In the following section, we will learn to separate a foreground object, which is a cat, from the rest of the background. For this, consider the following code:
using Images, ImageView, ImageSegmentation
img = load("sample-images/cat-3352842_640.jpg");
imshow(img)
Let's start by loading this image into Julia and previewing it:

Julia implements the seeded_region_growing function to achieve this task. The prerequisites for using the seeded_region_growing function are the following:
- An image
- A set of points specifying each region
The seeded_region_growing function requires us to supply the coordinates of the cat manually. The easiest option would be to hover the cursor over the cat and note down the coordinates from the bottom-left corner of the preview window, such as [220, 250]. We also need a value for the grass, such as [220, 500].
The ImageSegmentation package requires us to define all segments as an array of tuples of CartesianIndex along with a segment number. Consider the following code:
seeds = [
(CartesianIndex(220,250), 1),
(CartesianIndex(220,500), 2)
]
The moment seeds are defined, we will be calling the seeded_region_growing function, which assigns every pixel to a segment as follows:
segments = seeded_region_growing(img, seeds)
Now, we will use the map function to replace the segment number of each pixel with a mean color value of a segment:
imshow(map(i->segment_mean(segments,i), labels_map(segments)))
This results in an image consisting of two colors, with green representing grass and the other representing the cat. Because the image is relatively simple and the cat can be easily separated, the result is of a very high quality. This is shown in the following image:
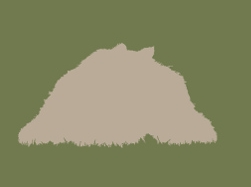
Despite the fact that this approach involves manual work, it can be handy when preparing training datasets for image segmentation tasks executed by neural networks.
The image of the cat was taken from https://pixabay.com/en/cat-british-longhair-breed-cat-3352842/.