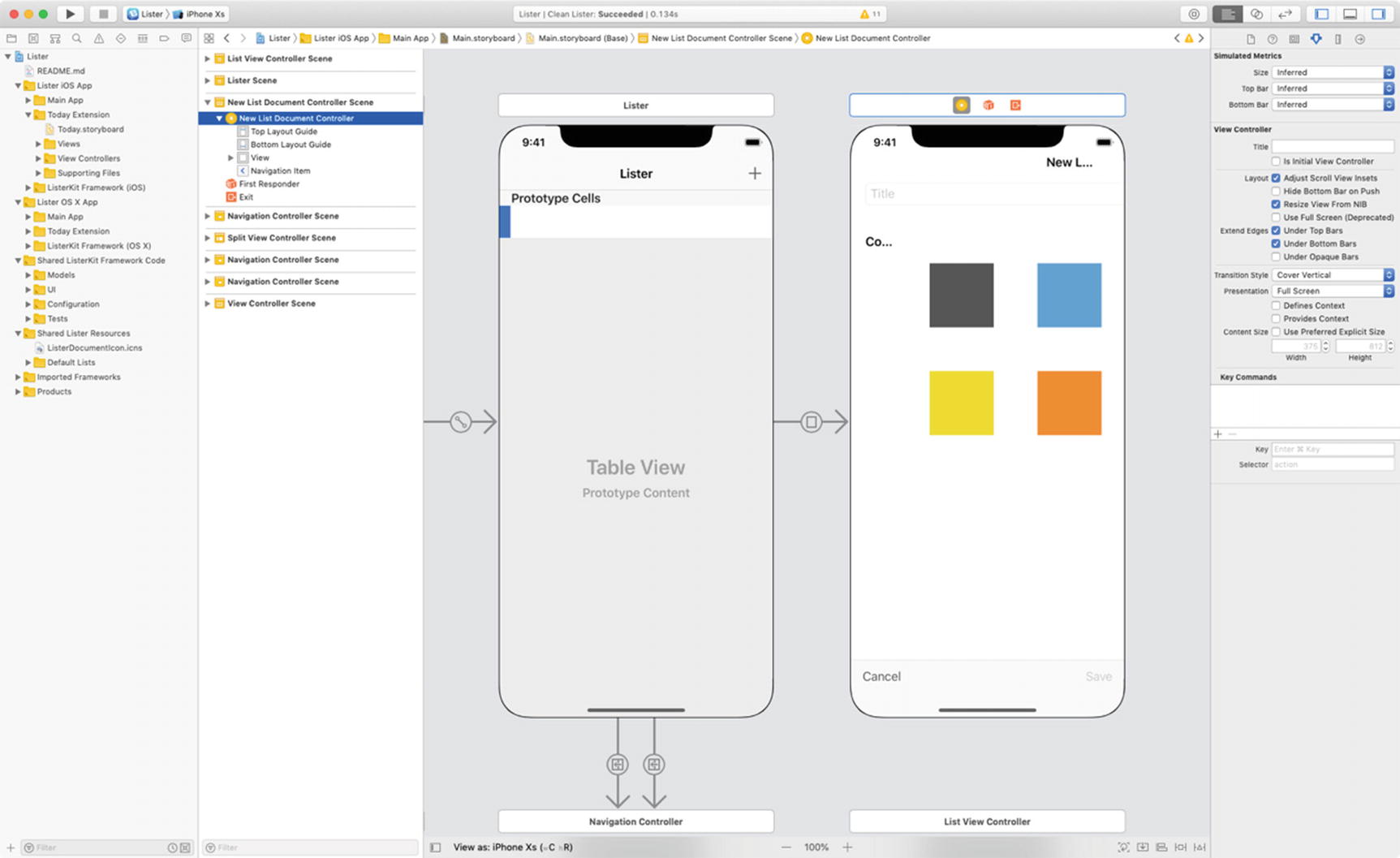
Xcode’s Interface Builder showing a storyboard file
Clicking this button opens the Standard editor. You will see a single editor pane with the contents of the selected file.
Clicking this button opens the Assistant editor. You will see a separate editor pane with content logically related to that in the Standard editor pane.
Clicking this button opens the Version editor. You will see the differences between the selected file in one pane and another version of that same file in a second pane. Used when working with source control.
Creating Your First Swift Playground Program
Now that you have learned a little about Xcode, it’s time to write your first Swift playground program and begin to understand the Swift language, Xcode, and some syntax. First, you have to install Xcode.
Installing and Launching Xcode 10.2
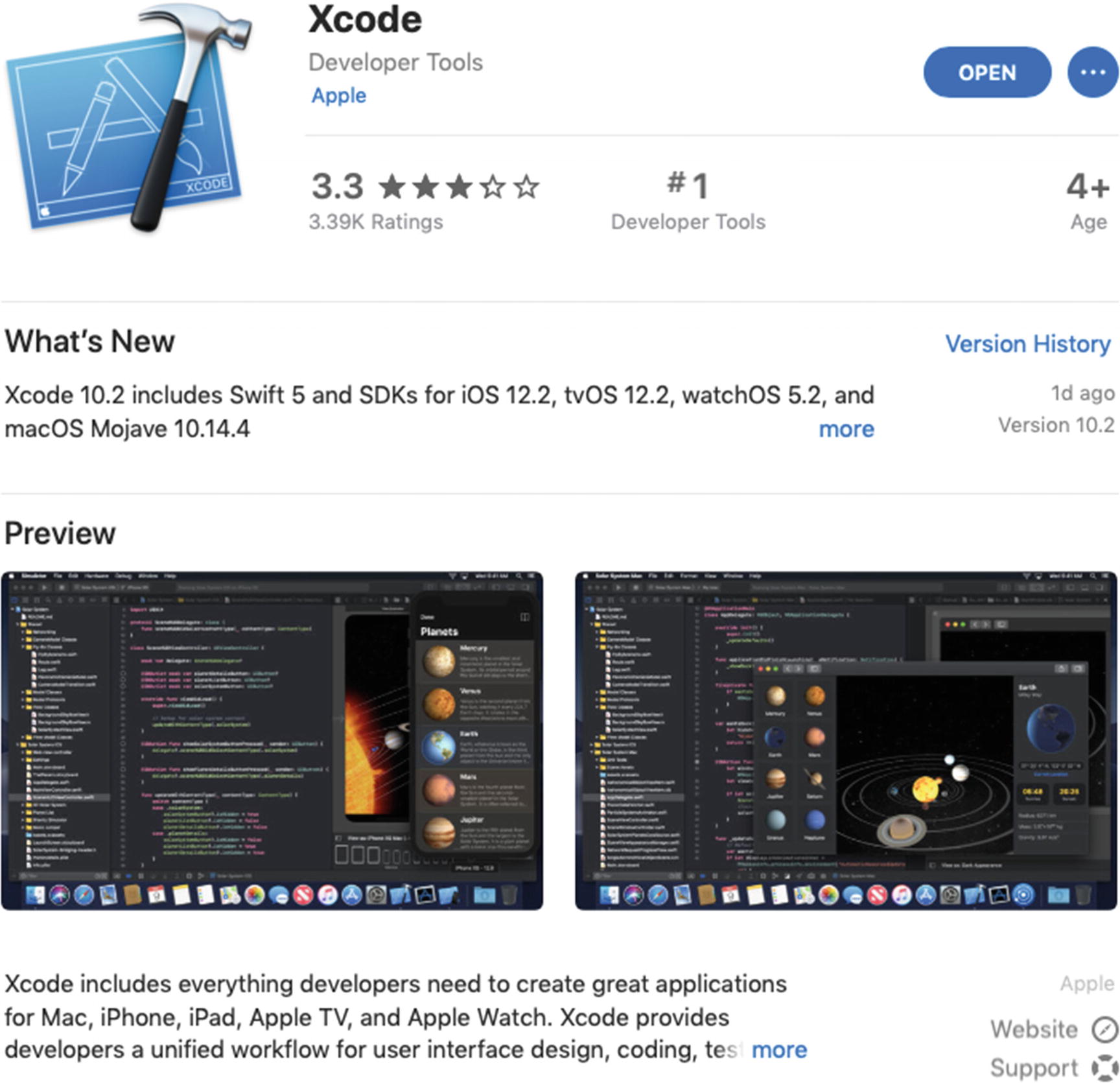
Xcode 10.2 is available for download from the Mac App Store for free
Note
This package has everything you need to write iOS, watchOS, tvOS, and macOS apps. To publish apps on the iOS or macOS App Stores, you will need to apply for the Apple Developer Program and pay $99 when you’re ready to submit. Figure 2-5 shows the Apple Developer Program web site at https://developer.apple.com/ .
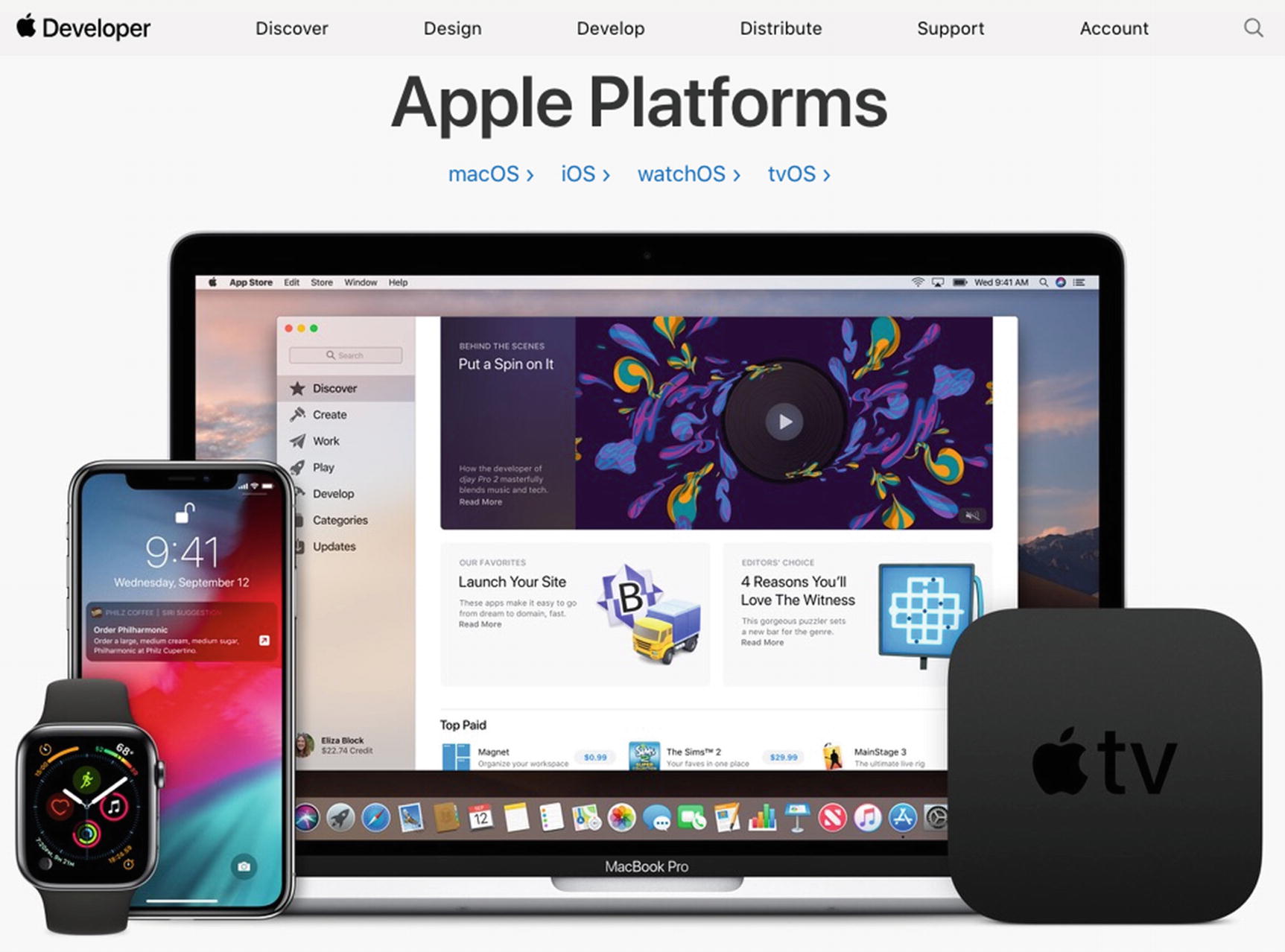
The Apple Developer Program
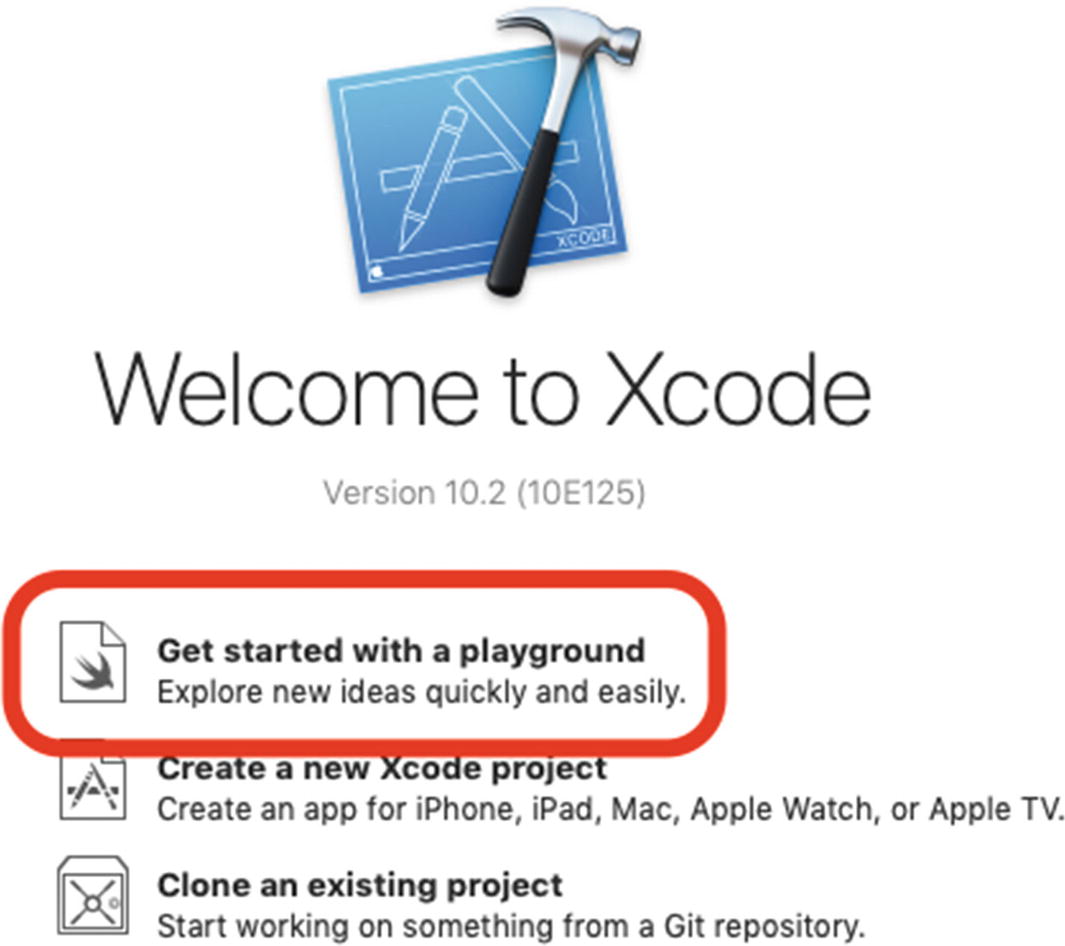
Creating your first Swift playground
Using Xcode 10.2
- 1.
Select a Blank iOS template and click Next, as shown in Figure 2-7.
- 2.
Name the playground HelloWorld and create it in a folder of your choice, like Documents or the Desktop.
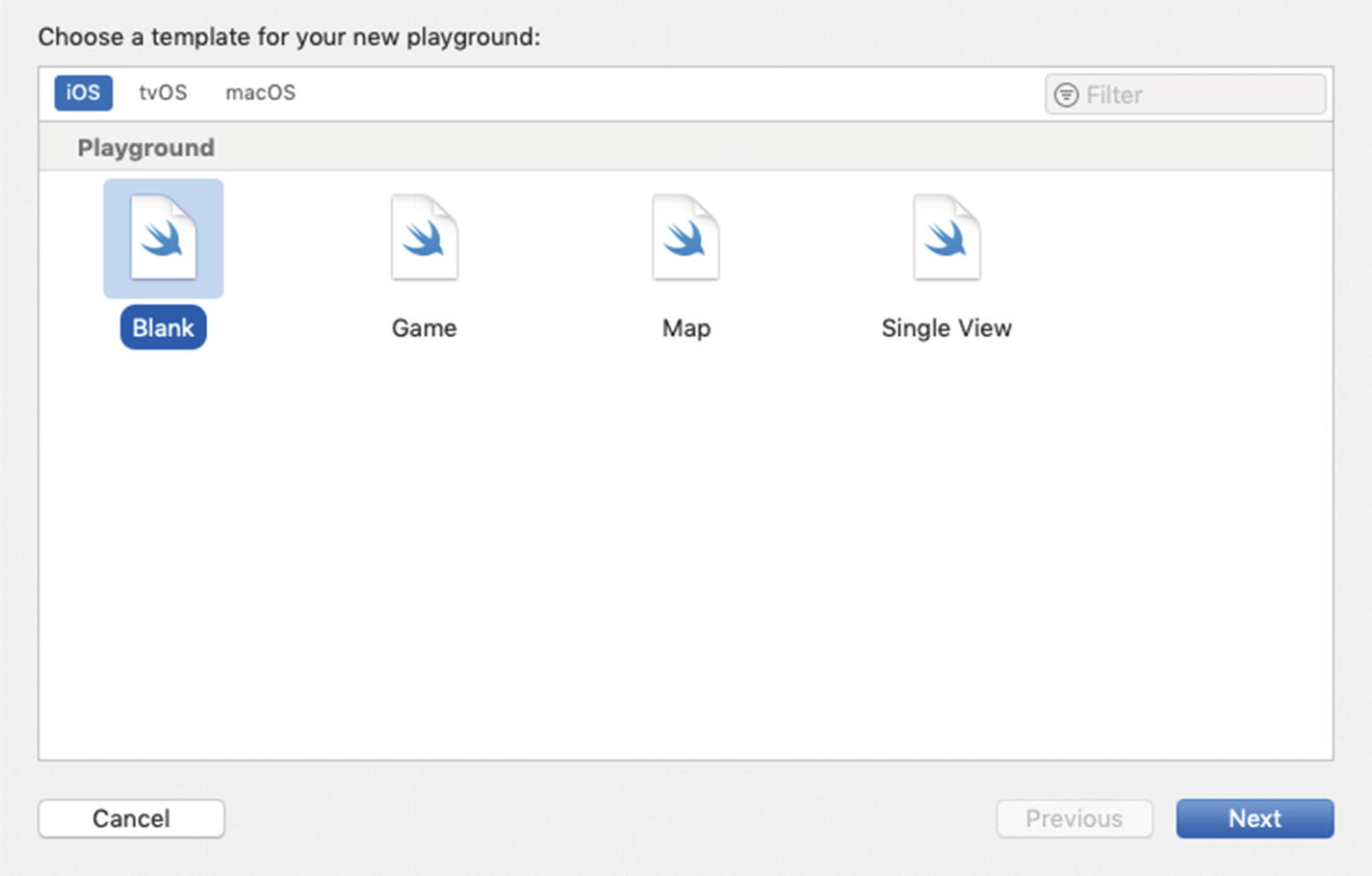
Choosing a Blank iOS playground template
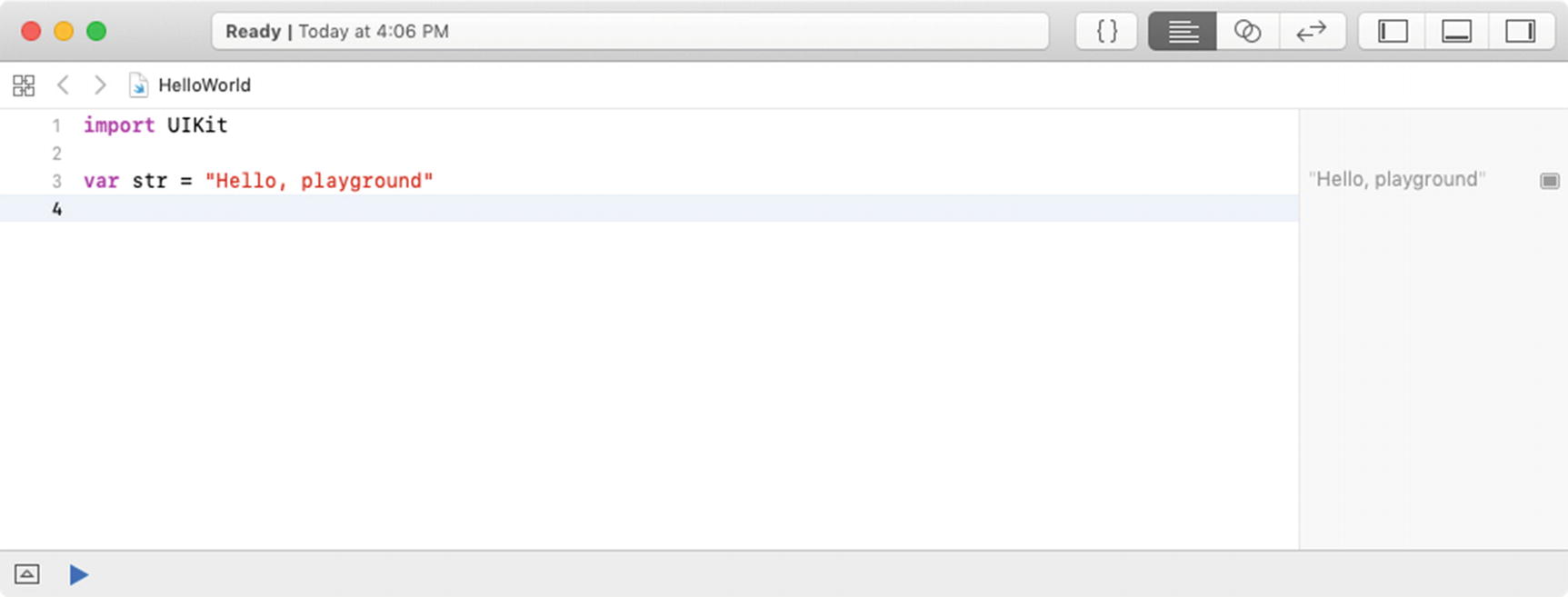
The playground window
The Editor area
The Results area
Xcode Playground IDE: Editor and Results Areas
The Editor area is the business end of the Xcode playground IDE—where your dreams are turned into reality. It is where you write your code. As you write your code, you will notice it changes color. Sometimes, Xcode will even try to autocomplete words for you. The colors have meanings that will become apparent as you use the IDE. The Editor area is also where you will debug your code.
Note
Even if we’ve mentioned it already, it is worth saying again: You will learn Swift programming by reading this book, but you will really learn Swift by writing and debugging your code. Debugging is where developers learn and become great developers.
As soon as you enter the line of code, Xcode automatically executes the line and shows the result, “Hello, playground\n”.
When you write Swift code, everything is important—commas, capitalization, and parentheses. The collection of rules that enable the compiler to compile your code to an executable app is called syntax.
Line 3 creates a string variable called str and assigns “Hello, playground” to the variable.
Line 4 prints the str string variable to the Results Area.
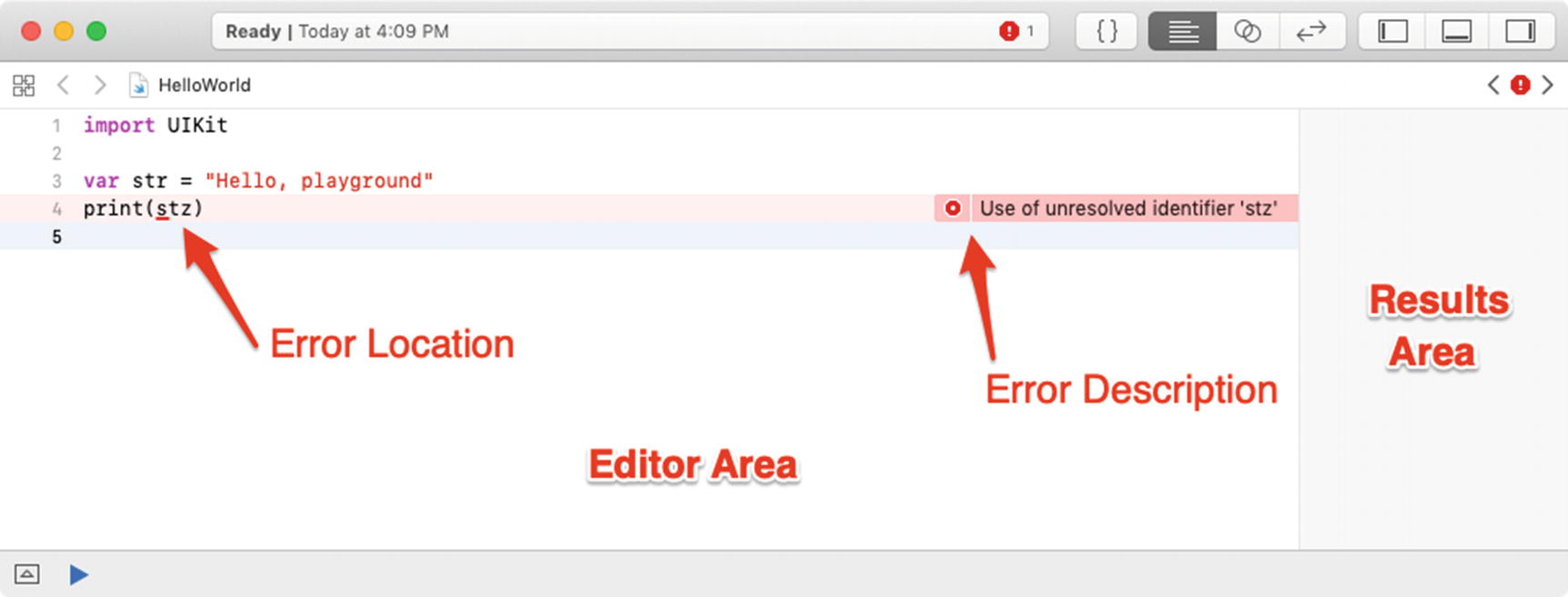
The playground with a syntax error caught by the Swift compiler
In Swift, print is a function that will print the contents of its parameters in the Results area. As you enter code, the Results area automatically updates with the results for each line of code that you entered.
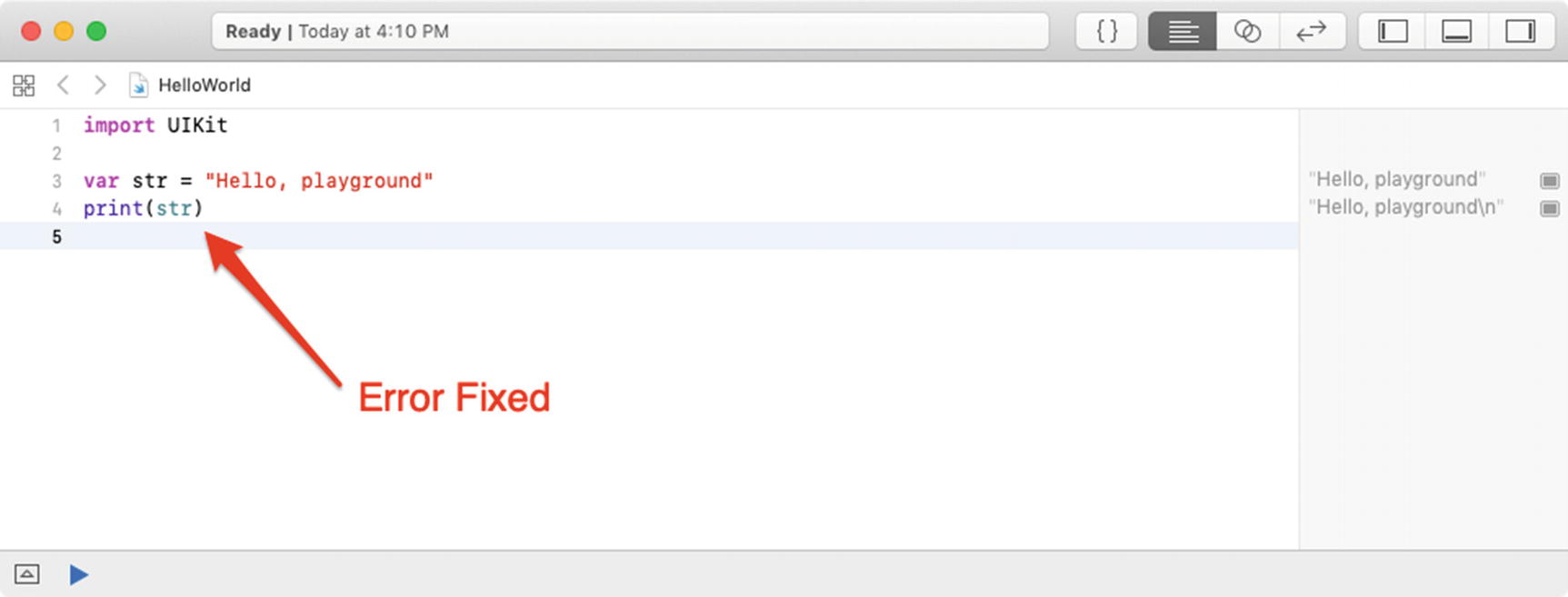
Syntax error fixed
Feel free to play around and change the text that is printed. You may want to add multiple variables or add two strings together. Have fun!
Summary
In this chapter, you built your first basic Swift playground. We also covered new Xcode terms that are key to your understanding of Swift.
Playground
Editor area
Results area
Exercise
Extend your playground by adding a line of code that prints any text of your choosing.