Image resizing is the process of changing an image's size without keeping proportions. This is done by calling the imresize function and supplying a new width and height.
Let's take our image with the cats as an example and resize it to 100 x 250 so that we can see what has changed:

We will use our classic code and load the image from disk:
using Images, ImageView
source_image = load("sample-images/cats-3061372_640.jpg");
resized_image = imresize(source_image, (100, 250));
imshow(resized_image);
You should be able to see an image of a smaller size. It has a width of 250 pixels and a height of 100 pixels:
A typical example would be to resize an image to fit a square. You would need to pass the width and height as equal values:
using Images, ImageView
source_image = load("sample-images/cats-3061372_640.jpg");
resized_image = imresize(source_image, (200, 200));
imshow(resized_image);
This would result in an image like this:
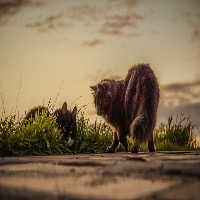