This chapter will focus on working with JavaScript arrays. As a JavaScript developer, you will use the array often; it is the most commonly used data structure. Arrays in JavaScript come with a lot of built-in methods. In fact, there are various ways to do the same type of array operations for each use case. By the end of this chapter, you will understand how to work with arrays and be able to choose the right method for the situation.
Introducing Arrays
For any data structure, developers are interested in time and space complexity associated with the four fundamental operations: access, insertion, deletion, and search. (For a review of Big-O notations, please refer to Chapter 1.)
Insertion
The time complexity of this operation is O(1) in theory. It should be noted that, practically, this depends on the JavaScript engine that runs the code. This applies to all natively supported JavaScript objects.
Deletion
The time complexity of .pop is O(1) similarly to .push.
Access
Iteration
Iteration is the process of accessing each of the items contained within a data structure. There are multiple ways to iterate through an array in JavaScript. They all have a time complexity of O(n) since the iteration is visiting n number of elements.
for (Variables; Condition; Modification)
for ( in )
This prints the following: 0,1,2,3.
This printsall, cows, are, and big.
for ( of )
This prints out all, cows, are, and big.
forEach( )
Both print all, cows, are, and big.
Helper Functions
The following sections discuss other commonly used helper functions for processing. In addition, working with arrays will be covered.
.slice(begin,end)
.from() takes O(n), where n is the size of the array. This is intuitive because copying the array requires copying all n elements of the array.
.splice(begin,size,element1,element2…)
This helper function returns and changes the contents of an array by removing existing elements and/or adding new elements.
.splice() is, worst case, O(n). Similarly to copying, if the range specified is the whole array, each n item has to be removed.
.concat()
.length Property
Spread Operator
Both the Math.max and Math.min functions take an unlimited number of parameters, so you can use the spread operator for the following operations.
Exercises
All the code for the exercises can be found on GitHub.1
Find Two Array Elements in an Array That Add Up to a Number
Problem: Given the array arr, find and return two indices of the array that add up to weight or return -1 if there is no combination that adds up to weight.
For example, in an array like [1,2,3,4,5], what numbers add up to 9?
The answers are 4 and 5, of course.
This solution iterates through an array looking to see whether a matching pair exists.
Two for loops over n elements of the array yields a high time complexity. However, no extra memory was created. Similar to how time complexity describes the time required relative to input size, n, to finish the algorithm, the space complexity describes the additional memory needed for implementation. The space complexity, O(1), is constant.
Time Complexity: O(n2)
Space Complexity: O(1)
Let’s think about how to do this in linear time of O(n).
What if any previously seen array elements were stored and could be checked easily?
Here, 4 and 5 are the combination, and their indices are [3,4]. How could it be determined that a solution exists when 5 is visited?
Time Complexity: O(n)
Space Complexity: O(n)
Storing into a hash table and looking an item up from a hash table is only O(1). Space complexity has increased to O(n) to store the visited array indices inside the hash table.
Implement the Array.Slice() Function from Scratch
Let’s review what the .slice() function does.
Time Complexity: O(n)
Space Complexity : O(n)
The time complexity is O(n) because all n items in the array must be accessed. Space complexity is also O(n) to hold all n items when copying the array.
Find the Median of Two Sorted Arrays of the Same Size
Recall that median in an even number of a set is the average of the two middle numbers. If the array is sorted, this is simple.
Here’s an example:
Now, you can iterate through both of the arrays and compare which is bigger to track the median. If the two arrays are the same size, the total size will be an even number.
This is because both two even numbers and two odd numbers add up to an even number. Please refer to Chapter 8 for more background.
Since both of the arrays are sorted, this function can be recursively called. Each time, it checks which median is greater.
If the second array’s median is greater, the first array is cut in half, and only the higher half is passed recursively.
If the first array’s median is greater, the second array is cut in half, and only the higher half is passed in as the first array for the next function call because the array2 parameter in the function must always be bigger than the array1 parameter. Finally, the size of the array represented as pos is required to check whether the size of the array is even or odd.
Here’s another example:
array1 = [1,2,3] and array2 = [4, 5, 6]
Here, the median of array1 is 2, and the median of array2 is 5. So, the median must be present within [2,3] and [4,5]. Since there are only four elements left, the median can be computed as follows:
Time Complexity: O(log2(n))
By cutting the array size by half each time, logarithmic time complexity is achieved.
Find Common Elements in K-Sorted Arrays
In this example with three arrays, k=3.
To do this, simply iterate over each array and count instances of every element. However, do not track repeated ones (5 and 5.5 should be counted once in one array iteration). To do this, check whether the last element is the same before incrementing. This will work only if it is sorted.
Time Complexity: O(kn)
Space Complexity: O(n)
Here, n is longest array length, and k is the number of arrays.
JavaScript Functional Array Methods
Some parts of JavaScript can be written just like a functional programming language. Unlike imperative programming, JavaScript does not focus on the state of the program. It does not use loops, only function (method) calls. You can learn more about functional programming in JavaScript from Beginning Functional JavaScript by Anto Aravinth (Apress, 2017).
In this section, only three functional array methods in JavaScript will be explored: map, filter, and reduce. These methods do not change the original array contents.
Map
The map function applies passed function transformation to every element in the array and returns a new array with those transformations applied.
Filter
The filter function returns only those elements of the array that meet a passed condition parameter. Again, this does not change the original array.
Reduce
The reduce function combines all the elements in the array into one value using a passed transformation function parameter.
Multidimensional Arrays

Multidimensional array

Jagged array

Three-by-three matrix

Three-by-three matrix of numbers
Exercises
All the code for the exercises can be found on GitHub.2
Spiral Print

Spiral print
Printing from left to right
Printing from top to bottom
Printing from right to left
Printing from bottom to top
Keeping a limit on these four operations
Top row
Bottom row
Left column
Right column
Time Complexity: O(mn)
Space Complexity: O(1)
Here, m is the number of rows, and n is the number of columns . Each item in the matrix is visited only once.
Tic-Tac-Toe Check
Given a matrix representing a tic-tac-toe board , determine whether someone won, whether it was a tie, or whether the game has not ended yet.3
Here are some examples.
Here it is as a matrix: [['O', 'X', '-'], ['-' ,'X', 'O'], ['O', 'X', '-']].
Here it is as a matrix: [['O','-','X'], ['-','O','-'], ['-','X','O']].
Path Finding

Finding a path
This will generate the proper matrix where each row is an array of the characters and the board is the array of those rows.

Console output
Time Complexity: O(mn)
Space Complexity: O(1)
Here, m is the row length , and n is the column length. Each element is visited only once.
Matrix Rotation
Rotate a matrix to the left by 90 degrees .
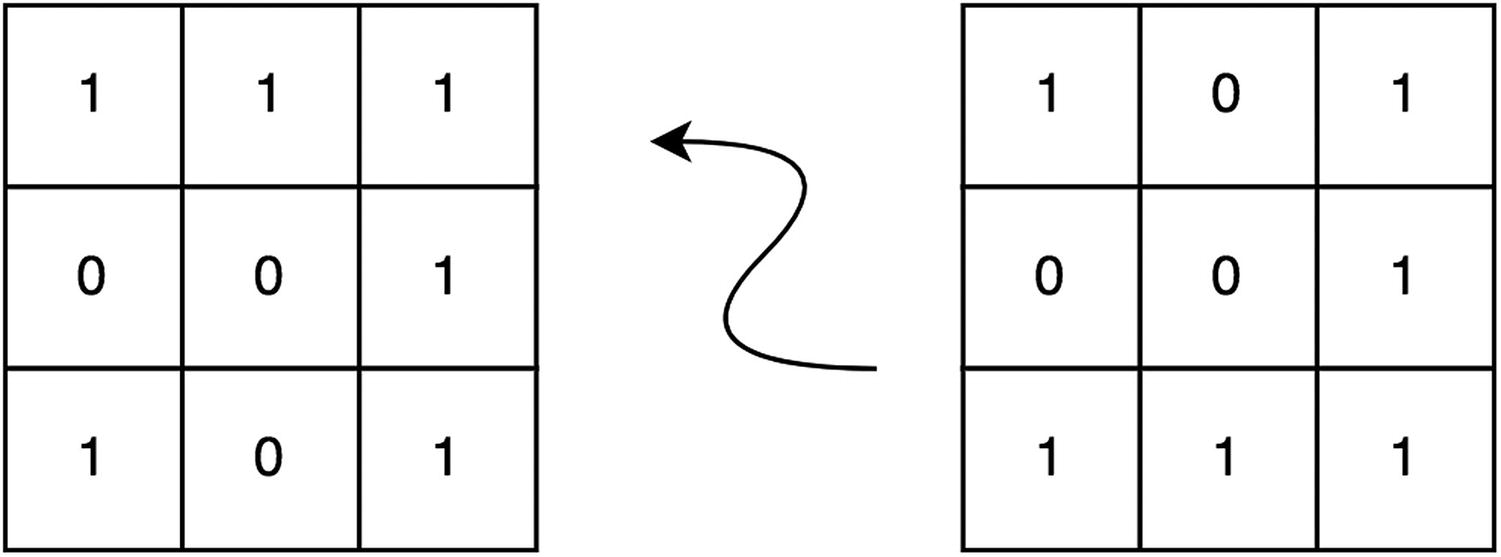
Matrix counterclockwise rotation
- 1.
The third column of the matrix becomes the first row of the result.
- 2.
The second column of the matrix becomes the second row of the result.
- 3.
The first column of the matrix becomes the third row of the result.
Time Complexity: O(mn)
Space Complexity: O(1)
Here, m is the row length, and n is the column length . Each element is visited only once. The space complexity is O(1) because the original array is modified instead of creating a new array.
Summary
Array Function Summary
Function | Usage |
---|---|
push(element) | Adds an element to the end of the array |
pop() | Removes the last element of the array |
shift() | Removes the first element of the array |
slice(beginIndex, endIndex) | Returns a part of the array from beginIndex to endIndex |
splice(beginIndex, endIndex) | Returns a part of the array from beginIndex to endIndex and modifies the original array by removing those elements |
concat(arr) | Adds new elements (from arr) into the array at the end of array |
Iteration Summary
Function | Usage |
---|---|
for (var prop in arr) | Iterates by the index of the array element |
for (var elem of arr) | Iterates by the value of the array element |
arr.forEach(fnc) | Applies the fnc value on each element |
Finally, recall that JavaScript utilizes jagged arrays, an array of arrays, to get multidimensional array behavior. With two-dimensional arrays, two-dimensional surfaces such as a tic-tac-toe board and maze can easily be represented.