Rust for the IoT, Building Internet of Things Apps with Rust and Raspberry Pi
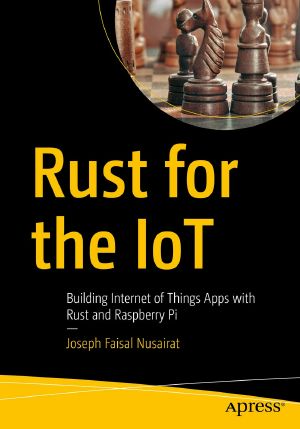
- Authors
- Nusairat, Joseph Faisal
- Publisher
- Apress
- Date
- 2020-09-15T00:00:00+00:00
- Size
- 16.60 MB
- Lang
- en
Get started programming Rust applications for the Internet of Things (IoT). This book is a programming skills migration book that teaches you the Rust programming techniques most useful for IoT applications.You'll step through from server to board development in creating a set of IoT applications.
In Rust for the IoT , you'll learn how to build amodern server-side applicationusing Rust on the backend. Then you'll use docker and Kubernetes to deploy these to a managed cloud.Finally you will use a Raspberry Pi with a SenseHat and Camera to capture the world around you and send that information to the cloud.
While you will be able to follow along without any cloud or hardware, to make the most of it we recommend a few cloud pieces and hardware that is designed to integrate with the software in this book. After reading and using this book, you'll see how to apply Rust to the Internet of Things.
What You Will Learn
Create a modern Rust backend complete with handling eventual consistency and interacting via a GraphQL interface Use the Raspberry PI to serve as a cheap IoT device that one can easily deploy around the house Capture temperature, video, and use the interactive joystick to interact with the software you’ve created Use OpenCV to perform facial detection from the PI’s camera and save that information to the cloud. Create deployable helm charts for the cloud, and for the device create complete ISOs that allow you to easily deploy the Pi’s OS + custom software
Who This Book Is For
You will need to have a basic understanding of cloud application development at a minimum and the basics of Rust coding. This book is for those interested in or working with the IoT and the Raspberry Pi who want to learn how Rust can work for them.
04
02
1. IntroductionIn an age of home automation and internet of all things (IoT), the IoT device market has blown up. Interoperability is still missing and creating and customizing is not only a fast market but a fast pursuit for hobbyists. This book will step you through creating your own IoT from web software and transforming to the hardware. We shall create an IoT device configurable to do the following:
- access and configure via the web
- use voice commands via Alexa to interact with our IoT endpoints
- record video from RasberryPie Cameras
- record video from Nest Camera
- store video to local RasberryPi and the cloud.
- write a display to the Raspberry Pi Device
2 The Server Side
Our first set of chapters will deal with setting up the server side itself. Getting the application up and running to store video content and stream it out to clients.
3, Parsers
We will start to go over how the Serde parser works, this is not only a useful parser to quickly parse the amounts of JSON data we will be getting but is also used under the hood by Iron (our web framework) for processing.
4. Web Site
Initially, we will build the web site to configure our IoT application, creating our Restful interfaces to it. This will be written in Iron that uses hypers futures under the cover to allow many simultaneous connections that would be needed for applying IoT at scale. This will expose interfaces to not only query but to also save data to the S3 buckets.
5. CQRS Site
Building upon the previous chapter we will use CQRS in creating dynamic queries to the site.
6. Serverless
Finally, the serverless architecture model will be used to run once a day to help clean up the S3 data. Since we want a cap on how much video data is stored this will remove enough old data to allow more to be stored.
7. Data Pipelines
The previous chapters created web interfaces that allow us to store by web or rest/ec calls to store vast amounts of data. This chapter will create ETLs in Rust that allows you to process the data for tagging, loading, and unloading. In here the users will be shown how to use the data store to process large extractions and transformations. Most of this will do with video data that has been tagged and to be able to get requests of the video data.
8. Board Application Development
This section is where we first start to interact with the board and have our board interact with the server in a traditional IoT/Server design. We will go over what you need to assemble and start up the board as well as configuring it to run a rust binary. As well as start features of the board.
9. Board Development
For this application, we will be running a RasberryPi and NixM8 board that we will be programming on. This chapter will run through the basics of setting up the board to run a Rust application and perform system IOs as well as Web IOS. The board will access the website we created earlier to retrieve data and return it to a terminal that it is running on via the logs.
10. Command Lines
Command line integration will be used to create local tools to test our board development work. We can use these to interact with the boards and test our interaction with the application. These will be the entry point into our feature set for the board.
11. Video Display
One of the first things we will go over is video display for the board. This will initially allow us a display to show what is stored on the remote servers for video playback, and will playback the video to the attached device. In addition, you will be able to stream the content to another Raspberry Pi device that acts as a client.
12. Camera Capture
We have gone over how to stream and show video that is stored in the cloud, in this chapter we will attach a camera to one of our devices and start capturing the data for storage. We will also go over how to capture camera data to store to a storage repository as well as pushing that same data to the cloud. [possibly broken up into 2 chpaters]
13. 3rd Party Integration
You now have the board created and working against the application, these few chapters will explain how we can leverage Rust to integrate with existing or 3rd party architecture that we may want to use.
14. Alexa Integration
First up is Alexa, this makes the most sense since this is why you purchased an Alexa board for this. In addition, this will give us a way to interact with the board without having to use the command line interfaces, since in real life you don't want to do that. This chapter will cover creating Alexa commands to interact with the video data and information on our cloud status and size.
We will also be using Alexa integration as our primary choice from here on out.
15. Intercepting Camera Data From a 3rd Party
While capturing data from our camera is nice, in the real world people often have different cameras they already bought, and if you are doing this as a home project you will most likely use a 3rd party camera. This chapter we will go over how to capture data from Nest cams ad well as the Ring doorbell camera. This allows us to have an ecosystem not tied to any one device but gives us a one size storage all. In addition, this will allow us not pay for as much cloud storage since we can store it to our local server and then keep a small segment up in the cloud.
16. Integrating with Devices
One of the two most common communication methods is devices with Zwave and Zigbee. In this, we will show how to configure the app to add some devices and use Alexa to communicate not only status but to activate/deactivate them etc.
17. Command Line Deployers
Finally, now that we have the app and all our coding complete we will go over how to make deployers for the board so that if you wanted to deploy this as the code to sell or others to use that have little technical knowledge they could.
13
02
Joseph Faisal Nusairat is aa senior partner at Integrallis Software and is asoftware developer who has been working full-time in the Columbus, Ohio, area since 1998, primarily focused on Java and most recently Rust application development. His career has taken him into a variety of Fortune 500 industries, including military applications, data centers, banking, Internet security, pharmaceuticals, and insurance. Throughout this experience, he has worked on all varieties of application development from design to architecture to development. Joseph, like most Java developers, is particularly fond of open source projects and tries to use as much open source software as possible when working with clients. Joseph is a graduate of Ohio University with dual degrees in computer science and microbiology and a minor in chemistry. While at Ohio University, Joseph also dabbled in student politics and was a research assistant in the virology labs.
18
02
Get started programming Rust applications for the Internet of Things (IoT). This book is a programming skills migration book that teaches you the Rust programming techniques most useful for IoT applications.You'll step through from server to board development in creating a set of IoT applications.
In Rust for the IoT , you'll learn how to build amodern server side applicationusing Rust on the backend. Then you'll use docker and Kubernetes to deploy these to a managed cloud.Finally you will use a Raspberry Pi with a SenseHat and Camera to capture the world around you and send that information to the cloud.
While you will be able to follow along without any cloud or hardware, to make the most of it we recommend a few cloud pieces and hardware that is designed to integrate with the software in this book. After reading and using this book, you'll see how to apply Rust to the Internet of Things.
You will:
Create a modern Rust backend complete with handling eventual consistency and interacting via a GraphQL interface Use the Raspberry PI to serve as a cheap IoT device that one can easily deploy around the house Capture temperature, video, and use the interactive joystick to interact with the software you’ve created Use OpenCV to perform facial detection from the PI’s camera and save that information to the cloud. Create deployable helm charts for the cloud, and for the device create complete ISOs that allow you to easily deploy the Pi’s OS + custom software
19
02
The first book on learning Rust for the Internet of Things
Includes many code-laden examples along the way
Covers all aspects of getting your code running
06
05
300
01
https://covers.springernature.com/boo...
01
01
https://www.springer.com/9781484258590
01
http://www.springer.com/
01
Springer Nature Imprint
APR
Apress
01
01
APR
Apress
01
05
5235327
Apress
Berkeley, CA
US
02
20200829
2020
01
WORLD
08
0
gr
01
254
mm
02
178
mm
27
03
9781484258606
15
9781484258606
01
ISBN-13 hyphenated
978-1-4842-5860-6
DG
Apress
01
ROW
NP
10
20200829
02
Recommended Retail Price
01
BIC discount group code
ASPVBT
02
Product discount group
SPVBT
01
59.99
AUD
AU
20190220
01
Recommended Retail Price
01
BIC discount group code
ASPVBT
02
Product discount group
SPVBT
01
54.54
AUD
AU
20190220
02
Recommended Retail Price
01
BIC discount group code
ASPVBT
02
Product discount group
SPVBT
01
41.50
CHF
CH
R
2.5
20190220
02
Recommended Retail Price
01
BIC discount group code
ASPVBT
02
Product discount group
SPVBT
01
37.44
EUR
DE
R
7
20190220
02
Recommended Retail Price
01
BIC discount group code
ASPVBT
02
Product discount group
SPVBT
01
36.91
EUR
FR
20190220
02
Recommended Retail Price
01
BIC discount group code
ASPVBT
02
Product discount group
SPVBT
01
36.39
EUR
IT
20190220
02
Recommended Retail Price
01
BIC discount group code
ASPVBT
02
Product discount group
SPVBT
01
38.14
EUR
NL
20190220
02
Recommended Retail Price
01
BIC discount group code
ASPVBT
02
Product discount group
SPVBT
01
38.49
EUR
AT
R
10
20190220
01
Recommended Retail Price
01
BIC discount group code
ASPVBT
02
Product discount group
SPVBT
01
34.99
EUR
ROW
20190220
01
Recommended Retail Price
01
BIC discount group code
ASPVBT
02
Product discount group
SPVBT
01
29.99
GBP
GB
20190220
01
Recommended Retail Price
01
BIC discount group code
ASPVBT
02
Product discount group
SPVBT
01
2500.00
INR
IN
20190220
Apress
01
US
02
Y
NP
10
20200829
01
Recommended Retail Price
01
BIC discount group code
ADGNY14
02
Product discount group
DGNY14
01
34.99
EUR
ROW
20190220
01
Recommended Retail Price
01
BIC discount group code
ADGNY14
02
Product discount group
DGNY14
01
39.99
USD
US
20190220