We will be implementing an image sharpening technique used by modern graphical editors, such as GIMP and Photoshop. This suggests that we apply the Gaussian smoothing filter to a copy of an image and subtract the smoothed version from the original image (in a weighted way so that the values of a constant area remain constant).
Let's try to apply sharpening to our image of cats and see the change:
using Images, ImageView
gaussian_smoothing = 1
intensity = 1
# load an image and apply Gaussian smoothing filter
img = load("sample-images/cats-3061372_640.jpg");
imgb = imfilter(img, Kernel.gaussian(gaussian_smoothing));
# convert images to Float to perform mathematical operations
img_array = Float16.(channelview(img));
imgb_array = Float16.(channelview(imgb));
# create a sharpened version of our image and fix values from 0 to 1
sharpened = img_array .* (1 + intensity) .+ imgb_array .* (-intensity);
sharpened = max.(sharpened, 0);
sharpened = min.(sharpened, 1);
imshow(sharpened)
# optional part: comparison
img[:, 1:321] = img[:, 320:640];
img[:, 320:640] = colorview(RGB, sharpened)[:, 320:640];
imshow(img)
The resulting image is as follows:
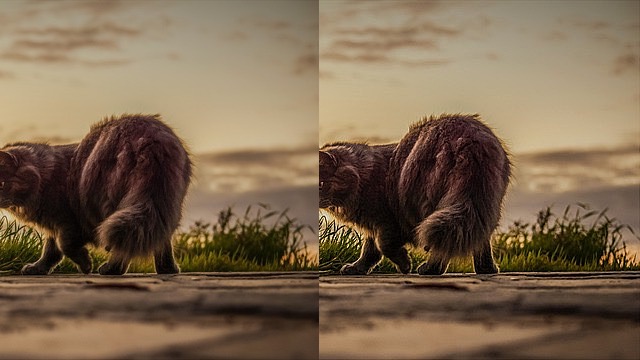
Changing gaussian_smoothing to 2 makes it even more sharpened:

Feel free to play around with the gaussian_smoothing and intensity parameters to achieve the required outcome. gaussian_smoothing is recommended to be set from 1 to 4 and the intensity from 0.1 to 5.